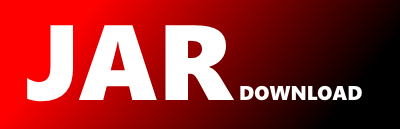
com.apollographql.apollo.rx2.RxJavaExtensions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apollo-rx2-support Show documentation
Show all versions of apollo-rx2-support Show documentation
Apollo GraphQL RxJava2 bindings
@file:Suppress("NOTHING_TO_INLINE")
@file:JvmName("KotlinExtensions")
package com.apollographql.apollo.rx2
import com.apollographql.apollo.ApolloCall
import com.apollographql.apollo.ApolloClient
import com.apollographql.apollo.ApolloMutationCall
import com.apollographql.apollo.ApolloPrefetch
import com.apollographql.apollo.ApolloQueryCall
import com.apollographql.apollo.ApolloQueryWatcher
import com.apollographql.apollo.ApolloSubscriptionCall
import com.apollographql.apollo.api.Mutation
import com.apollographql.apollo.api.Operation
import com.apollographql.apollo.api.Query
import com.apollographql.apollo.api.Response
import com.apollographql.apollo.api.Subscription
import com.apollographql.apollo.cache.normalized.ApolloStoreOperation
import io.reactivex.BackpressureStrategy
import io.reactivex.Completable
import io.reactivex.Flowable
import io.reactivex.Observable
import io.reactivex.Single
import io.reactivex.annotations.CheckReturnValue
@JvmSynthetic
@CheckReturnValue
inline fun ApolloPrefetch.rx(): Completable =
Rx2Apollo.from(this)
@JvmSynthetic
@CheckReturnValue
inline fun ApolloStoreOperation.rx(): Single =
Rx2Apollo.from(this)
@JvmSynthetic
@CheckReturnValue
inline fun ApolloQueryWatcher.rx(): Observable> =
Rx2Apollo.from(this)
@JvmSynthetic
@CheckReturnValue
inline fun ApolloCall.rx(): Observable> =
Rx2Apollo.from(this)
@JvmSynthetic
@CheckReturnValue
inline fun ApolloSubscriptionCall.rx(
backpressureStrategy: BackpressureStrategy = BackpressureStrategy.LATEST
): Flowable> = Rx2Apollo.from(this, backpressureStrategy)
/**
* Creates a new [ApolloQueryCall] call and then converts it to an [Observable].
*
* The number of emissions this Observable will have is based on the
* [com.apollographql.apollo.fetcher.ResponseFetcher] used with the call.
*/
@JvmSynthetic
@CheckReturnValue
inline fun ApolloClient.rxQuery(
query: Query,
configure: ApolloQueryCall.() -> ApolloQueryCall = { this }
): Observable> = query(query).configure().rx()
/**
* Creates a new [ApolloMutationCall] call and then converts it to a [Single].
*/
@JvmSynthetic
@CheckReturnValue
inline fun ApolloClient.rxMutate(
mutation: Mutation,
configure: ApolloMutationCall.() -> ApolloMutationCall = { this }
): Single> = mutate(mutation).configure().rx().singleOrError()
/**
* Creates a new [ApolloMutationCall] call and then converts it to a [Single].
*
* Provided optimistic updates will be stored in [com.apollographql.apollo.cache.normalized.ApolloStore]
* immediately before mutation execution. Any [ApolloQueryWatcher] dependent on the changed cache records will
* be re-fetched.
*/
@JvmSynthetic
@CheckReturnValue
inline fun ApolloClient.rxMutate(
mutation: Mutation,
withOptimisticUpdates: D,
configure: ApolloMutationCall.() -> ApolloMutationCall = { this }
): Single> = mutate(mutation, withOptimisticUpdates).configure().rx().singleOrError()
/**
* Creates the [ApolloPrefetch] by wrapping the operation object inside and then converts it to a [Completable].
*/
@JvmSynthetic
@CheckReturnValue
inline fun ApolloClient.rxPrefetch(
operation: Operation
): Completable = prefetch(operation).rx()
/**
* Creates a new [ApolloSubscriptionCall] call and then converts it to a [Flowable].
*
* Back-pressure strategy can be provided via [backpressureStrategy] parameter. The default value is [BackpressureStrategy.LATEST]
*/
@JvmSynthetic
@CheckReturnValue
inline fun ApolloClient.rxSubscribe(
subscription: Subscription,
backpressureStrategy: BackpressureStrategy = BackpressureStrategy.LATEST
): Flowable> = subscribe(subscription).rx(backpressureStrategy)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy