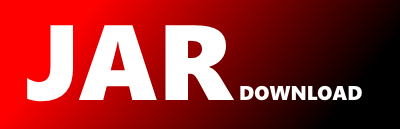
com.appscode.stash.client.models.V1alpha1ResticSpec Maven / Gradle / Ivy
The newest version!
/*
* Stash
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: v0.7.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.appscode.stash.client.models;
import java.util.Objects;
import com.appscode.stash.client.models.V1alpha1Backend;
import com.appscode.stash.client.models.V1alpha1FileGroup;
import com.appscode.stash.client.models.V1alpha1RetentionPolicy;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.kubernetes.client.models.V1LabelSelector;
import io.kubernetes.client.models.V1LocalObjectReference;
import io.kubernetes.client.models.V1ResourceRequirements;
import io.kubernetes.client.models.V1VolumeMount;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* V1alpha1ResticSpec
*/
public class V1alpha1ResticSpec {
@SerializedName("backend")
private V1alpha1Backend backend = null;
@SerializedName("fileGroups")
private List fileGroups = null;
@SerializedName("imagePullSecrets")
private List imagePullSecrets = null;
@SerializedName("paused")
private Boolean paused = null;
@SerializedName("resources")
private V1ResourceRequirements resources = null;
@SerializedName("retentionPolicies")
private List retentionPolicies = null;
@SerializedName("schedule")
private String schedule = null;
@SerializedName("selector")
private V1LabelSelector selector = null;
@SerializedName("type")
private String type = null;
@SerializedName("volumeMounts")
private List volumeMounts = null;
public V1alpha1ResticSpec backend(V1alpha1Backend backend) {
this.backend = backend;
return this;
}
/**
* Get backend
* @return backend
**/
@ApiModelProperty(value = "")
public V1alpha1Backend getBackend() {
return backend;
}
public void setBackend(V1alpha1Backend backend) {
this.backend = backend;
}
public V1alpha1ResticSpec fileGroups(List fileGroups) {
this.fileGroups = fileGroups;
return this;
}
public V1alpha1ResticSpec addFileGroupsItem(V1alpha1FileGroup fileGroupsItem) {
if (this.fileGroups == null) {
this.fileGroups = new ArrayList();
}
this.fileGroups.add(fileGroupsItem);
return this;
}
/**
* Get fileGroups
* @return fileGroups
**/
@ApiModelProperty(value = "")
public List getFileGroups() {
return fileGroups;
}
public void setFileGroups(List fileGroups) {
this.fileGroups = fileGroups;
}
public V1alpha1ResticSpec imagePullSecrets(List imagePullSecrets) {
this.imagePullSecrets = imagePullSecrets;
return this;
}
public V1alpha1ResticSpec addImagePullSecretsItem(V1LocalObjectReference imagePullSecretsItem) {
if (this.imagePullSecrets == null) {
this.imagePullSecrets = new ArrayList();
}
this.imagePullSecrets.add(imagePullSecretsItem);
return this;
}
/**
* ImagePullSecrets is an optional list of references to secrets in the same namespace to use for pulling any of the images used by this PodSpec. If specified, these secrets will be passed to individual puller implementations for them to use. For example, in the case of docker, only DockerConfig type secrets are honored. More info: https://kubernetes.io/docs/concepts/containers/images#specifying-imagepullsecrets-on-a-pod
* @return imagePullSecrets
**/
@ApiModelProperty(value = "ImagePullSecrets is an optional list of references to secrets in the same namespace to use for pulling any of the images used by this PodSpec. If specified, these secrets will be passed to individual puller implementations for them to use. For example, in the case of docker, only DockerConfig type secrets are honored. More info: https://kubernetes.io/docs/concepts/containers/images#specifying-imagepullsecrets-on-a-pod")
public List getImagePullSecrets() {
return imagePullSecrets;
}
public void setImagePullSecrets(List imagePullSecrets) {
this.imagePullSecrets = imagePullSecrets;
}
public V1alpha1ResticSpec paused(Boolean paused) {
this.paused = paused;
return this;
}
/**
* Indicates that the Restic is paused from taking backup. Default value is 'false'
* @return paused
**/
@ApiModelProperty(value = "Indicates that the Restic is paused from taking backup. Default value is 'false'")
public Boolean isPaused() {
return paused;
}
public void setPaused(Boolean paused) {
this.paused = paused;
}
public V1alpha1ResticSpec resources(V1ResourceRequirements resources) {
this.resources = resources;
return this;
}
/**
* Compute Resources required by the sidecar container.
* @return resources
**/
@ApiModelProperty(value = "Compute Resources required by the sidecar container.")
public V1ResourceRequirements getResources() {
return resources;
}
public void setResources(V1ResourceRequirements resources) {
this.resources = resources;
}
public V1alpha1ResticSpec retentionPolicies(List retentionPolicies) {
this.retentionPolicies = retentionPolicies;
return this;
}
public V1alpha1ResticSpec addRetentionPoliciesItem(V1alpha1RetentionPolicy retentionPoliciesItem) {
if (this.retentionPolicies == null) {
this.retentionPolicies = new ArrayList();
}
this.retentionPolicies.add(retentionPoliciesItem);
return this;
}
/**
* Get retentionPolicies
* @return retentionPolicies
**/
@ApiModelProperty(value = "")
public List getRetentionPolicies() {
return retentionPolicies;
}
public void setRetentionPolicies(List retentionPolicies) {
this.retentionPolicies = retentionPolicies;
}
public V1alpha1ResticSpec schedule(String schedule) {
this.schedule = schedule;
return this;
}
/**
* Get schedule
* @return schedule
**/
@ApiModelProperty(value = "")
public String getSchedule() {
return schedule;
}
public void setSchedule(String schedule) {
this.schedule = schedule;
}
public V1alpha1ResticSpec selector(V1LabelSelector selector) {
this.selector = selector;
return this;
}
/**
* Get selector
* @return selector
**/
@ApiModelProperty(value = "")
public V1LabelSelector getSelector() {
return selector;
}
public void setSelector(V1LabelSelector selector) {
this.selector = selector;
}
public V1alpha1ResticSpec type(String type) {
this.type = type;
return this;
}
/**
* https://github.com/appscode/stash/issues/225
* @return type
**/
@ApiModelProperty(value = "https://github.com/appscode/stash/issues/225")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public V1alpha1ResticSpec volumeMounts(List volumeMounts) {
this.volumeMounts = volumeMounts;
return this;
}
public V1alpha1ResticSpec addVolumeMountsItem(V1VolumeMount volumeMountsItem) {
if (this.volumeMounts == null) {
this.volumeMounts = new ArrayList();
}
this.volumeMounts.add(volumeMountsItem);
return this;
}
/**
* Pod volumes to mount into the sidecar container's filesystem.
* @return volumeMounts
**/
@ApiModelProperty(value = "Pod volumes to mount into the sidecar container's filesystem.")
public List getVolumeMounts() {
return volumeMounts;
}
public void setVolumeMounts(List volumeMounts) {
this.volumeMounts = volumeMounts;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
V1alpha1ResticSpec v1alpha1ResticSpec = (V1alpha1ResticSpec) o;
return Objects.equals(this.backend, v1alpha1ResticSpec.backend) &&
Objects.equals(this.fileGroups, v1alpha1ResticSpec.fileGroups) &&
Objects.equals(this.imagePullSecrets, v1alpha1ResticSpec.imagePullSecrets) &&
Objects.equals(this.paused, v1alpha1ResticSpec.paused) &&
Objects.equals(this.resources, v1alpha1ResticSpec.resources) &&
Objects.equals(this.retentionPolicies, v1alpha1ResticSpec.retentionPolicies) &&
Objects.equals(this.schedule, v1alpha1ResticSpec.schedule) &&
Objects.equals(this.selector, v1alpha1ResticSpec.selector) &&
Objects.equals(this.type, v1alpha1ResticSpec.type) &&
Objects.equals(this.volumeMounts, v1alpha1ResticSpec.volumeMounts);
}
@Override
public int hashCode() {
return Objects.hash(backend, fileGroups, imagePullSecrets, paused, resources, retentionPolicies, schedule, selector, type, volumeMounts);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V1alpha1ResticSpec {\n");
sb.append(" backend: ").append(toIndentedString(backend)).append("\n");
sb.append(" fileGroups: ").append(toIndentedString(fileGroups)).append("\n");
sb.append(" imagePullSecrets: ").append(toIndentedString(imagePullSecrets)).append("\n");
sb.append(" paused: ").append(toIndentedString(paused)).append("\n");
sb.append(" resources: ").append(toIndentedString(resources)).append("\n");
sb.append(" retentionPolicies: ").append(toIndentedString(retentionPolicies)).append("\n");
sb.append(" schedule: ").append(toIndentedString(schedule)).append("\n");
sb.append(" selector: ").append(toIndentedString(selector)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" volumeMounts: ").append(toIndentedString(volumeMounts)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy