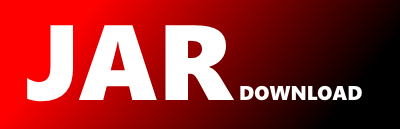
com.appslandia.plum.utils.ServletUtils Maven / Gradle / Ivy
// The MIT License (MIT)
// Copyright © 2015 AppsLandia. All rights reserved.
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
package com.appslandia.plum.utils;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.io.Writer;
import java.security.Principal;
import java.util.Arrays;
import java.util.Collection;
import java.util.Enumeration;
import java.util.Locale;
import java.util.Map;
import java.util.Properties;
import javax.enterprise.inject.spi.CDI;
import javax.servlet.FilterConfig;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletRequestWrapper;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpServletResponseWrapper;
import javax.servlet.http.HttpSession;
import com.appslandia.common.base.FormatProvider;
import com.appslandia.common.base.PropertyConfig;
import com.appslandia.common.utils.AssertUtils;
import com.appslandia.common.utils.HexUtils;
import com.appslandia.common.utils.IOUtils;
import com.appslandia.common.utils.ObjectUtils;
import com.appslandia.common.utils.StringUtils;
import com.appslandia.common.utils.URLEncoding;
import com.appslandia.common.utils.ValueUtils;
import com.appslandia.plum.base.ActionDesc;
import com.appslandia.plum.base.ActionDescProvider;
import com.appslandia.plum.base.Messages;
import com.appslandia.plum.base.ModelState;
import com.appslandia.plum.base.MutexContextListener;
import com.appslandia.plum.base.MutexSessionListener;
import com.appslandia.plum.base.PrefCookie;
import com.appslandia.plum.base.RequestContext;
import com.appslandia.plum.base.Resources;
import com.appslandia.plum.base.TempData;
import com.appslandia.plum.base.UserPrincipal;
import com.appslandia.plum.base.ViewBag;
/**
*
* @author Loc Ha
*
*/
public class ServletUtils {
public static final String ACTION_INDEX = "index";
public static final String REQUEST_ATTRIBUTE_MODEL = "model";
public static final String PARAM_RETURN_URL = "returnUrl";
public static String getUriAndQuery(HttpServletRequest request) {
if (request.getQueryString() != null) {
return request.getRequestURI() + "?" + request.getQueryString();
}
return request.getRequestURI();
}
public static StringBuilder getFormLoginUrl(HttpServletRequest request) {
RequestContext requestContext = getRequestContext(request);
ActionDesc formLogin = getAppScoped(request, ActionDescProvider.class).getFormLogin(requestContext.getModule());
StringBuilder url = new StringBuilder();
url.append(request.getServletContext().getContextPath());
if (requestContext.isPathLanguage()) {
url.append('/').append(requestContext.getLanguage().getId());
}
url.append('/').append(formLogin.getController()).append('/').append(formLogin.getAction());
return url;
}
public static String getSecureUrl(HttpServletRequest request, int httpsPort) {
StringBuilder url = new StringBuilder();
url.append("https://").append(request.getServerName());
if (httpsPort == 443) {
url.append(getUriAndQuery(request));
} else {
url.append(':').append(httpsPort).append(getUriAndQuery(request));
}
return url.toString();
}
public static String getClientIpInfo(HttpServletRequest request) {
StringBuilder sb = new StringBuilder();
sb.append("RemoteAddr=").append(request.getRemoteAddr());
String forwarded = request.getHeader("Forwarded");
if (forwarded != null) {
sb.append(", Forwarded=").append(forwarded);
}
forwarded = request.getHeader("X-Forwarded-For");
if (forwarded != null) {
sb.append(", X-Forwarded-For=").append(forwarded);
}
return sb.toString();
}
public static boolean isLocalhost(HttpServletRequest request) {
return request.getServerName().contains("localhost") || "127.0.0.1".equals(request.getServerName()) || "::ffff:127.0.0.1".equals(request.getServerName())
|| "::1".equals(request.getServerName()) || "0:0:0:0:0:0:0:1".equals(request.getServerName());
}
public static String getAppDir(ServletContext sc) {
String appDir = sc.getRealPath("/");
AssertUtils.assertNotNull(appDir, "Can't determine appDir.");
return appDir;
}
public static boolean isGzipAccepted(HttpServletRequest request) {
String ae = request.getHeader("Accept-Encoding");
return (ae != null) && (ae.toLowerCase(Locale.ENGLISH).contains("gzip"));
}
public static boolean isAjaxRequest(HttpServletRequest request) {
String xrw = request.getHeader("X-Requested-With");
return "XMLHttpRequest".equalsIgnoreCase(xrw);
}
public static void setContentDisposition(HttpServletResponse response, String fileName, boolean inline) {
String dispositionType = inline ? "inline" : "attachment";
response.setHeader("Content-Disposition", dispositionType + "; filename=\"" + URLEncoding.encodePath(fileName) + "\"");
}
public static boolean allowContentType(String contentType, String allowType) {
if (contentType == null) {
return false;
}
int idx = contentType.indexOf(';');
String mimeType = (idx < 0) ? contentType : contentType.substring(0, idx);
return allowType.equalsIgnoreCase(mimeType);
}
public static String parseLanguage(HttpServletRequest request, Collection supportedLanguages) {
Enumeration requestLocales = request.getLocales();
while (requestLocales.hasMoreElements()) {
Locale requestLocale = requestLocales.nextElement();
String matchedLang = supportedLanguages.stream().filter(sl -> new Locale(sl).getLanguage().equals(requestLocale.getLanguage())).findFirst().orElse(null);
if (matchedLang != null) {
return matchedLang;
}
}
return null;
}
public static Object getMutex(HttpSession session) {
Object mutex = session.getAttribute(MutexSessionListener.ATTRIBUTE_MUTEX);
return (mutex != null) ? mutex : session;
}
public static Object getMutex(ServletContext context) {
Object mutex = context.getAttribute(MutexContextListener.ATTRIBUTE_MUTEX);
return (mutex != null) ? mutex : context;
}
public static Writer getWriter(HttpServletResponse response) throws IOException {
try {
return response.getWriter();
} catch (IllegalStateException ex) {
return new BufferedWriter(new OutputStreamWriter(response.getOutputStream(), response.getCharacterEncoding()));
}
}
public static PrintWriter getPrintWriter(HttpServletResponse response) throws IOException {
try {
return response.getWriter();
} catch (IllegalStateException ex) {
return new PrintWriter(new BufferedWriter(new OutputStreamWriter(response.getOutputStream(), response.getCharacterEncoding())));
}
}
public static HttpServletResponse assertNotCommitted(HttpServletResponse response) throws IllegalStateException {
if (response.isCommitted()) {
throw new IllegalStateException("The response is already committed.");
}
return response;
}
public static RequestDispatcher getRequestDispatcher(HttpServletRequest request, String location) {
RequestDispatcher dispatcher = request.getRequestDispatcher(location);
if (dispatcher == null) {
throw new IllegalArgumentException("getRequestDispatcher (location=" + location + ")");
}
return dispatcher;
}
public static void forward(HttpServletRequest request, HttpServletResponse response, String location) throws ServletException, IOException {
getRequestDispatcher(request, location).forward(request, assertNotCommitted(response));
}
public static void include(HttpServletRequest request, HttpServletResponse response, String location) throws ServletException, IOException {
getRequestDispatcher(request, location).include(request, response);
}
public static void sendRedirect(HttpServletResponse response, String location, int status) throws IllegalStateException {
assertNotCommitted(response);
response.resetBuffer();
response.setHeader("Location", location);
response.setStatus(status);
}
public static String toWrapperPath(HttpServletRequest request) {
StringBuilder sb = new StringBuilder();
HttpServletRequest req = request;
while (true) {
if (sb.length() == 0) {
sb.append(ObjectUtils.toObjectInfo(req));
} else {
sb.append(" > ").append(ObjectUtils.toObjectInfo(req));
}
if (!(req instanceof HttpServletRequestWrapper)) {
return sb.toString();
}
req = (HttpServletRequest) ((HttpServletRequestWrapper) req).getRequest();
}
}
public static String toWrapperPath(HttpServletResponse response) {
StringBuilder sb = new StringBuilder();
HttpServletResponse resp = response;
while (true) {
if (sb.length() == 0) {
sb.append(ObjectUtils.toObjectInfo(resp));
} else {
sb.append(" > ").append(ObjectUtils.toObjectInfo(resp));
}
if (!(resp instanceof HttpServletResponseWrapper)) {
return sb.toString();
}
resp = (HttpServletResponse) ((HttpServletResponseWrapper) resp).getResponse();
}
}
public static T unwrapRequest(HttpServletRequest request, Class toImpl) {
HttpServletRequest req = request;
while (true) {
if (req.getClass() == toImpl) {
return ObjectUtils.cast(req);
}
if (!(req instanceof HttpServletRequestWrapper)) {
return null;
}
req = (HttpServletRequest) ((HttpServletRequestWrapper) req).getRequest();
}
}
public static T unwrapResponse(HttpServletResponse response, Class toImpl) {
HttpServletResponse resp = response;
while (true) {
if (resp.getClass() == toImpl) {
return ObjectUtils.cast(resp);
}
if (!(resp instanceof HttpServletResponseWrapper)) {
return null;
}
resp = (HttpServletResponse) ((HttpServletResponseWrapper) resp).getResponse();
}
}
public static String getCookieValue(HttpServletRequest request, String name) {
Cookie[] cookies = request.getCookies();
if (cookies == null) {
return null;
}
Cookie cookie = Arrays.stream(cookies).filter(c -> c.getName().equalsIgnoreCase(name)).findFirst().orElse(null);
return (cookie != null) ? cookie.getValue() : null;
}
public static void removeCookie(HttpServletResponse response, String name, String domain, String path) {
Cookie cookie = new Cookie(name, StringUtils.EMPTY_STRING);
cookie.setMaxAge(0);
if (domain != null) {
cookie.setDomain(domain);
}
cookie.setPath(path);
response.addCookie(cookie);
}
public static String getCookiePath(ServletContext sc) {
String path = ValueUtils.valueOrAlt(sc.getSessionCookieConfig().getPath(), sc.getContextPath());
return !path.isEmpty() ? path : "/";
}
public static String generateEtag(byte[] md5) {
StringBuilder sb = new StringBuilder(36);
sb.append("W/\"");
HexUtils.appendAsHex(sb, md5);
sb.append('"');
return sb.toString();
}
public static boolean checkNotModified(HttpServletRequest request, HttpServletResponse response, long lastModifiedMs) {
response.setDateHeader("Last-Modified", lastModifiedMs);
long ifModifiedSince = request.getDateHeader("If-Modified-Since");
boolean notModified = ifModifiedSince >= (lastModifiedMs / 1000 * 1000);
if (notModified) {
response.setStatus(HttpServletResponse.SC_NOT_MODIFIED);
}
return notModified;
}
public static boolean checkNotModified(HttpServletRequest request, HttpServletResponse response, String etag) {
response.setHeader("ETag", etag);
String ifNoneMatch = request.getHeader("If-None-Match");
boolean notModified = etag.equals(ifNoneMatch);
if (notModified) {
response.setStatus(HttpServletResponse.SC_NOT_MODIFIED);
}
return notModified;
}
public static boolean checkPrecondition(HttpServletRequest request, HttpServletResponse response, long lastModifiedMs) {
response.setDateHeader("Last-Modified", lastModifiedMs);
long ifUnmodifiedSince = request.getDateHeader("If-Unmodified-Since");
return ifUnmodifiedSince == (lastModifiedMs / 1000 * 1000);
}
public static boolean checkPrecondition(HttpServletRequest request, HttpServletResponse response, String etag) {
response.setHeader("ETag", etag);
String ifMatch = request.getHeader("If-Match");
return etag.equals(ifMatch);
}
public static UserPrincipal getUserPrincipal(HttpServletRequest request) {
Principal principal = request.getUserPrincipal();
if (principal == null) {
return null;
}
if (!(principal instanceof UserPrincipal)) {
throw new IllegalStateException("request.getUserPrincipal() must be UserPrincipal.");
}
return (UserPrincipal) principal;
}
public static void setWWWAuthenticate(HttpServletResponse response, String authType, String realmName) {
response.setHeader("WWW-Authenticate", authType + " realm=\"" + realmName + "\"");
response.setStatus(HttpServletResponse.SC_UNAUTHORIZED);
}
public static Object removeAttribute(HttpServletRequest request, String attribute) {
Object obj = request.getAttribute(attribute);
if (obj != null) {
request.removeAttribute(attribute);
}
return obj;
}
public static String getInitParam(FilterConfig config, String paramName, String defaultValue) {
String paramVal = StringUtils.trimToNull(config.getInitParameter(paramName));
return (paramVal != null) ? paramVal : defaultValue;
}
public static String getInitParam(ServletConfig config, String paramName, String defaultValue) {
String paramVal = StringUtils.trimToNull(config.getInitParameter(paramName));
return (paramVal != null) ? paramVal : defaultValue;
}
public static String getInitParam(ServletContext sc, String paramName, String defaultValue) {
String paramVal = StringUtils.trimToNull(sc.getInitParameter(paramName));
return (paramVal != null) ? paramVal : defaultValue;
}
public static RequestContext getRequestContext(HttpServletRequest request) {
RequestContext obj = (RequestContext) request.getAttribute(RequestContext.REQUEST_ATTRIBUTE_ID);
if (obj == null) {
throw new IllegalStateException("requestContext is null.");
}
return obj;
}
public static ModelState getModelState(HttpServletRequest request) {
ModelState obj = (ModelState) request.getAttribute(ModelState.REQUEST_ATTRIBUTE_ID);
if (obj == null) {
obj = new ModelState();
request.setAttribute(ModelState.REQUEST_ATTRIBUTE_ID, obj);
}
return obj;
}
public static Messages getMessages(HttpServletRequest request) {
Messages obj = (Messages) request.getAttribute(Messages.REQUEST_ATTRIBUTE_ID);
if (obj == null) {
obj = new Messages();
request.setAttribute(Messages.REQUEST_ATTRIBUTE_ID, obj);
}
return obj;
}
public static TempData getTempData(HttpServletRequest request) {
TempData obj = (TempData) request.getAttribute(TempData.REQUEST_ATTRIBUTE_ID);
if (obj == null) {
obj = new TempData();
request.setAttribute(TempData.REQUEST_ATTRIBUTE_ID, obj);
}
return obj;
}
public static ViewBag getViewBag(HttpServletRequest request) {
ViewBag obj = (ViewBag) request.getAttribute(ViewBag.REQUEST_ATTRIBUTE_ID);
if (obj == null) {
obj = new ViewBag();
request.setAttribute(ViewBag.REQUEST_ATTRIBUTE_ID, obj);
}
return obj;
}
public static PrefCookie getPrefCookie(HttpServletRequest request) {
return (PrefCookie) request.getAttribute(PrefCookie.REQUEST_ATTRIBUTE_ID);
}
public static Resources getResources(HttpServletRequest request) {
return ServletUtils.getRequestContext(request).getResources();
}
public static FormatProvider getFormatProvider(HttpServletRequest request) {
return ServletUtils.getRequestContext(request).getFormatProvider();
}
public static void addError(HttpServletRequest request, String fieldName, String msgKey) {
ServletUtils.getModelState(request).addError(fieldName, getResources(request).get(msgKey));
}
public static void addError(HttpServletRequest request, String fieldName, String msgKey, Map msgParams) {
ServletUtils.getModelState(request).addError(fieldName, getResources(request).get(msgKey, msgParams));
}
public static T getAppScoped(HttpServletRequest request, Class beanType) {
return getAppScoped(request.getServletContext(), beanType);
}
public static T getAppScoped(ServletContext sc, Class beanType) {
T impl = ObjectUtils.cast(sc.getAttribute(beanType.getName()));
if (impl == null) {
synchronized (getMutex(sc)) {
if ((impl = ObjectUtils.cast(sc.getAttribute(beanType.getName()))) == null) {
impl = CDI.current().select(beanType).get();
sc.setAttribute(beanType.getName(), impl);
}
}
}
return impl;
}
public static boolean loadProps(ServletContext sc, String resourcePath, Properties props) throws IOException {
InputStream is = sc.getResourceAsStream(resourcePath);
if (is == null) {
return false;
}
try {
props.load(is);
return true;
} finally {
IOUtils.closeQuietly(is);
}
}
public static boolean loadProps(ServletContext sc, String resourcePath, PropertyConfig config) throws IOException {
InputStream is = sc.getResourceAsStream(resourcePath);
if (is == null) {
return false;
}
try {
config.load(is);
return true;
} finally {
IOUtils.closeQuietly(is);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy