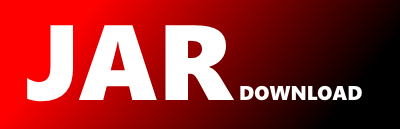
com.applitools.eyes.visualgridclient.services.RenderingGridService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eyes-common-java3 Show documentation
Show all versions of eyes-common-java3 Show documentation
Common code for Applitools Eyes Java SDK projects
package com.applitools.eyes.visualgridclient.services;
import com.applitools.eyes.Logger;
import com.applitools.eyes.visualgridclient.model.RenderingTask;
import com.applitools.utils.GeneralUtils;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
public class RenderingGridService extends Thread {
private static final int FACTOR = 5;
private final Object debugLock;
private final RGServiceListener listener;
private boolean isServiceOn = true;
private ExecutorService executor;
protected Logger logger;
private boolean isPaused;
public void setLogger(Logger logger) {
}
public interface RGServiceListener {
RenderingTask getNextTask();
}
RenderingGridService(String serviceName, ThreadGroup servicesGroup, Logger logger, int threadPoolSize, Object debugLock, RGServiceListener listener) {
super(servicesGroup, serviceName);
this.executor = new ThreadPoolExecutor(threadPoolSize, threadPoolSize * FACTOR, 1, TimeUnit.DAYS, new ArrayBlockingQueue(20));
this.debugLock = debugLock;
this.listener = listener;
this.logger = logger;
this.isPaused = debugLock != null;
}
@Override
public void run() {
try {
while (isServiceOn) {
if (isPaused) {
synchronized (debugLock) {
try {
debugLock.wait();
this.isPaused = false;
} catch (InterruptedException e) {
GeneralUtils.logExceptionStackTrace(logger, e);
}
}
}
runNextTask();
}
if (this.executor != null) {
this.executor.shutdown();
}
logger.verbose("Service '" + this.getName() + "' is finished");
} catch (Throwable e) {
logger.verbose("Rendering Service Error : "+e);
}
}
private void runNextTask() {
final RenderingTask task = this.listener.getNextTask();
if (task != null) {
task.addListener(new RenderingTask.RenderTaskListener() {
@Override
public void onRenderSuccess() {
debugNotify();
}
@Override
public void onRenderFailed(Exception e) {
debugNotify();
}
});
try {
this.executor.submit(task);
} catch (Exception e) {
logger.verbose("Exception in - this.executor.submit(task); ");
if(e.getMessage().contains("Read timed out")){
logger.verbose("Read timed out");
}
e.printStackTrace();
GeneralUtils.logExceptionStackTrace(logger, e);
}
}
}
private void debugNotify() {
if (debugLock != null) {
synchronized (debugLock) {
debugLock.notify();
}
}
}
public void debugPauseService() {
this.isPaused = true;
}
public void stopService() {
this.isServiceOn = false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy