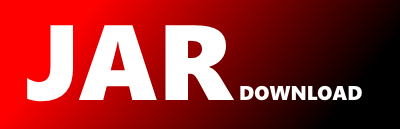
com.appslandia.plum.base.RequestAccessor Maven / Gradle / Ivy
// The MIT License (MIT)
// Copyright © 2015 AppsLandia. All rights reserved.
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
package com.appslandia.plum.base;
import java.util.Arrays;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Stream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletRequestWrapper;
import com.appslandia.common.formatters.FormatterException;
import com.appslandia.common.utils.AssertUtils;
import com.appslandia.common.utils.ObjectUtils;
import com.appslandia.common.utils.StringUtils;
import com.appslandia.plum.utils.ServletUtils;
/**
*
* @author Loc Ha
*
*/
public class RequestAccessor extends HttpServletRequestWrapper {
public static final String PARAM_SUBMIT_ACTION = "submitAction";
public RequestAccessor(HttpServletRequest request) {
super(request);
}
public void bindModel(Object model) throws Exception {
ServletUtils.getAppScoped(this, ModelBinder.class).bindModel(this, model);
}
public void bindModel(Object model, Function excludePaths) throws Exception {
ServletUtils.getAppScoped(this, ModelBinder.class).bindModel(this, model, excludePaths);
}
public AppConfig getAppConfig() {
return ServletUtils.getAppScoped(this, AppConfig.class);
}
public Stream getParamValues(String name) {
String[] values = getParameterValues(name);
return Arrays.stream((values != null) ? values : StringUtils.EMPTY_ARRAY);
}
public T findParamValue(String name, Class targetType) throws IllegalArgumentException, FormatterException {
String[] values = getParameterValues(name);
if (values == null) {
return null;
}
String value = Arrays.stream(values).filter(v -> !StringUtils.isNullOrEmpty(v)).findFirst().orElse(null);
return ObjectUtils.cast(getRequestContext().getFormatterProvider().getFormatter(targetType).parse(value, getRequestContext().getFormatProvider()));
}
public T getParamValue(String name, Class targetType) throws IllegalArgumentException, FormatterException {
String value = getParameter(name);
if (value == null) {
return null;
}
return ObjectUtils.cast(getRequestContext().getFormatterProvider().getFormatter(targetType).parse(value, getRequestContext().getFormatProvider()));
}
public boolean isGetOrHead() {
return getRequestContext().isGetOrHead();
}
public boolean isLocalhost() {
return ServletUtils.isLocalhost(this);
}
public boolean isAjaxRequest() {
return ServletUtils.isAjaxRequest(this);
}
public String paramToNull(String name) {
return StringUtils.trimToNull(getParameter(name));
}
public PrefCookie getPrefCookie() {
return ServletUtils.getPrefCookie(this);
}
public String getPrefCookie(String name) {
PrefCookie prefCookie = getPrefCookie();
return (prefCookie != null) ? prefCookie.getString(name) : null;
}
public String getReturnUrl() {
return paramToNull(ServletUtils.PARAM_RETURN_URL);
}
public String getSubmitAction() {
return getParameter(PARAM_SUBMIT_ACTION);
}
public boolean isSaveAction() {
return "save".equalsIgnoreCase(getSubmitAction());
}
public boolean isSaveContAction() {
return "saveCont".equalsIgnoreCase(getSubmitAction());
}
public boolean isDeleteAction() {
return "delete".equalsIgnoreCase(getSubmitAction());
}
public void store(String key, Object value) {
setAttribute(key, value);
}
public void storeModel(Object model) {
store(ServletUtils.REQUEST_ATTRIBUTE_MODEL, model);
}
public void storePagerModel(PagerModel pagerModel) {
store(PagerModel.REQUEST_ATTRIBUTE_ID, pagerModel);
}
public void storeSortBag(SortBag sortBag) {
store(SortBag.REQUEST_ATTRIBUTE_ID, sortBag);
}
public boolean hasPrincipalForCurrentModule() {
return hasPrincipal() && getRequestContext().getModule().equalsIgnoreCase(getUserPrincipal().getModule());
}
public boolean hasPrincipal() {
return getUserPrincipal() != null;
}
@Override
public UserPrincipal getUserPrincipal() {
return ServletUtils.getUserPrincipal((HttpServletRequest) super.getRequest());
}
public UserPrincipal getRequiredPrincipal() {
return AssertUtils.assertStateNotNull(getUserPrincipal(), "getUserPrincipal() must be not null.");
}
public int getUserId() {
return getRequiredPrincipal().getUserId();
}
public boolean isUserInRoles(String... roles) {
AssertUtils.assertHasElements(roles);
return Arrays.stream(roles).anyMatch(role -> isUserInRole(role));
}
public String toActionUrl(String language, String controller, String action, Map parameters) throws IllegalArgumentException {
return ServletUtils.getAppScoped(this, ActionParser.class).toActionUrl(this, language, controller, action, parameters, false);
}
public String toAbsActionUrl(String language, String controller, String action, Map parameters) throws IllegalArgumentException {
return ServletUtils.getAppScoped(this, ActionParser.class).toActionUrl(this, language, controller, action, parameters, true);
}
public Problem parseProblem(Throwable throwable) {
return ServletUtils.getAppScoped(this, ExceptionHandler.class).getProblem(this, getRequestContext(), throwable);
}
public RequestContext getRequestContext() {
return ServletUtils.getRequestContext(this);
}
public ModelState getModelState() {
return ServletUtils.getModelState(this);
}
public TempData getTempData() {
return ServletUtils.getTempData(this);
}
public ViewBag getViewBag() {
return ServletUtils.getViewBag(this);
}
public Messages getMessages() {
return ServletUtils.getMessages(this);
}
public Resources getResources() {
return getRequestContext().getResources();
}
public String getResource(String key) {
return getResources().get(key);
}
public String getResource(String key, Object... params) {
return getResources().get(key, params);
}
public String getResource(String key, Map params) {
return getResources().get(key, params);
}
public void assertTrue(boolean expr) throws BadRequestException {
if (!expr) {
throw new BadRequestException(Resources.ERROR_BAD_REQUEST, getResources());
}
}
public T assertNotNull(T value) throws BadRequestException {
if (value == null) {
throw new BadRequestException(Resources.ERROR_BAD_REQUEST, getResources());
}
return value;
}
public void assertPositive(int value) throws BadRequestException {
if (value <= 0) {
throw new BadRequestException(Resources.ERROR_BAD_REQUEST, getResources());
}
}
public void assertValidFields(String... fieldNames) throws BadRequestException {
if (!getModelState().areValid(fieldNames)) {
throw new BadRequestException(Resources.ERROR_BAD_REQUEST, getResources());
}
}
public void assertValidModel() throws BadRequestException {
if (!getModelState().isValid()) {
throw new BadRequestException(Resources.ERROR_BAD_REQUEST, getResources());
}
}
public void assertInRoles(String[] roles) throws ForbiddenException {
AssertUtils.assertHasElements(roles);
if (!isUserInRoles(roles)) {
throw new ForbiddenException(Resources.ERROR_FORBIDDEN, getResources());
}
}
public void assertForbidden(boolean expr) throws ForbiddenException {
if (!expr) {
throw new ForbiddenException(Resources.ERROR_FORBIDDEN, getResources());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy