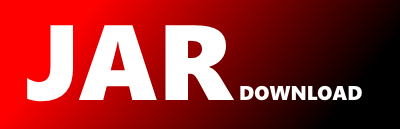
com.appslandia.plum.base.ActionDesc Maven / Gradle / Ivy
// The MIT License (MIT)
// Copyright © 2015 AppsLandia. All rights reserved.
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
package com.appslandia.plum.base;
import java.lang.reflect.Method;
import java.util.List;
/**
*
* @author Loc Ha
*
*/
public class ActionDesc {
private String controller;
private String action;
private Method method;
private Class> controllerClass;
private List paramDescs;
private List pathParams;
private int pathParamCount;
private String module;
private List allowMethods;
private String allowMethodsString;
private ConsumeType consumeType;
private EnableFilters enableFilters;
private Authorize authorize;
private CacheControl cacheControl;
private EnableCors enableCors;
private EnableHttps enableHttps;
private EnableGzip enableGzip;
private EnableParts enableParts;
private EnableEtag enableEtag;
private EnableCache enableCache;
private EnableCsrf enableCsrf;
private EnableCaptcha enableCaptcha;
private EnableLang enableLang;
private EnableJsonError enableJsonError;
private EnableAsync enableAsync;
private ChildAction childAction;
public String getController() {
return this.controller;
}
protected void setController(String controller) {
this.controller = controller;
}
public String getAction() {
return this.action;
}
protected void setAction(String action) {
this.action = action;
}
public Method getMethod() {
return this.method;
}
protected void setMethod(Method method) {
this.method = method;
}
public Class> getControllerClass() {
return this.controllerClass;
}
protected void setControllerClass(Class> controllerClass) {
this.controllerClass = controllerClass;
}
public List getParamDescs() {
return this.paramDescs;
}
protected void setParamDescs(List paramDescs) {
this.paramDescs = paramDescs;
}
public List getPathParams() {
return this.pathParams;
}
protected void setPathParams(List pathParams) {
this.pathParams = pathParams;
this.pathParamCount = ActionDescProvider.getPathParamCount(pathParams);
}
public int getPathParamCount() {
return this.pathParamCount;
}
protected void setPathParamCount(int pathParamCount) {
this.pathParamCount = pathParamCount;
}
public String getModule() {
return this.module;
}
protected void setModule(String module) {
this.module = module;
}
public List getAllowMethods() {
return this.allowMethods;
}
protected void setAllowMethods(List allowMethods) {
this.allowMethods = allowMethods;
this.allowMethodsString = String.join(", ", allowMethods);
}
public String getAllowMethodsString() {
return this.allowMethodsString;
}
protected void setAllowMethodsString(String allowMethodsString) {
this.allowMethodsString = allowMethodsString;
}
public ConsumeType getConsumeType() {
return this.consumeType;
}
protected void setConsumeType(ConsumeType consumeType) {
this.consumeType = consumeType;
}
public EnableFilters getEnableFilters() {
return this.enableFilters;
}
protected void setEnableFilters(EnableFilters enableFilters) {
this.enableFilters = enableFilters;
}
public Authorize getAuthorize() {
return this.authorize;
}
protected void setAuthorize(Authorize authorize) {
this.authorize = authorize;
}
public CacheControl getCacheControl() {
return this.cacheControl;
}
protected void setCacheControl(CacheControl cacheControl) {
this.cacheControl = cacheControl;
}
public EnableCors getEnableCors() {
return this.enableCors;
}
protected void setEnableCors(EnableCors enableCors) {
this.enableCors = enableCors;
}
public EnableHttps getEnableHttps() {
return this.enableHttps;
}
protected void setEnableHttps(EnableHttps enableHttps) {
this.enableHttps = enableHttps;
}
public EnableGzip getEnableGzip() {
return this.enableGzip;
}
protected void setEnableGzip(EnableGzip enableGzip) {
this.enableGzip = enableGzip;
}
public EnableParts getEnableParts() {
return this.enableParts;
}
protected void setEnableParts(EnableParts enableParts) {
this.enableParts = enableParts;
}
public EnableEtag getEnableEtag() {
return this.enableEtag;
}
protected void setEnableEtag(EnableEtag enableEtag) {
this.enableEtag = enableEtag;
}
public EnableCache getEnableCache() {
return this.enableCache;
}
protected void setEnableCache(EnableCache enableCache) {
this.enableCache = enableCache;
}
public EnableCsrf getEnableCsrf() {
return this.enableCsrf;
}
protected void setEnableCsrf(EnableCsrf enableCsrf) {
this.enableCsrf = enableCsrf;
}
public EnableCaptcha getEnableCaptcha() {
return this.enableCaptcha;
}
protected void setEnableCaptcha(EnableCaptcha enableCaptcha) {
this.enableCaptcha = enableCaptcha;
}
public EnableLang getEnableLang() {
return this.enableLang;
}
protected void setEnableLang(EnableLang enableLang) {
this.enableLang = enableLang;
}
public EnableAsync getEnableAsync() {
return this.enableAsync;
}
protected void setEnableAsync(EnableAsync enableAsync) {
this.enableAsync = enableAsync;
}
public EnableJsonError getEnableJsonError() {
return this.enableJsonError;
}
protected void setEnableJsonError(EnableJsonError enableJsonError) {
this.enableJsonError = enableJsonError;
}
public ChildAction getChildAction() {
return this.childAction;
}
protected void setChildAction(ChildAction childAction) {
this.childAction = childAction;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy