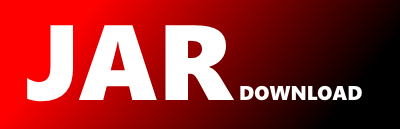
com.appslandia.plum.base.RequestContext Maven / Gradle / Ivy
// The MIT License (MIT)
// Copyright © 2015 AppsLandia. All rights reserved.
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
package com.appslandia.plum.base;
import java.util.Map;
import com.appslandia.common.base.FormatProvider;
import com.appslandia.common.base.Language;
import com.appslandia.common.formatters.Formatter;
import com.appslandia.common.formatters.FormatterProvider;
import com.appslandia.plum.tags.XmlEscaper;
/**
*
* @author Loc Ha
*
*/
public class RequestContext {
public static final String REQUEST_ATTRIBUTE_ID = "ctx";
private boolean pathLanguage;
private FormatProvider formatProvider;
private Resources resources;
private FormatterProvider formatterProvider;
private boolean getOrHead;
private ActionDesc actionDesc;
private Map pathParamMap;
private String module;
public RequestContext createRequestContext(ActionDesc actionDesc) {
RequestContext context = new RequestContext();
context.pathLanguage = this.pathLanguage;
context.formatProvider = this.formatProvider;
context.resources = this.resources;
context.formatterProvider = this.formatterProvider;
context.getOrHead = this.getOrHead;
context.actionDesc = actionDesc;
context.module = this.module;
return context;
}
public boolean isRoute(String action, String controller) {
if (this.actionDesc == null) {
return false;
}
return this.actionDesc.getAction().equalsIgnoreCase(action) && this.actionDesc.getController().equalsIgnoreCase(controller);
}
public Language getLanguage() {
return this.formatProvider.getLanguage();
}
public boolean isPathLanguage() {
return this.pathLanguage;
}
protected void setPathLanguage(boolean pathLanguage) {
this.pathLanguage = pathLanguage;
}
public FormatProvider getFormatProvider() {
return this.formatProvider;
}
protected void setFormatProvider(FormatProvider formatProvider) {
this.formatProvider = formatProvider;
}
public Resources getResources() {
return this.resources;
}
protected void setResources(Resources resources) {
this.resources = resources;
}
public FormatterProvider getFormatterProvider() {
return this.formatterProvider;
}
protected void setFormatterProvider(FormatterProvider formatterProvider) {
this.formatterProvider = formatterProvider;
}
public boolean isGetOrHead() {
return this.getOrHead;
}
protected void setGetOrHead(boolean getOrHead) {
this.getOrHead = getOrHead;
}
public ActionDesc getActionDesc() {
return this.actionDesc;
}
protected void setActionDesc(ActionDesc actionDesc) {
this.actionDesc = actionDesc;
}
public Map getPathParamMap() {
return this.pathParamMap;
}
protected void setPathParamMap(Map pathParamMap) {
this.pathParamMap = pathParamMap;
}
public String getModule() {
return this.module;
}
protected void setModule(String module) {
this.module = module;
}
public String res(String key) {
return this.resources.get(key);
}
public String res(String key, Object p1) {
return this.resources.get(key, p1);
}
public String res(String key, Object p1, Object p2) {
return this.resources.get(key, p1, p2);
}
public String res(String key, Object p1, Object p2, Object p3) {
return this.resources.get(key, p1, p2, p3);
}
public String esc(String key) {
return XmlEscaper.escapeXml(this.resources.get(key));
}
public String esc(String key, Object p1) {
return XmlEscaper.escapeXml(this.resources.get(key, p1));
}
public String esc(String key, Object p1, Object p2) {
return XmlEscaper.escapeXml(this.resources.get(key, p1, p2));
}
public String esc(String key, Object p1, Object p2, Object p3) {
return XmlEscaper.escapeXml(this.resources.get(key, p1, p2, p3));
}
public String ifEsc(boolean b, String trueKey) {
if (b) {
return XmlEscaper.escapeXml(this.resources.get(trueKey));
}
return null;
}
public String ifEsc(boolean b, String trueKey, Object p1) {
if (b) {
return XmlEscaper.escapeXml(this.resources.get(trueKey, p1));
}
return null;
}
public String ifEsc(boolean b, String trueKey, Object p1, Object p2) {
if (b) {
return XmlEscaper.escapeXml(this.resources.get(trueKey, p1, p2));
}
return null;
}
public String ifEsc(boolean b, String trueKey, Object p1, Object p2, Object p3) {
if (b) {
return XmlEscaper.escapeXml(this.resources.get(trueKey, p1, p2, p3));
}
return null;
}
public String iifEsc(boolean b, String trueKey, String falseKey) {
if (b) {
return XmlEscaper.escapeXml(this.resources.get(trueKey));
}
return XmlEscaper.escapeXml(this.resources.get(falseKey));
}
public String escCt(String key) {
return XmlEscaper.escapeXmlContent(this.resources.get(key));
}
public String escCt(String key, Object p1) {
return XmlEscaper.escapeXmlContent(this.resources.get(key, p1));
}
public String escCt(String key, Object p1, Object p2) {
return XmlEscaper.escapeXmlContent(this.resources.get(key, p1, p2));
}
public String escCt(String key, Object p1, Object p2, Object p3) {
return XmlEscaper.escapeXmlContent(this.resources.get(key, p1, p2, p3));
}
public String ifEscCt(boolean b, String trueKey) {
if (b) {
return XmlEscaper.escapeXmlContent(this.resources.get(trueKey));
}
return null;
}
public String ifEscCt(boolean b, String trueKey, Object p1) {
if (b) {
return XmlEscaper.escapeXmlContent(this.resources.get(trueKey, p1));
}
return null;
}
public String ifEscCt(boolean b, String trueKey, Object p1, Object p2) {
if (b) {
return XmlEscaper.escapeXmlContent(this.resources.get(trueKey, p1, p2));
}
return null;
}
public String ifEscCt(boolean b, String trueKey, Object p1, Object p2, Object p3) {
if (b) {
return XmlEscaper.escapeXmlContent(this.resources.get(trueKey, p1, p2, p3));
}
return null;
}
public String iifEscCt(boolean b, String trueKey, String falseKey) {
if (b) {
return XmlEscaper.escapeXmlContent(this.resources.get(trueKey));
}
return XmlEscaper.escapeXmlContent(this.resources.get(falseKey));
}
public String countEsc(String hasCountKey, Number count, String zeroOrNullKey) {
if (count == null || count.intValue() == 0) {
return XmlEscaper.escapeXml(this.resources.get(zeroOrNullKey));
}
return XmlEscaper.escapeXml(this.resources.get(hasCountKey, count.intValue()));
}
public String countEscCt(String hasCountKey, Number count, String zeroOrNullKey) {
if (count == null || count.intValue() == 0) {
return XmlEscaper.escapeXmlContent(this.resources.get(zeroOrNullKey));
}
return XmlEscaper.escapeXmlContent(this.resources.get(hasCountKey, count.intValue()));
}
public String format(Object value) {
if (value == null) {
return null;
}
return format(value, null);
}
public String format(Object value, String formatterId) {
if (value == null) {
return null;
}
Formatter formatter = this.formatterProvider.findFormatter(formatterId, value.getClass());
if (formatter != null) {
return formatter.format(value, this.formatProvider);
} else {
return value.toString();
}
}
public String fmtEsc(Object value, String formatterId) {
if (value == null) {
return null;
}
return XmlEscaper.escapeXml(format(value, formatterId));
}
public String fmtEscCt(Object value, String formatterId) {
if (value == null) {
return null;
}
return XmlEscaper.escapeXmlContent(format(value, formatterId));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy