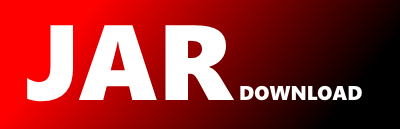
com.aragost.javahg.merge.BackoutConflictResolvingContext Maven / Gradle / Ivy
package com.aragost.javahg.merge;
import java.io.IOException;
import com.aragost.javahg.internals.AbstractCommand;
import com.aragost.javahg.internals.HgInputStream;
import com.aragost.javahg.internals.UnexpectedCommandOutputException;
public class BackoutConflictResolvingContext extends ConflictResolvingContext {
private static final byte[] MERGING_WITH_CHANGESET = "merging with changeset ".getBytes();
private static final byte[] BACKS_OUT_CHANGESET = " backs out changeset ".getBytes();
private static final byte[] CHANGESET = "changeset".getBytes();
private static final byte[] REVERTING = "reverting".getBytes();
private static final byte[] CREATED_NEW_HEAD = "created new head".getBytes();
private final boolean merge;
public BackoutConflictResolvingContext(AbstractCommand command, boolean merge) {
super(command);
this.merge = merge;
}
/**
* Example:
*
* $ hg backout --merge 7:7
* reverting foo.txt
* created new head
* changeset 9:664c3e4cbb2b backs out changeset 7:3f5bacea93aa
* merging with changeset 9:664c3e4cbb2b
* merging foo.txt
* warning: conflicts during merge.
* merging foo.txt incomplete! (edit conflicts, then use 'hg resolve --mark')
* 0 files updated, 0 files merged, 0 files removed, 1 files unresolved
* use 'hg resolve' to retry unresolved file merges or 'hg update -C .' to abandon
*
*
* @see com.aragost.javahg.merge.ConflictResolvingContext#processStream(com.aragost.javahg.internals.HgInputStream,
* boolean)
*/
@Override
public void processStream(HgInputStream in, boolean whenUnknowReturn)
throws IOException {
String createdChangeset = null;
while (!in.isEof()) {
if (in.match(REVERTING)) {
in.upTo('\n');
} else if (in.match(CREATED_NEW_HEAD)) {
in.upTo('\n');
} else if (in.match(CHANGESET)) {
createdChangeset = in.textUpTo(BACKS_OUT_CHANGESET);
in.upTo('\n');
} else if (in.match(MERGING_WITH_CHANGESET)) {
in.upTo('\n');
} else {
break;
}
}
if (createdChangeset == null && merge) {
if (whenUnknowReturn) {
return;
}
throw new UnexpectedCommandOutputException(this.getCommand(), null);
}
super.processStream(in, whenUnknowReturn);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy