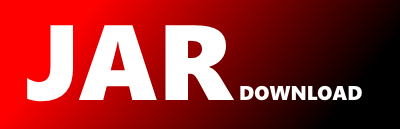
com.appslandia.plum.base.ResponseKeyBuilder Maven / Gradle / Ivy
// The MIT License (MIT)
// Copyright © 2015 AppsLandia. All rights reserved.
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
package com.appslandia.plum.base;
import java.util.HashMap;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.servlet.http.HttpServletRequest;
import com.appslandia.common.utils.URLEncoding;
import com.appslandia.common.utils.URLUtils;
import com.appslandia.plum.utils.ServletUtils;
/**
*
* @author Loc Ha
*
*/
public class ResponseKeyBuilder {
public static final ResponseKeyBuilder INSTANCE = new ResponseKeyBuilder();
public Map getKeyParams(HttpServletRequest request) {
RequestContext requestContext = ServletUtils.getRequestContext(request);
Map parameters = new HashMap<>();
parameters.put("language", requestContext.getLanguage().getId());
parameters.put("controller", requestContext.getActionDesc().getController());
parameters.put("action", requestContext.getActionDesc().getAction());
initKeyParams(request, parameters);
return parameters;
}
protected void initKeyParams(HttpServletRequest request, Map parameters) {
}
public String getResponseKey(HttpServletRequest request, String keyUri, boolean gzipContent) {
return buildResponseKey(keyUri, getKeyParams(request), gzipContent);
}
public static String getResponseKey(RequestContext requestContext, String languageId) {
Map parameters = new HashMap<>();
parameters.put("language", languageId);
parameters.put("controller", requestContext.getActionDesc().getController());
parameters.put("action", requestContext.getActionDesc().getAction());
String keyUri = (languageId != null) ? "/{language}/{controller}/{action}" : "/{controller}/{action}";
return buildResponseKey(keyUri, parameters, false);
}
private static final Pattern PATH_PARAMS_PATTERN = Pattern.compile("\\{[^}]+?}");
private static String buildResponseKey(String keyUri, Map parameters, boolean gzipContent) {
Matcher matcher = PATH_PARAMS_PATTERN.matcher(keyUri);
StringBuilder sb = new StringBuilder(keyUri.length() + ((parameters != null) ? 16 * parameters.size() : 0));
int prevEnd = 0;
while (matcher.find()) {
// Non parameter
if (prevEnd == 0) {
sb.append(keyUri.substring(0, matcher.start()));
} else {
sb.append(keyUri.substring(prevEnd, matcher.start()));
}
// {parameter}
String parameterGroup = matcher.group();
String parameterName = parameterGroup.substring(1, parameterGroup.length() - 1);
Object parameterVal = parameters.get(parameterName);
if (parameterVal == null) {
throw new IllegalArgumentException("Path parameter is required (name=" + parameterName + ")");
}
sb.append(URLEncoding.encodePath(parameterVal.toString()));
parameters.remove(parameterName);
prevEnd = matcher.end();
}
if (prevEnd < keyUri.length()) {
sb.append(keyUri.substring(prevEnd));
}
// Query String
boolean firstParam = true;
boolean hasQuery = false;
for (Map.Entry param : parameters.entrySet()) {
if (param.getKey().equals("language") || param.getKey().equals("controller") || param.getKey().equals("action")) {
continue;
}
if (!firstParam) {
sb.append('&');
} else {
sb.append('?');
firstParam = false;
}
hasQuery = true;
URLUtils.addQueryParam(sb, param.getKey(), param.getValue());
}
// gzipContent
if (gzipContent) {
sb.append(hasQuery ? "&__gzipContent=true" : "?__gzipContent=true");
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy