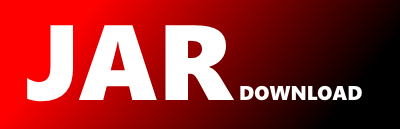
com.googlecode.openbeans.Encoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of app55-java Show documentation
Show all versions of app55-java Show documentation
App55 client library for the Java platform.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.openbeans;
import java.awt.Choice;
import java.awt.Color;
import java.awt.Component;
import java.awt.Container;
import java.awt.Cursor;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Insets;
import java.awt.List;
import java.awt.Menu;
import java.awt.MenuBar;
import java.awt.MenuShortcut;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.ScrollPane;
import java.awt.SystemColor;
import java.awt.font.TextAttribute;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.util.Date;
import java.util.Hashtable;
import java.util.IdentityHashMap;
import javax.swing.Box;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JFrame;
import javax.swing.JTabbedPane;
import javax.swing.ToolTipManager;
/**
* The Encoder
, together with PersistenceDelegate
s, can encode an object into a series of java statements. By executing these
* statements, a new object can be created and it will has the same state as the original object which has been passed to the encoder. Here "has the same state"
* means the two objects are indistinguishable from their public API.
*
* The Encoder
and PersistenceDelegate
s do this by creating copies of the input object and all objects it references. The copy
* process continues recursively util every object in the object graph has its new copy and the new version has the same state as the old version. All
* statements used to create those new objects and executed on them during the process form the result of encoding.
*
*
*/
public class Encoder
{
private static final Hashtable, PersistenceDelegate> delegates = new Hashtable, PersistenceDelegate>();
private static final DefaultPersistenceDelegate defaultPD = new DefaultPersistenceDelegate();
private static final UtilCollectionsPersistenceDelegate utilCollectionsPD = new UtilCollectionsPersistenceDelegate();
private static final ArrayPersistenceDelegate arrayPD = new ArrayPersistenceDelegate();
private static final ProxyPersistenceDelegate proxyPD = new ProxyPersistenceDelegate();
private static final NullPersistenceDelegate nullPD = new NullPersistenceDelegate();
private static final ExceptionListener defaultExListener = new DefaultExceptionListener();
private static class DefaultExceptionListener implements ExceptionListener
{
public void exceptionThrown(Exception exception)
{
System.err.println("Exception during encoding:" + exception); //$NON-NLS-1$
System.err.println("Continue..."); //$NON-NLS-1$
}
}
static
{
PersistenceDelegate ppd = new PrimitiveWrapperPersistenceDelegate();
delegates.put(Boolean.class, ppd);
delegates.put(Byte.class, ppd);
delegates.put(Character.class, ppd);
delegates.put(Double.class, ppd);
delegates.put(Float.class, ppd);
delegates.put(Integer.class, ppd);
delegates.put(Long.class, ppd);
delegates.put(Short.class, ppd);
delegates.put(Class.class, new ClassPersistenceDelegate());
delegates.put(Field.class, new FieldPersistenceDelegate());
delegates.put(Method.class, new MethodPersistenceDelegate());
delegates.put(String.class, new StringPersistenceDelegate());
delegates.put(Proxy.class, new ProxyPersistenceDelegate());
delegates.put(Choice.class, new AwtChoicePersistenceDelegate());
delegates.put(Color.class, new AwtColorPersistenceDelegate());
delegates.put(Container.class, new AwtContainerPersistenceDelegate());
delegates.put(Component.class, new AwtComponentPersistenceDelegate());
delegates.put(Cursor.class, new AwtCursorPersistenceDelegate());
delegates.put(Dimension.class, new AwtDimensionPersistenceDelegate());
delegates.put(Font.class, new AwtFontPersistenceDelegate());
delegates.put(Insets.class, new AwtInsetsPersistenceDelegate());
delegates.put(List.class, new AwtListPersistenceDelegate());
delegates.put(Menu.class, new AwtMenuPersistenceDelegate());
delegates.put(MenuBar.class, new AwtMenuBarPersistenceDelegate());
delegates.put(MenuShortcut.class, new AwtMenuShortcutPersistenceDelegate());
delegates.put(Point.class, new AwtPointPersistenceDelegate());
delegates.put(Rectangle.class, new AwtRectanglePersistenceDelegate());
delegates.put(SystemColor.class, new AwtSystemColorPersistenceDelegate());
delegates.put(TextAttribute.class, new AwtFontTextAttributePersistenceDelegate());
delegates.put(Box.class, new SwingBoxPersistenceDelegate());
delegates.put(JFrame.class, new SwingJFramePersistenceDelegate());
delegates.put(JTabbedPane.class, new SwingJTabbedPanePersistenceDelegate());
delegates.put(DefaultComboBoxModel.class, new SwingDefaultComboBoxModelPersistenceDelegate());
delegates.put(ToolTipManager.class, new SwingToolTipManagerPersistenceDelegate());
delegates.put(ScrollPane.class, new AwtScrollPanePersistenceDelegate());
delegates.put(Date.class, new UtilDatePersistenceDelegate());
PersistenceDelegate pd = new UtilListPersistenceDelegate();
delegates.put(java.util.List.class, pd);
delegates.put(java.util.AbstractList.class, pd);
pd = new UtilCollectionPersistenceDelegate();
delegates.put(java.util.Collection.class, pd);
delegates.put(java.util.AbstractCollection.class, pd);
pd = new UtilMapPersistenceDelegate();
delegates.put(java.util.Map.class, pd);
delegates.put(java.util.AbstractMap.class, pd);
delegates.put(java.util.Hashtable.class, pd);
}
private ExceptionListener listener = defaultExListener;
private IdentityHashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy