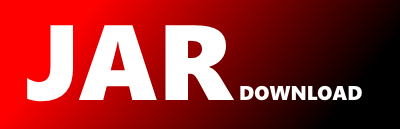
com.applitools.connectivity.api.AsyncRequestImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eyes-connectivity-java3-jersey2x Show documentation
Show all versions of eyes-connectivity-java3-jersey2x Show documentation
Applitools Eyes SDK REST API communication implementation depending on Jersey version 2x
package com.applitools.connectivity.api;
import com.applitools.eyes.Logger;
import com.applitools.utils.ArgumentGuard;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.Invocation;
import javax.ws.rs.client.InvocationCallback;
import javax.ws.rs.core.Response;
import java.util.concurrent.Future;
public class AsyncRequestImpl extends AsyncRequest {
Invocation.Builder request;
AsyncRequestImpl(Invocation.Builder request, Logger logger) {
super(logger);
this.request = request;
}
@Override
public AsyncRequest header(String name, String value) {
ArgumentGuard.notNullOrEmpty(name, "header name");
ArgumentGuard.notNullOrEmpty(value, String.format("value of %s", name));
request = request.header(name, value);
return this;
}
@Override
public Future> method(String method, final AsyncRequestCallback callback, Object data, String contentType, final boolean logIfError) {
ArgumentGuard.notNullOrEmpty(method, "method");
InvocationCallback invocationCallback = new InvocationCallback() {
@Override
public void completed(Response response) {
try {
callback.onComplete(new ResponseImpl(response, logIfError ? logger : new Logger()));
} catch (Exception e) {
callback.onFail(e);
}
}
@Override
public void failed(Throwable throwable) {
callback.onFail(throwable);
}
};
if (data == null || contentType == null) {
return request.async().method(method, invocationCallback);
}
return request.async().method(method, Entity.entity(data, contentType), invocationCallback);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy