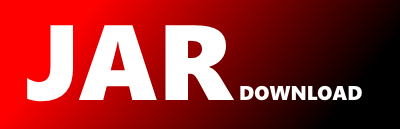
com.googlecode.openbeans.XMLDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of app55-java Show documentation
Show all versions of app55-java Show documentation
App55 client library for the Java platform.
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.openbeans;
import java.io.InputStream;
import java.lang.reflect.Array;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Stack;
import javax.xml.parsers.SAXParserFactory;
import org.apache.harmony.beans.internal.nls.Messages;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import org.xml.sax.helpers.DefaultHandler;
import com.googlecode.openbeans.Statement.MethodComparator;
/**
* XMLDecoder
reads objects from xml created by XMLEncoder
.
*
* The API is similar to ObjectInputStream
.
*
*/
public class XMLDecoder
{
private ClassLoader defaultClassLoader = null;
private static class DefaultExceptionListener implements ExceptionListener
{
public void exceptionThrown(Exception e)
{
System.err.println(e.getMessage());
System.err.println("Continue..."); //$NON-NLS-1$
}
}
private class SAXHandler extends DefaultHandler
{
boolean inJavaElem = false;
HashMap idObjMap = new HashMap();
@Override
public void characters(char[] ch, int start, int length) throws SAXException
{
if (!inJavaElem)
{
return;
}
if (readObjs.size() > 0)
{
Elem elem = readObjs.peek();
if (elem.isBasicType)
{
String str = new String(ch, start, length);
elem.methodName = elem.methodName == null ? str : elem.methodName + str;
}
}
}
@SuppressWarnings("nls")
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException
{
if (!inJavaElem)
{
if ("java".equals(qName))
{
inJavaElem = true;
}
else
{
listener.exceptionThrown(new Exception(Messages.getString("beans.72", qName)));
}
return;
}
if ("object".equals(qName))
{
startObjectElem(attributes);
}
else if ("array".equals(qName))
{
startArrayElem(attributes);
}
else if ("void".equals(qName))
{
startVoidElem(attributes);
}
else if ("boolean".equals(qName) || "byte".equals(qName) || "char".equals(qName) || "class".equals(qName) || "double".equals(qName)
|| "float".equals(qName) || "int".equals(qName) || "long".equals(qName) || "short".equals(qName) || "string".equals(qName)
|| "null".equals(qName))
{
startBasicElem(qName, attributes);
}
}
@SuppressWarnings("nls")
private void startObjectElem(Attributes attributes)
{
Elem elem = new Elem();
elem.isExpression = true;
elem.id = attributes.getValue("id");
elem.idref = attributes.getValue("idref");
elem.attributes = attributes;
if (elem.idref == null)
{
obtainTarget(elem, attributes);
obtainMethod(elem, attributes);
}
readObjs.push(elem);
}
private void obtainTarget(Elem elem, Attributes attributes)
{
String className = attributes.getValue("class"); //$NON-NLS-1$
if (className != null)
{
try
{
elem.target = classForName(className);
}
catch (ClassNotFoundException e)
{
listener.exceptionThrown(e);
}
}
else
{
Elem parent = latestUnclosedElem();
if (parent == null)
{
elem.target = owner;
return;
}
elem.target = execute(parent);
}
}
@SuppressWarnings("nls")
private void obtainMethod(Elem elem, Attributes attributes)
{
elem.methodName = attributes.getValue("method");
if (elem.methodName != null)
{
return;
}
elem.methodName = attributes.getValue("property");
if (elem.methodName != null)
{
elem.fromProperty = true;
return;
}
elem.methodName = attributes.getValue("index");
if (elem.methodName != null)
{
elem.fromIndex = true;
return;
}
elem.methodName = attributes.getValue("field");
if (elem.methodName != null)
{
elem.fromField = true;
return;
}
elem.methodName = attributes.getValue("owner");
if (elem.methodName != null)
{
elem.fromOwner = true;
return;
}
elem.methodName = "new"; // default method name
}
@SuppressWarnings("nls")
private Class> classForName(String className) throws ClassNotFoundException
{
if ("boolean".equals(className))
{
return Boolean.TYPE;
}
else if ("byte".equals(className))
{
return Byte.TYPE;
}
else if ("char".equals(className))
{
return Character.TYPE;
}
else if ("double".equals(className))
{
return Double.TYPE;
}
else if ("float".equals(className))
{
return Float.TYPE;
}
else if ("int".equals(className))
{
return Integer.TYPE;
}
else if ("long".equals(className))
{
return Long.TYPE;
}
else if ("short".equals(className))
{
return Short.TYPE;
}
else
{
return Class.forName(className, true, defaultClassLoader == null ? Thread.currentThread().getContextClassLoader() : defaultClassLoader);
}
}
private void startArrayElem(Attributes attributes)
{
Elem elem = new Elem();
elem.isExpression = true;
elem.id = attributes.getValue("id"); //$NON-NLS-1$
elem.attributes = attributes;
try
{
// find component class
Class> compClass = classForName(attributes.getValue("class")); //$NON-NLS-1$
String lengthValue = attributes.getValue("length"); //$NON-NLS-1$
if (lengthValue != null)
{
// find length
int length = Integer.parseInt(attributes.getValue("length")); //$NON-NLS-1$
// execute, new array instance
elem.result = Array.newInstance(compClass, length);
elem.isExecuted = true;
}
else
{
// create array without length attribute,
// delay the excution to the end,
// get array length from sub element
elem.target = compClass;
elem.methodName = "newArray"; //$NON-NLS-1$
elem.isExecuted = false;
}
}
catch (Exception e)
{
listener.exceptionThrown(e);
}
readObjs.push(elem);
}
@SuppressWarnings("nls")
private void startVoidElem(Attributes attributes)
{
Elem elem = new Elem();
elem.id = attributes.getValue("id");
elem.attributes = attributes;
obtainTarget(elem, attributes);
obtainMethod(elem, attributes);
readObjs.push(elem);
}
@SuppressWarnings("nls")
private void startBasicElem(String tagName, Attributes attributes)
{
Elem elem = new Elem();
elem.isBasicType = true;
elem.isExpression = true;
elem.id = attributes.getValue("id");
elem.idref = attributes.getValue("idref");
elem.attributes = attributes;
elem.target = tagName;
readObjs.push(elem);
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException
{
if (!inJavaElem)
{
return;
}
if ("java".equals(qName)) { //$NON-NLS-1$
inJavaElem = false;
return;
}
// find the elem to close
Elem toClose = latestUnclosedElem();
if ("string".equals(toClose.target))
{
StringBuilder sb = new StringBuilder();
for (int index = readObjs.size() - 1; index >= 0; index--)
{
Elem elem = (Elem) readObjs.get(index);
if (toClose == elem)
{
break;
}
if ("char".equals(elem.target))
{
sb.insert(0, elem.methodName);
}
}
toClose.methodName = toClose.methodName != null ? toClose.methodName + sb.toString() : sb.toString();
}
// make sure it is executed
execute(toClose);
// set to closed
toClose.isClosed = true;
// pop it and its children
while (readObjs.pop() != toClose)
{
//
}
if (toClose.isExpression)
{
// push back expression
readObjs.push(toClose);
}
}
private Elem latestUnclosedElem()
{
for (int i = readObjs.size() - 1; i >= 0; i--)
{
Elem elem = readObjs.get(i);
if (!elem.isClosed)
{
return elem;
}
}
return null;
}
private Object execute(Elem elem)
{
if (elem.isExecuted)
{
return elem.result;
}
// execute to obtain result
try
{
if (elem.idref != null)
{
elem.result = idObjMap.get(elem.idref);
}
else if (elem.isBasicType)
{
elem.result = executeBasic(elem);
}
else
{
elem.result = executeCommon(elem);
}
}
catch (Exception e)
{
listener.exceptionThrown(e);
}
// track id
if (elem.id != null)
{
idObjMap.put(elem.id, elem.result);
}
elem.isExecuted = true;
return elem.result;
}
@SuppressWarnings("nls")
private Object executeCommon(Elem elem) throws Exception
{
// pop args
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy