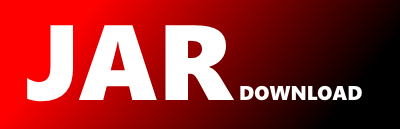
com.apollographql.apollo3.gradle.internal.SchemaDownloader.kt Maven / Gradle / Ivy
package com.apollographql.apollo3.gradle.internal
import com.apollographql.apollo3.compiler.fromJson
object SchemaDownloader {
fun downloadIntrospection(
endpoint: String,
headers: Map
): String {
val body = mapOf(
"query" to introspectionQuery,
"operationName" to "IntrospectionQuery"
)
val response = SchemaHelper.executeQuery(body, endpoint, headers)
return response.body.use { responseBody ->
responseBody!!.string()
}
}
fun downloadRegistry(
key: String,
graph: String,
variant: String,
endpoint: String = "https://graphql.api.apollographql.com/api/graphql"
): String {
val query = """
query DownloadSchema(${'$'}graphID: ID!, ${'$'}variant: String!) {
service(id: ${'$'}graphID) {
variant(name: ${'$'}variant) {
activeSchemaPublish {
schema {
document
}
}
}
}
}
""".trimIndent()
val variables = mapOf("graphID" to graph, "variant" to variant)
val response = SchemaHelper.executeQuery(query, variables, endpoint, mapOf("x-api-key" to key))
val responseString = response.body.use { it?.string() }
val document = responseString
?.fromJson
© 2015 - 2025 Weber Informatics LLC | Privacy Policy