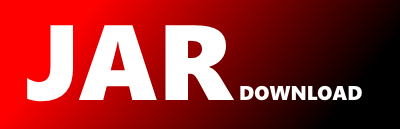
com.app55.message.Message Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of app55-java Show documentation
Show all versions of app55-java Show documentation
App55 client library for the Java platform.
The newest version!
package com.app55.message;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.net.URLEncoder;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.TreeMap;
import org.codehaus.jackson.annotate.JsonIgnore;
import org.codehaus.jackson.annotate.JsonProperty;
import com.app55.Gateway;
import com.app55.converter.Converter;
import com.app55.util.EncodeUtil;
import com.app55.util.ReflectionUtil;
import com.googlecode.openbeans.PropertyDescriptor;
public abstract class Message
{
protected Map additionalFields = new HashMap();
private Gateway gateway;
@JsonIgnore
public Gateway getGateway()
{
return gateway;
}
public void setGateway(Gateway gateway)
{
this.gateway = gateway;
}
@JsonProperty(value = "sig")
public abstract String getSignature();
@JsonProperty(value = "ts")
public abstract String getTimestamp();
@JsonIgnore
public String getFormData()
{
return toFormData(true, null);
}
protected String toSignature()
{
return toSignature(false);
}
protected String toSignature(boolean includeApiKey)
{
Map exclude = new HashMap();
exclude.put("sig", true);
String formData = toFormData(includeApiKey, exclude);
byte[] digest = EncodeUtil.sha1(gateway.getApiSecret() + formData);
return EncodeUtil.base64(digest);
}
public String toFormData(boolean includeApiKey, Map exclude)
{
try
{
String formData = "";
Map description = describe(this, null, exclude);
if (includeApiKey)
{
description.put("api_key", gateway.getApiKey());
if (exclude == null || !exclude.containsKey("sig"))
{
description.remove("sig");
description.put("sig", toSignature(true));
}
}
for (Map.Entry entry : additionalFields.entrySet())
description.put(entry.getKey(), entry.getValue());
for (Map.Entry entry : description.entrySet())
formData += "&" + entry.getKey() + "=" + URLEncoder.encode(entry.getValue(), "UTF-8");
if (formData.length() > 0)
formData = formData.substring(1);
formData = formData.replace("+", "%20");
formData = formData.replace("+", "%20");
return formData;
}
catch (UnsupportedEncodingException e)
{
// This will never happen. UTF-8 is supported.
return null;
}
}
@SuppressWarnings("unused")
private Map describe(Object o)
{
return describe(o, null, null);
}
private Map describe(Object o, String prefix)
{
return describe(o, prefix, null);
}
private Map describe(Object o, String prefix, Map exclude)
{
if (o == null)
return new HashMap();
prefix = prefix == null ? "" : prefix + ".";
Map description = new TreeMap();
Map properties = ReflectionUtil.getAllProperties(o);
for (Entry property : properties.entrySet())
{
Method m = property.getValue().getReadMethod();
if (exclude != null && exclude.containsKey(property.getKey()))
continue;
Object value = null;
try
{
value = m.invoke(o);
}
catch (InvocationTargetException e)
{
// If the invocation results in an exception, this wraps it. So if anything goes wrong, skip this method.
}
catch (IllegalAccessException e)
{
// No access to method means it shouldn't be invoked.
}
if (value == null)
continue;
if (Converter.getInstance().canConvert(value))
{
description.put(prefix + property.getKey(), Converter.getInstance().convert(value));
}
else if (value instanceof Collection)
{
int i = 0;
for (Object obj : (Collection>) value)
{
for (Entry entry : describe(obj, prefix + property.getKey() + '.' + i).entrySet())
description.put(entry.getKey(), entry.getValue());
i++;
}
}
else
{
for (Entry entry : describe(value, prefix + property.getKey()).entrySet())
description.put(entry.getKey(), entry.getValue());
}
}
return description;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy