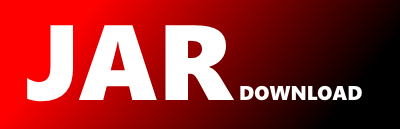
com.apollographql.apollo.cache.normalized.ApolloStoreOperation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apollo-normalized-cache Show documentation
Show all versions of apollo-normalized-cache Show documentation
Apollo GraphQL Normalized Cache APIs
package com.apollographql.apollo.cache.normalized
import com.apollographql.apollo.exception.ApolloException
import java.util.concurrent.Executor
import java.util.concurrent.atomic.AtomicBoolean
import java.util.concurrent.atomic.AtomicReference
/**
* Apollo store operation to be performed.
*
*
* This class is a wrapper around operation to be performed on [ApolloStore]. Due to the fact that any operation
* can potentially include SQLite instruction, any operation on [ApolloStore] must be performed in background
* thread. Use [.enqueue] to schedule such operation in the dispatcher with a callback to get results.
*
*
* @param result type for this operation
*/
abstract class ApolloStoreOperation protected constructor(private val dispatcher: Executor) {
private val callback = AtomicReference?>()
private val executed = AtomicBoolean()
protected abstract fun perform(): T
/**
* Execute store operation
*
*
* **NOTE: this is a sync operation, proceed with a caution as it may include SQLite instruction****
** *
*
* @throws [ApolloException] in case of any errors
*/
@Throws(ApolloException::class)
fun execute(): T {
checkIfExecuted()
return try {
perform()
} catch (e: Exception) {
throw ApolloException("Failed to perform store operation", e)
}
}
/**
* Schedules operation to be executed in dispatcher
*
* @param callback to be notified about operation result
*/
open fun enqueue(callback: Callback?) {
checkIfExecuted()
this.callback.set(callback)
dispatcher.execute(Runnable {
val result: T
result = try {
perform()
} catch (e: Exception) {
notifyFailure(ApolloException("Failed to perform store operation", e))
return@Runnable
}
notifySuccess(result)
})
}
fun notifySuccess(result: T) {
val callback = callback.getAndSet(null) ?: return
callback.onSuccess(result)
}
fun notifyFailure(t: Throwable) {
val callback = callback.getAndSet(null) ?: return
callback.onFailure(t)
}
private fun checkIfExecuted() {
check(executed.compareAndSet(false, true)) { "Already Executed" }
}
/**
* Operation result callback
*
* @param result type
*/
interface Callback {
fun onSuccess(result: T)
fun onFailure(t: Throwable)
}
companion object {
@JvmStatic
fun emptyOperation(result: T): ApolloStoreOperation {
return object : ApolloStoreOperation(emptyExecutor()) {
override fun perform(): T {
return result
}
override fun enqueue(callback: Callback?) {
callback?.onSuccess(result)
}
}
}
@JvmStatic
fun emptyExecutor() = Executor { }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy