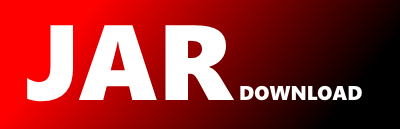
com.appsflyer.AppsFlyerProperties Maven / Gradle / Ivy
package com.appsflyer;
import android.app.Activity;
import android.content.Context;
import android.content.SharedPreferences;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.*;
/**
* static properties
*/
public class AppsFlyerProperties {
// the user id given by the app (optional)
public static final String APP_USER_ID = "AppUserId";
public static final String APP_ID = "appid";
public static final String CURRENCY_CODE = "currencyCode";
public static final String IS_UPDATE = "IS_UPDATE";
public static final String AF_KEY = "AppsFlyerKey";
public static final String USE_HTTP_FALLBACK = "useHttpFallback";
public static final String COLLECT_ANDROID_ID = "collectAndroidId";
public static final String COLLECT_IMEI = "collectIMEI";
public static final String COLLECT_FINGER_PRINT = "collectFingerPrint";
public static final String CHANNEL = "channel";
public static final String EXTENSION = "sdkExtension";
public static final String COLLECT_MAC = "collectMAC";
public static final String DEVICE_TRACKING_DISABLED = "deviceTrackingDisabled";
public static final String IS_MONITOR = "shouldMonitor";
public static final String USER_EMAIL = "userEmail"; // should be removed in the future
public static final String USER_EMAILS = "userEmails";
public static final String EMAIL_CRYPT_TYPE = "userEmailsCryptType";
public static final String ADDITIONAL_CUSTOM_DATA = "additionalCustomData";
public static final String COLLECT_FACEBOOK_ATTR_ID = "collectFacebookAttrId";
public static final String DISABLE_LOGS_COMPLETELY = "disableLogs";
private static final String SAVED_PROPERTIES = "savedProperties";
private static final String SHOULD_LOG = "shouldLog";
private static final String AF_REFERRER = "AF_REFERRER";
static final String GCM_TOKEN = "GCM_TOKEN";
static final String GCM_TOKEN_SENT_TO_SERVER = "GCM_TOKEN_SENT_TO_SERVER";
public static final String GCM_PROJECT_ID = "GCM_PROJECT_ID";
static final String GCM_INSTANCE_ID = "GCM_INSTANCE_ID";
private static AppsFlyerProperties instance = new AppsFlyerProperties();
private Map properties = new HashMap();
private boolean isOnReceiveCalled;
private boolean isLaunchCalled;
private String referrer;
public enum EmailsCryptType {
NONE(0), SHA1(1), MD5(2);
private final int value;
private EmailsCryptType(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
private AppsFlyerProperties() {
}
public static AppsFlyerProperties getInstance() {
return instance;
}
public void set(String key, String value){
properties.put(key,value);
}
public void set(String key, String value[]){
properties.put(key,value);
}
public void setCustomData(String customData){
properties.put(ADDITIONAL_CUSTOM_DATA,customData);
}
public void setUserEmails(String emails) {
properties.put(USER_EMAILS, emails);
}
public void set(String key, int value){
properties.put(key,Integer.toString(value));
}
public String[] getStringArray(String key){
return (String[]) properties.get(key);
}
public String getString(String key){
return (String) properties.get(key);
}
public void set(String key, boolean value) {
properties.put(key, Boolean.toString(value));
}
public boolean getBoolean(String key,boolean defaultValue){
String value = getString(key);
if (value == null){
return defaultValue;
}
return Boolean.valueOf(value);
}
public int getInt(String key,int defaultValue){
String value = getString(key);
if (value == null){
return defaultValue;
}
return Integer.valueOf(value);
}
protected boolean isOnReceiveCalled() {
return isOnReceiveCalled;
}
protected void setOnReceiveCalled() {
isOnReceiveCalled = true;
}
protected boolean isFirstLaunchCalled() {
return isLaunchCalled;
}
protected void setFirstLaunchCalled() {
isLaunchCalled = true;
}
protected void setReferrer(String referrer){
set(AF_REFERRER, referrer);
this.referrer = referrer;
}
public String getReferrer(Context context) {
if(referrer != null){
return referrer;
} else if (getString(AF_REFERRER) != null) {
return getString(AF_REFERRER);
}
else {
SharedPreferences sharedPreferences = context.getSharedPreferences(AppsFlyerLib.AF_SHARED_PREF, 0);
return sharedPreferences.getString(AppsFlyerLib.REFERRER_PREF,null);
}
}
public void enableLogOutput(boolean shouldEnable){
set(SHOULD_LOG, shouldEnable);
}
public boolean isEnableLog() {
boolean isEnableLog = getBoolean(SHOULD_LOG, true);
return isEnableLog;
}
public boolean isLogsDisabledCompletely() {
return getBoolean(DISABLE_LOGS_COMPLETELY, false);
}
public void saveProperties(Context context) {
String propertiesJson = new JSONObject(properties).toString();
SharedPreferences sharedPreferences = context.getSharedPreferences(AppsFlyerLib.AF_SHARED_PREF, 0);
android.content.SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putString(SAVED_PROPERTIES, propertiesJson);
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.GINGERBREAD) {
editor.apply();
} else {
editor.commit();
}
}
public void loadProperties(Context context) {
SharedPreferences sharedPreferences = context.getSharedPreferences(AppsFlyerLib.AF_SHARED_PREF, 0);
String propertiesString = sharedPreferences.getString(SAVED_PROPERTIES, null);
if (propertiesString != null) {
try {
JSONObject jsonProperties = new JSONObject(propertiesString);
Iterator iterator = jsonProperties.keys();
while (iterator.hasNext()) {
String key = (String) iterator.next();
if (properties.get(key) == null) {
properties.put(key, jsonProperties.getString(key));
}
}
} catch (JSONException jex) {
AFLogger.afLogE("Failed loading properties", jex);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy