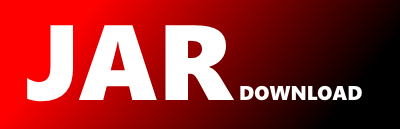
com.aragost.javahg.internals.Utils Maven / Gradle / Ivy
/*
* #%L
* JavaHg
* %%
* Copyright (C) 2011 aragost Trifork ag
* %%
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
* #L%
*/
package com.aragost.javahg.internals;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.Reader;
import java.lang.reflect.Array;
import java.nio.charset.Charset;
import java.nio.charset.CharsetDecoder;
import org.joda.time.DateTime;
import org.joda.time.DateTimeZone;
import com.google.common.io.CharStreams;
public class Utils {
public static Charset getUtf8Charset() {
return Charset.forName("UTF-8");
}
/**
* Read four bytes from the stream, and return the integer
* represented by these bytes in big endian. If EOF is reached
* then -1 is returned.
*
* @param stream
* the input stream
* @return the decoded integer.
* @throws IOException
*/
public static int readBigEndian(InputStream stream) throws IOException {
byte b0 = (byte) stream.read();
byte b1 = (byte) stream.read();
byte b2 = (byte) stream.read();
int b3 = stream.read();
if (b3 == -1) {
return -1;
}
return (b0 << 24) + ((b1 & 0xFF) << 16) + ((b2 & 0xFF) << 8) + ((byte) b3 & 0xFF);
}
/**
* Write 4 bytes representing the specified integer in big-endian.
*
* @param n
* the integer
* @param stream
* the output stream
* @throws IOException
*/
public static void writeBigEndian(int n, OutputStream stream) throws IOException {
byte[] bytes = new byte[] { (byte) (n >>> 24), (byte) (n >>> 16), (byte) (n >>> 8), (byte) n };
stream.write(bytes);
}
public static String formatDate(DateTime date) {
int offset = date.getZone().getOffset(date) / 1000;
long unixTimestamp = date.getMillis() / 1000;
return "" + unixTimestamp + " " + offset;
}
public static DateTime parseDate(String string) {
String[] parts = string.split(" ");
if (parts.length != 2) {
throw new IllegalArgumentException("Wrong date format: " + string);
}
long millis = 1000L * Long.valueOf(parts[0]);
int timezoneOffset = 1000 * Integer.valueOf(parts[1]);
return new DateTime(millis, DateTimeZone.forOffsetMillis(timezoneOffset));
}
public static void consumeAll(InputStream stream) throws IOException {
while (true) {
if (stream.read() == -1) {
break;
}
}
}
public static void consumeAll(Reader stream) throws IOException {
while (true) {
if (stream.read() == -1) {
break;
}
}
}
/**
* Read everything from the stream and return it as a String
*
* @param in
* the input stream
* @param decoder
* the {@link CharsetDecoder} encapsulating the policy
* of handling errors while decoding.
* @return the decoded String.
*/
public static String readStream(InputStream in, CharsetDecoder decoder) {
try {
return CharStreams.toString(new InputStreamReader(in, decoder));
} catch (IOException e) {
throw new RuntimeIOException(e);
}
}
/**
* Insert an element as the first to an array. The input array is
* not changed, instead a copy is returned.
*
* @param
* @param first
* element to insert at position 0 in the result.
* @param rest
* elements to insert at position 1 to
* {@code rest.length}.
* @return the unshifted array of length {@code 1 + rest.length}.
*/
public static T[] arrayUnshift(T first, T[] rest) {
@SuppressWarnings("unchecked")
T[] result = (T[]) Array.newInstance(first.getClass(), 1 + rest.length);
result[0] = first;
System.arraycopy(rest, 0, result, 1, rest.length);
return result;
}
/**
* Concatenate two arrays. The input arrays are copied into the
* output array.
*
* @param
* @param a
* @param b
* @return the concatenated array of length
* {@code a.length + b.length}.
*/
public static T[] arrayConcat(T[] a, T[] b) {
@SuppressWarnings("unchecked")
T[] result = (T[]) Array.newInstance(a.getClass().getComponentType(), a.length + b.length);
System.arraycopy(a, 0, result, 0, a.length);
System.arraycopy(b, 0, result, a.length, b.length);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy