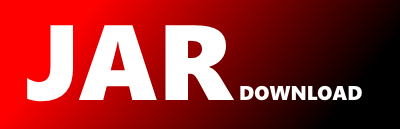
com.araguacaima.specification.util.SpecificationMapBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of composite-specification Show documentation
Show all versions of composite-specification Show documentation
The Composite Specification Pattern is a particular implementation of the "Specification" design
pattern, whereby business logic can be recombined by stringing business logic together with Boolean logic.
The newest version!
/*
* Copyright 2020 araguacaima
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.araguacaima.specification.util;
import com.araguacaima.specification.common.ReflectionUtils;
import com.araguacaima.specification.common.StringUtils;
import com.araguacaima.specification.interpreter.logical.LogicalEvaluator;
import org.apache.commons.collections4.MapUtils;
import org.apache.commons.collections4.Predicate;
import java.io.IOException;
import java.util.*;
@SuppressWarnings("WeakerAccess")
public class SpecificationMapBuilder {
private final Map instancesMap = new HashMap<>();
private final String propertiesFile = "specification.properties";
public SpecificationMapBuilder() {
}
public SpecificationMap getInstance(Class> clazz)
throws IOException {
return getInstance(clazz, false);
}
public SpecificationMap getInstance(Class> clazz, boolean forceReplace)
throws IOException {
ClassLoader classLoader = clazz.getClassLoader();
Properties prop = new Properties();
prop.load(classLoader.getResourceAsStream(propertiesFile));
return buildInstance(prop, clazz, forceReplace, clazz.getClassLoader());
}
private SpecificationMap getInstance(Properties properties, Class> clazz) {
return buildInstance(properties, clazz, false, clazz.getClassLoader());
}
public SpecificationMap getInstance(Map map, Class> clazz) {
return buildInstance(MapUtils.toProperties(map), clazz, false, clazz.getClassLoader());
}
public SpecificationMap getInstance(Map map, Class> clazz, ClassLoader classLoader) {
return buildInstance(MapUtils.toProperties(map), clazz, false, classLoader);
}
public SpecificationMap getInstance(Map map, Class> clazz, boolean replace) {
return buildInstance(MapUtils.toProperties(map), clazz, replace, clazz.getClassLoader());
}
private SpecificationMap buildInstance(Properties properties,
Class> clazz,
boolean replace,
ClassLoader classLoader) {
SpecificationMap instance;
Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy