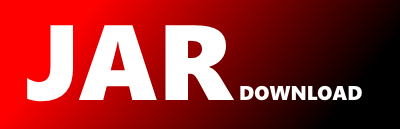
com.aragost.javahg.WorkingCopy Maven / Gradle / Ivy
package com.aragost.javahg;
import java.io.IOException;
import java.util.List;
import com.aragost.javahg.commands.AddCommand;
import com.aragost.javahg.commands.BranchCommand;
import com.aragost.javahg.commands.MergeCommand;
import com.aragost.javahg.commands.ParentsCommand;
import com.aragost.javahg.commands.RemoveCommand;
import com.aragost.javahg.commands.StatusCommand;
import com.aragost.javahg.commands.StatusResult;
import com.aragost.javahg.merge.MergeContext;
/**
* Represents working copy for a Repository.
*
* Warning: The instance cache the information and will not see
* changes to the working copy. Create a new instance if you need the
* most current state.
*
*/
public class WorkingCopy {
private Repository repository;
private boolean parentsRetrieved = false;
private Changeset parent1;
private Changeset parent2;
private String branchName;
WorkingCopy(Repository repository) {
this.repository = repository;
}
public Changeset getParent1() {
ensureParentsRetrieved();
return this.parent1;
}
public Changeset getParent2() {
ensureParentsRetrieved();
return this.parent2;
}
/**
* @return branch name for working copy
*/
public synchronized String getBranchName() {
if (this.branchName == null) {
this.branchName = BranchCommand.on(this.repository).get();
}
return this.branchName;
}
/**
* Set the branch name of working copy
*
* @param branchName
*/
public synchronized void setBranchName(String branchName) {
BranchCommand.on(this.repository).set(branchName);
this.branchName = branchName;
}
public void remove(String... files) {
RemoveCommand.on(this.repository).execute(files);
}
public void add(String... files) {
AddCommand.on(this.repository).execute(files);
}
/**
* Merge the working copy with the specified changeset
*
* @param remote
* @return a MergeContext
* @throws IOException
*/
public MergeContext merge(Changeset remote) throws IOException {
return MergeCommand.on(this.repository).rev(remote.getNode()).execute();
}
/**
*
* @return status for working copy relative to first parent
*/
public StatusResult status() {
return StatusCommand.on(this.repository).execute();
}
/**
*
* @return status for working copy relative to second parent
*/
public StatusResult parent2Status() {
if (getParent2() == null) {
return null;
}
return StatusCommand.on(this.repository).rev(getParent2().getNode()).execute();
}
private synchronized void ensureParentsRetrieved() {
if (!this.parentsRetrieved) {
retrieveParents();
this.parentsRetrieved = true;
}
}
private void retrieveParents() {
List parents = ParentsCommand.on(this.repository).execute();
switch (parents.size()) {
// Fall though on purpose
case 2:
this.parent2 = parents.get(1);
case 1:
this.parent1 = parents.get(0);
case 0:
break;
default:
throw new RuntimeException("More that 2 parents from parents command");
}
}
}