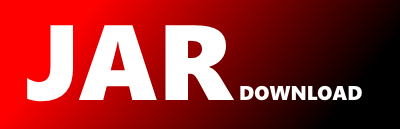
com.appslandia.plum.base.CookieTempDataManager Maven / Gradle / Ivy
// The MIT License (MIT)
// Copyright © 2015 AppsLandia. All rights reserved.
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
package com.appslandia.plum.base;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import javax.inject.Inject;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.appslandia.common.base.BaseEncoder;
import com.appslandia.common.base.TextGenerator;
import com.appslandia.common.cdi.Json;
import com.appslandia.common.cdi.Json.Profile;
import com.appslandia.common.json.JsonProcessor;
import com.appslandia.common.utils.AssertUtils;
import com.appslandia.plum.utils.ServletUtils;
/**
*
* @author Loc Ha
*
*/
public class CookieTempDataManager extends TempDataManager {
public static final String DEFAULT_COOKIE_NAME = "tempData";
public static final int DEFAULT_COOKIE_AGE = (int) TimeUnit.SECONDS.convert(15, TimeUnit.SECONDS);
public static final String CONFIG_COOKIE_NAME = CookieTempDataManager.class.getName() + ".cookie_name";
public static final String CONFIG_COOKIE_AGE = CookieTempDataManager.class.getName() + ".cookie_age";
@Inject
protected AppConfig appConfig;
@Inject
protected CookieHandler cookieHandler;
@Inject
@Json(Profile.COMPACT)
protected JsonProcessor jsonProcessor;
protected String getCookieName() {
return this.appConfig.getString(CONFIG_COOKIE_NAME, DEFAULT_COOKIE_NAME);
}
protected int getCookieAge() {
return this.appConfig.getInt(CONFIG_COOKIE_AGE, DEFAULT_COOKIE_AGE);
}
protected String encode(TempData tempData) {
return BaseEncoder.BASE64_URL_NP.encode(tempData.mapAsJson(this.jsonProcessor).getBytes(StandardCharsets.UTF_8));
}
protected TempData decode(String tempData) {
try {
Map map = this.jsonProcessor.readAsMap(new StringReader(new String(BaseEncoder.BASE64_URL_NP.decode(tempData), StandardCharsets.UTF_8)));
return new TempData(map);
} catch (Exception ex) {
return null;
}
}
@Override
public String saveTempData(HttpServletRequest request, HttpServletResponse response, TempData tempData) {
AssertUtils.assertNotNull(tempData);
String cookieValue = encode(tempData);
if (cookieValue.length() > 4093) {
throw new IllegalArgumentException("TempData is too big.");
}
this.cookieHandler.saveCookie(response, getCookieName(), cookieValue, getCookieAge(), c -> c.setHttpOnly(true));
return null;
}
@Override
public TempData loadTempData(HttpServletRequest request, HttpServletResponse response) {
String cookieValue = ServletUtils.getCookieValue(request, getCookieName());
if (cookieValue == null) {
return null;
}
this.cookieHandler.removeCookie(response, getCookieName());
TempData tempData = decode(cookieValue);
if (tempData == null) {
return null;
}
request.setAttribute(TempData.REQUEST_ATTRIBUTE_ID, tempData);
return tempData;
}
@Override
protected TextGenerator getTempDataIdGenerator() {
throw new UnsupportedOperationException();
}
@Override
protected void doSaveTempData(HttpServletRequest request, HttpServletResponse response, String tempDataId, TempData tempData) {
throw new UnsupportedOperationException();
}
@Override
protected TempData doLoadTempData(HttpServletRequest request, HttpServletResponse response, String tempDataId) {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy