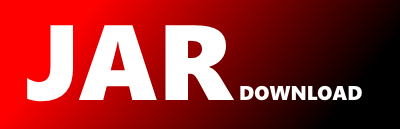
com.applitools.eyes.selenium.universal.mapper.TDynamicRegionMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eyes-selenium-java5 Show documentation
Show all versions of eyes-selenium-java5 Show documentation
Applitools Eyes SDK for Selenium Java WebDriver
package com.applitools.eyes.selenium.universal.mapper;
import com.applitools.eyes.DynamicRegionByRectangle;
import com.applitools.eyes.DynamicTextType;
import com.applitools.eyes.GetDynamicTextType;
import com.applitools.eyes.fluent.GetRegion;
import com.applitools.eyes.selenium.TargetPathLocator;
import com.applitools.eyes.selenium.fluent.DynamicRegionByElement;
import com.applitools.eyes.selenium.fluent.DynamicRegionBySelector;
import com.applitools.eyes.selenium.fluent.DynamicRegionByTargetPathLocator;
import com.applitools.eyes.selenium.universal.dto.ElementDynamicRegionDto;
import com.applitools.eyes.selenium.universal.dto.ElementRegionDto;
import com.applitools.eyes.selenium.universal.dto.TargetPathLocatorDynamicRegionDto;
import com.applitools.eyes.selenium.universal.dto.TargetPathLocatorDto;
import com.applitools.eyes.universal.dto.*;
import com.applitools.eyes.universal.mapper.RectangleRegionMapper;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
/**
* dynamic region mapper
*/
public class TDynamicRegionMapper {
public static TDynamicRegion toTDynamicRegionDto(GetRegion getDynamicRegion) {
if (getDynamicRegion == null) {
return null;
}
if (getDynamicRegion instanceof DynamicRegionByRectangle) {
RectangleDynamicRegionDto rectangleDynamicRegionDto = new RectangleDynamicRegionDto();
RectangleRegionDto rectangleRegionDto =
RectangleRegionMapper.toRectangleRegionDto(((DynamicRegionByRectangle) getDynamicRegion).getRegion());
rectangleDynamicRegionDto.setRegion(rectangleRegionDto);
if (((GetDynamicTextType) getDynamicRegion).getDynamicSettings() != null) {
rectangleDynamicRegionDto.setType(
Arrays.stream(((DynamicRegionByRectangle) getDynamicRegion).getDynamicSettings().getIgnorePatterns())
.map(DynamicTextType::getName).toArray(String[]::new));
}
return rectangleDynamicRegionDto;
} else if (getDynamicRegion instanceof DynamicRegionByElement) {
ElementDynamicRegionDto elementDynamicRegionDto = new ElementDynamicRegionDto();
WebElement element = ((DynamicRegionByElement) getDynamicRegion).getElement();
ElementRegionDto elementRegionDto = ElementRegionMapper.toElementRegionDto(element);
elementDynamicRegionDto.setRegion(elementRegionDto);
if (((GetDynamicTextType) getDynamicRegion).getDynamicSettings() != null) {
elementDynamicRegionDto.setType(
Arrays.stream(((DynamicRegionByElement) getDynamicRegion).getDynamicTextTypes())
.map(DynamicTextType::getName).toArray(String[]::new));
}
return elementDynamicRegionDto;
} else if (getDynamicRegion instanceof DynamicRegionBySelector) {
SelectorDynamicRegionDto selectorDynamicRegionDto = new SelectorDynamicRegionDto();
By by = ((DynamicRegionBySelector) getDynamicRegion).getSelector();
SelectorRegionDto selectorRegionDto = SelectorRegionMapper.toSelectorRegionDto(by);
selectorDynamicRegionDto.setRegion(selectorRegionDto);
if (((GetDynamicTextType) getDynamicRegion).getDynamicSettings() != null) {
selectorDynamicRegionDto.setType(
Arrays.stream(((DynamicRegionBySelector) getDynamicRegion).getDynamicTextTypes())
.map(DynamicTextType::getName).toArray(String[]::new));
}
return selectorDynamicRegionDto;
} else if (getDynamicRegion instanceof DynamicRegionByTargetPathLocator) {
TargetPathLocatorDynamicRegionDto targetPathLocatorDynamicRegionDto = new TargetPathLocatorDynamicRegionDto();
TargetPathLocator tpl = ((DynamicRegionByTargetPathLocator) getDynamicRegion).getTargetPathLocator();
TargetPathLocatorDto selectorRegionDto = TargetPathLocatorMapper.toTargetPathLocatorDto(tpl);
targetPathLocatorDynamicRegionDto.setRegion(selectorRegionDto);
if (((GetDynamicTextType) getDynamicRegion).getDynamicSettings() != null) {
targetPathLocatorDynamicRegionDto.setType(
Arrays.stream(((DynamicRegionByTargetPathLocator) getDynamicRegion).getDynamicTextTypes())
.map(DynamicTextType::getName).toArray(String[]::new));
}
return targetPathLocatorDynamicRegionDto;
}
return null;
}
public static List toTDynamicRegionDtoList(List getDynamicRegionList) {
if (getDynamicRegionList == null || getDynamicRegionList.isEmpty()) {
return null;
}
return getDynamicRegionList.stream().map(TDynamicRegionMapper::toTDynamicRegionDto).collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy