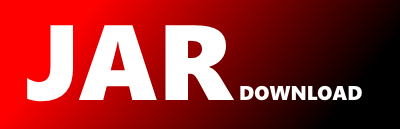
com.app55.message.Response Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of app55-java Show documentation
Show all versions of app55-java Show documentation
App55 client library for the Java platform.
The newest version!
package com.app55.message;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.SortedMap;
import java.util.TreeMap;
import org.codehaus.jackson.annotate.JsonIgnore;
import org.codehaus.jackson.annotate.JsonProperty;
import com.app55.Gateway;
import com.app55.util.ReflectionUtil;
import com.googlecode.openbeans.PropertyDescriptor;
public class Response extends Message
{
private String signature;
private String timestamp;
protected Response()
{
}
protected Response(Gateway gateway, Map ht)
{
setGateway(gateway);
this.populate(ht);
}
@Override
@JsonProperty(value = "sig")
public String getSignature()
{
return signature;
}
public void setSignature(String signature)
{
this.signature = signature;
}
@Override
@JsonProperty(value = "ts")
public String getTimestamp()
{
return timestamp;
}
public void setTimestamp(String timestamp)
{
this.timestamp = timestamp;
}
@JsonIgnore
public boolean isValidSignature()
{
String expectedSig = getExpectedSignature();
return expectedSig.equals(signature);
}
@JsonIgnore
public String getExpectedSignature()
{
return toSignature();
}
@SuppressWarnings("unchecked")
private void populateAdditional(Map hashtable, String prefix)
{
SortedMap ht = new TreeMap(hashtable);
for (Entry entry : ht.entrySet())
{
String key = entry.getKey();
Object value = entry.getValue();
if (value != null)
{
if (value instanceof Map)
{
populateAdditional((Map) value, prefix + key + ".");
}
else if (value instanceof List)
{
int i = 0;
for (Object arrayItem : ((List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy