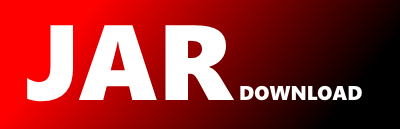
com.appslandia.plum.base.ActionParser Maven / Gradle / Ivy
// The MIT License (MIT)
// Copyright © 2015 AppsLandia. All rights reserved.
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
package com.appslandia.plum.base;
import java.util.List;
import java.util.Map;
import javax.enterprise.context.ApplicationScoped;
import javax.inject.Inject;
import javax.servlet.http.HttpServletRequest;
import com.appslandia.common.utils.StringUtils;
import com.appslandia.common.utils.URLEncoding;
import com.appslandia.common.utils.URLUtils;
import com.appslandia.plum.utils.ServletUtils;
/**
*
* @author Loc Ha
*
*/
@ApplicationScoped
public class ActionParser {
@Inject
protected AppConfig appConfig;
@Inject
protected ActionDescProvider actionDescProvider;
@Inject
protected LanguageProvider languageProvider;
public ActionDesc parse(List pathItems, Map pathParamMap) {
// /
// /language.extension
if (pathItems.size() == 0) {
return this.actionDescProvider.getHomeDesc();
}
ActionDesc actionDesc = null;
String controller = pathItems.get(0);
// /controller.extension
if (pathItems.size() == 1) {
actionDesc = this.actionDescProvider.getActionDesc(controller, ServletUtils.ACTION_INDEX);
if ((actionDesc == null) || (actionDesc.getChildAction() != null)) {
return null;
}
if (actionDesc.getPathParams().size() > 0) {
return null;
}
return actionDesc;
}
// /controller/action(/parameter)*.extension
String action = pathItems.get(1);
actionDesc = this.actionDescProvider.getActionDesc(controller, action);
if ((actionDesc == null) || (actionDesc.getChildAction() != null)) {
return null;
}
// Remove controller/action
pathItems.remove(0);
pathItems.remove(0);
List pathParams = actionDesc.getPathParams();
if (pathItems.size() != pathParams.size()) {
return null;
}
// pathParamMap
for (int i = 0; i < pathParams.size(); i++) {
PathParam pathParm = pathParams.get(i);
if (pathParm.getParamName() != null) {
pathParamMap.put(pathParm.getParamName(), URLEncoding.decodePath(pathItems.get(i)));
} else {
if (!parseSubParams(pathItems.get(i), pathParm.getSubParams(), pathParamMap)) {
return null;
}
}
}
return actionDesc;
}
public String toActionUrl(HttpServletRequest request, String language, String controller, String action, Map parameters, boolean absoluteUrl)
throws IllegalArgumentException {
ActionDesc actionDesc = this.actionDescProvider.getActionDesc(controller, action);
if ((actionDesc == null) || (actionDesc.getChildAction() != null)) {
throw new IllegalArgumentException("Action is required (controller=" + controller + ", action=" + action + ")");
}
RequestContext requestContext = ServletUtils.getRequestContext(request);
StringBuilder url = new StringBuilder(controller.length() + action.length() + 5 + ((parameters != null) ? 16 * parameters.size() : 0));
// protocol://serverName:serverPort
if (absoluteUrl) {
if ((actionDesc.getEnableHttps() != null) || request.isSecure()) {
url.append("https://").append(request.getServerName());
if (this.appConfig.getHttpsPort() != 443) {
url.append(':').append(this.appConfig.getHttpsPort());
}
} else {
url.append("http://").append(request.getServerName());
if (this.appConfig.getHttpPort() != 80) {
url.append(':').append(this.appConfig.getHttpPort());
}
}
}
// Context path
url.append(request.getServletContext().getContextPath());
// Language
if (language == null) {
if (requestContext.isPathLanguage()) {
url.append('/').append(requestContext.getLanguage().getId());
}
} else {
if (this.languageProvider.getLanguage(language) == null) {
throw new IllegalArgumentException("Language is not supported (language=" + language + ")");
}
url.append('/').append(language);
}
// Controller/Action
url.append('/').append(controller).append('/').append(action);
// Path Parameters
if (parameters != null) {
addPathParams(url, parameters, actionDesc.getPathParams());
} else if (actionDesc.getPathParams().size() > 0) {
throw new IllegalArgumentException("Path parameters are required.");
}
// Query Parameters
if ((parameters != null) && (parameters.size() > actionDesc.getPathParamCount())) {
url.append('?');
addQueryParams(url, parameters, actionDesc.getPathParams());
}
return url.toString();
}
// pathItem: parameter(-parameter)+
public static boolean parseSubParams(String pathItem, List subParams, Map pathParamMap) {
int nextIdx = 0;
int startIdx = 0;
while (true) {
// Last parameter
if (nextIdx == subParams.size() - 1) {
if (startIdx >= pathItem.length()) {
return false;
}
String value = pathItem.substring(startIdx);
if (value.isEmpty()) {
return false;
}
pathParamMap.put(subParams.get(nextIdx).getParamName(), URLEncoding.decodePath(value));
return true;
}
// Not last parameter
int endIdx = pathItem.indexOf('-', startIdx);
if (endIdx < 0) {
return false;
}
String value = pathItem.substring(startIdx, endIdx);
if (value.isEmpty()) {
return false;
}
pathParamMap.put(subParams.get(nextIdx).getParamName(), URLEncoding.decodePath(value));
startIdx = endIdx + 1;
nextIdx++;
}
}
public static void addPathParams(StringBuilder url, Map parameters, List pathParams) {
for (PathParam pathParam : pathParams) {
if (pathParam.getParamName() != null) {
Object value = parameters.get(pathParam.getParamName());
if (value == null) {
throw new IllegalArgumentException("Path parameter is required (name=" + pathParam.getParamName() + ")");
}
url.append('/').append(URLEncoding.encodePathXmlSafe(value.toString()));
continue;
}
// Sub Parameters
boolean isFirstSub = true;
for (PathParam subParam : pathParam.getSubParams()) {
Object value = parameters.get(subParam.getParamName());
if (value == null) {
throw new IllegalArgumentException("Path parameter is required (name=" + subParam.getParamName() + ")");
}
if (isFirstSub) {
url.append('/').append(URLEncoding.encodePathXmlSafe(value.toString()));
isFirstSub = false;
} else {
url.append('-').append(URLEncoding.encodePathXmlSafe(value.toString()));
}
}
}
}
public static final String PARAM_QUERY_STRING = "__QUERY_STRING";
public static void addQueryParams(StringBuilder url, Map parameters, List pathParams) {
// QUERY_STRING
String queryString = (String) parameters.get(PARAM_QUERY_STRING);
boolean hasQueryString = !StringUtils.isNullOrEmpty(queryString);
if (hasQueryString) {
url.append(queryString);
}
boolean isFirstParam = !hasQueryString;
for (Map.Entry param : parameters.entrySet()) {
if (pathParams.stream().anyMatch(p -> p.hasPathParam(param.getKey()))) {
continue;
}
if (PARAM_QUERY_STRING.equals(param.getKey())) {
continue;
}
if (isFirstParam) {
isFirstParam = false;
} else {
url.append('&');
}
URLUtils.addQueryParam(url, param.getKey(), param.getValue());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy