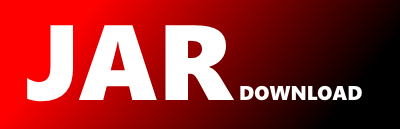
com.applitools.eyes.visualgridclient.model.RenderRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eyes-common-java3 Show documentation
Show all versions of eyes-common-java3 Show documentation
Common code for Applitools Eyes Java SDK projects
package com.applitools.eyes.visualgridclient.model;
import com.applitools.eyes.visualgridclient.services.VisualGridTask;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class RenderRequest {
@JsonInclude
private String renderId;
@JsonIgnore
private final VisualGridTask visualGridTask;
@JsonInclude(JsonInclude.Include.NON_NULL)
private String webhook;
@JsonInclude(JsonInclude.Include.NON_NULL)
private String url;
@JsonInclude(JsonInclude.Include.NON_NULL)
private RGridDom dom;
@JsonInclude(JsonInclude.Include.NON_NULL)
private Map resources;
@JsonInclude(JsonInclude.Include.NON_NULL)
private RenderInfo renderInfo;
@JsonIgnore
private String platform;
@JsonIgnore
private String browserName;
@JsonInclude(JsonInclude.Include.NON_NULL)
private Object scriptHooks;
@JsonInclude(JsonInclude.Include.NON_NULL)
private List selectorsToFindRegionsFor;
@JsonInclude(JsonInclude.Include.NON_NULL)
private boolean sendDom;
public RenderRequest(String webHook, String url, RGridDom dom, Map resources, RenderInfo renderInfo, String platform, String browserName, Object scriptHooks, List selectorsToFindRegionsFor, boolean sendDom, VisualGridTask visualGridTask) {
this.webhook = webHook;
this.url = url;
this.dom = dom;
this.resources = resources;
this.renderInfo = renderInfo;
this.platform = platform;
this.browserName = browserName;
this.scriptHooks = scriptHooks;
this.selectorsToFindRegionsFor = selectorsToFindRegionsFor;
this.sendDom = sendDom;
this.visualGridTask = visualGridTask;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public RGridDom getDom() {
return dom;
}
public void setDom(RGridDom dom) {
this.dom = dom;
}
public Map getResources() {
return resources;
}
public void setResources(Map resources) {
this.resources = resources;
}
public RenderInfo getRenderInfo() {
return renderInfo;
}
public void setRenderInfo(RenderInfo renderInfo) {
this.renderInfo = renderInfo;
}
public String getPlatform() {
return platform;
}
public void setPlatform(String platform) {
this.platform = platform;
}
public String getBrowserName() {
return browserName;
}
public void setBrowserName(String browserName) {
this.browserName = browserName;
}
public Object getScriptHooks() {
return scriptHooks;
}
public void setScriptHooks(Object scriptHooks) {
this.scriptHooks = scriptHooks;
}
public List getSelectorsToFindRegionsFor() {
return selectorsToFindRegionsFor;
}
public void setSelectorsToFindRegionsFor(List selectorsToFindRegionsFor) {
this.selectorsToFindRegionsFor = selectorsToFindRegionsFor;
}
public boolean isSendDom() {
return sendDom;
}
public void setSendDom(boolean sendDom) {
this.sendDom = sendDom;
}
@JsonProperty("browser")
@JsonInclude(JsonInclude.Include.NON_NULL)
public Map getBrowser(){
Map map = new HashMap<>();
map.put("name", this.browserName);
map.put("platform", this.platform);
return map;
}
public VisualGridTask getVisualGridTask() {
return visualGridTask;
}
public String getWebhook() {
return webhook;
}
public String getRenderId() {
return renderId;
}
public void setRenderId(String renderId) {
this.renderId = renderId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy