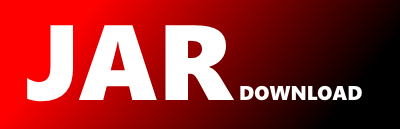
com.appscode.stash.client.models.V1alpha1SnapshotStatus Maven / Gradle / Ivy
The newest version!
/*
* Stash
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: v0.7.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.appscode.stash.client.models;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* V1alpha1SnapshotStatus
*/
public class V1alpha1SnapshotStatus {
@SerializedName("gid")
private Integer gid = null;
@SerializedName("hostname")
private String hostname = null;
@SerializedName("paths")
private List paths = new ArrayList();
@SerializedName("tree")
private String tree = null;
@SerializedName("uid")
private Integer uid = null;
@SerializedName("username")
private String username = null;
public V1alpha1SnapshotStatus gid(Integer gid) {
this.gid = gid;
return this;
}
/**
* Get gid
* @return gid
**/
@ApiModelProperty(required = true, value = "")
public Integer getGid() {
return gid;
}
public void setGid(Integer gid) {
this.gid = gid;
}
public V1alpha1SnapshotStatus hostname(String hostname) {
this.hostname = hostname;
return this;
}
/**
* Get hostname
* @return hostname
**/
@ApiModelProperty(required = true, value = "")
public String getHostname() {
return hostname;
}
public void setHostname(String hostname) {
this.hostname = hostname;
}
public V1alpha1SnapshotStatus paths(List paths) {
this.paths = paths;
return this;
}
public V1alpha1SnapshotStatus addPathsItem(String pathsItem) {
this.paths.add(pathsItem);
return this;
}
/**
* Get paths
* @return paths
**/
@ApiModelProperty(required = true, value = "")
public List getPaths() {
return paths;
}
public void setPaths(List paths) {
this.paths = paths;
}
public V1alpha1SnapshotStatus tree(String tree) {
this.tree = tree;
return this;
}
/**
* Get tree
* @return tree
**/
@ApiModelProperty(required = true, value = "")
public String getTree() {
return tree;
}
public void setTree(String tree) {
this.tree = tree;
}
public V1alpha1SnapshotStatus uid(Integer uid) {
this.uid = uid;
return this;
}
/**
* Get uid
* @return uid
**/
@ApiModelProperty(required = true, value = "")
public Integer getUid() {
return uid;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public V1alpha1SnapshotStatus username(String username) {
this.username = username;
return this;
}
/**
* Get username
* @return username
**/
@ApiModelProperty(required = true, value = "")
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
V1alpha1SnapshotStatus v1alpha1SnapshotStatus = (V1alpha1SnapshotStatus) o;
return Objects.equals(this.gid, v1alpha1SnapshotStatus.gid) &&
Objects.equals(this.hostname, v1alpha1SnapshotStatus.hostname) &&
Objects.equals(this.paths, v1alpha1SnapshotStatus.paths) &&
Objects.equals(this.tree, v1alpha1SnapshotStatus.tree) &&
Objects.equals(this.uid, v1alpha1SnapshotStatus.uid) &&
Objects.equals(this.username, v1alpha1SnapshotStatus.username);
}
@Override
public int hashCode() {
return Objects.hash(gid, hostname, paths, tree, uid, username);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V1alpha1SnapshotStatus {\n");
sb.append(" gid: ").append(toIndentedString(gid)).append("\n");
sb.append(" hostname: ").append(toIndentedString(hostname)).append("\n");
sb.append(" paths: ").append(toIndentedString(paths)).append("\n");
sb.append(" tree: ").append(toIndentedString(tree)).append("\n");
sb.append(" uid: ").append(toIndentedString(uid)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy