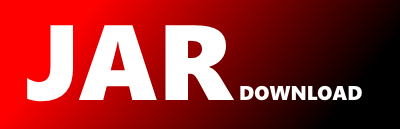
com.arakelian.docker.junit.model.ImmutableDockerConfig Maven / Gradle / Ivy
package com.arakelian.docker.junit.model;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link DockerConfig}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDockerConfig.builder()}.
*/
@Generated(from = "DockerConfig", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableDockerConfig implements DockerConfig {
private final ImmutableList containerConfigurer;
private final ImmutableList hostConfigurer;
private final String image;
private final String name;
private final String[] ports;
private final ImmutableList startedListener;
private final boolean allowRunningBetweenUnitTests;
private final boolean alwaysPullLatestImage;
private final boolean alwaysRemoveContainer;
private ImmutableDockerConfig(ImmutableDockerConfig.Builder builder) {
this.image = builder.image;
this.name = builder.name;
this.ports = builder.ports;
if (builder.containerConfigurerIsSet()) {
initShim.containerConfigurer(builder.containerConfigurer.build());
}
if (builder.hostConfigurerIsSet()) {
initShim.hostConfigurer(builder.hostConfigurer.build());
}
if (builder.startedListenerIsSet()) {
initShim.startedListener(builder.startedListener.build());
}
if (builder.allowRunningBetweenUnitTestsIsSet()) {
initShim.allowRunningBetweenUnitTests(builder.allowRunningBetweenUnitTests);
}
if (builder.alwaysPullLatestImageIsSet()) {
initShim.alwaysPullLatestImage(builder.alwaysPullLatestImage);
}
if (builder.alwaysRemoveContainerIsSet()) {
initShim.alwaysRemoveContainer(builder.alwaysRemoveContainer);
}
this.containerConfigurer = initShim.getContainerConfigurer();
this.hostConfigurer = initShim.getHostConfigurer();
this.startedListener = initShim.getStartedListener();
this.allowRunningBetweenUnitTests = initShim.isAllowRunningBetweenUnitTests();
this.alwaysPullLatestImage = initShim.isAlwaysPullLatestImage();
this.alwaysRemoveContainer = initShim.isAlwaysRemoveContainer();
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "DockerConfig", generator = "Immutables")
private final class InitShim {
private byte containerConfigurerBuildStage = STAGE_UNINITIALIZED;
private ImmutableList containerConfigurer;
ImmutableList getContainerConfigurer() {
if (containerConfigurerBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (containerConfigurerBuildStage == STAGE_UNINITIALIZED) {
containerConfigurerBuildStage = STAGE_INITIALIZING;
this.containerConfigurer = ImmutableList.copyOf(getContainerConfigurerInitialize());
containerConfigurerBuildStage = STAGE_INITIALIZED;
}
return this.containerConfigurer;
}
void containerConfigurer(ImmutableList containerConfigurer) {
this.containerConfigurer = containerConfigurer;
containerConfigurerBuildStage = STAGE_INITIALIZED;
}
private byte hostConfigurerBuildStage = STAGE_UNINITIALIZED;
private ImmutableList hostConfigurer;
ImmutableList getHostConfigurer() {
if (hostConfigurerBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (hostConfigurerBuildStage == STAGE_UNINITIALIZED) {
hostConfigurerBuildStage = STAGE_INITIALIZING;
this.hostConfigurer = ImmutableList.copyOf(getHostConfigurerInitialize());
hostConfigurerBuildStage = STAGE_INITIALIZED;
}
return this.hostConfigurer;
}
void hostConfigurer(ImmutableList hostConfigurer) {
this.hostConfigurer = hostConfigurer;
hostConfigurerBuildStage = STAGE_INITIALIZED;
}
private byte startedListenerBuildStage = STAGE_UNINITIALIZED;
private ImmutableList startedListener;
ImmutableList getStartedListener() {
if (startedListenerBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (startedListenerBuildStage == STAGE_UNINITIALIZED) {
startedListenerBuildStage = STAGE_INITIALIZING;
this.startedListener = ImmutableList.copyOf(getStartedListenerInitialize());
startedListenerBuildStage = STAGE_INITIALIZED;
}
return this.startedListener;
}
void startedListener(ImmutableList startedListener) {
this.startedListener = startedListener;
startedListenerBuildStage = STAGE_INITIALIZED;
}
private byte allowRunningBetweenUnitTestsBuildStage = STAGE_UNINITIALIZED;
private boolean allowRunningBetweenUnitTests;
boolean isAllowRunningBetweenUnitTests() {
if (allowRunningBetweenUnitTestsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (allowRunningBetweenUnitTestsBuildStage == STAGE_UNINITIALIZED) {
allowRunningBetweenUnitTestsBuildStage = STAGE_INITIALIZING;
this.allowRunningBetweenUnitTests = isAllowRunningBetweenUnitTestsInitialize();
allowRunningBetweenUnitTestsBuildStage = STAGE_INITIALIZED;
}
return this.allowRunningBetweenUnitTests;
}
void allowRunningBetweenUnitTests(boolean allowRunningBetweenUnitTests) {
this.allowRunningBetweenUnitTests = allowRunningBetweenUnitTests;
allowRunningBetweenUnitTestsBuildStage = STAGE_INITIALIZED;
}
private byte alwaysPullLatestImageBuildStage = STAGE_UNINITIALIZED;
private boolean alwaysPullLatestImage;
boolean isAlwaysPullLatestImage() {
if (alwaysPullLatestImageBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (alwaysPullLatestImageBuildStage == STAGE_UNINITIALIZED) {
alwaysPullLatestImageBuildStage = STAGE_INITIALIZING;
this.alwaysPullLatestImage = isAlwaysPullLatestImageInitialize();
alwaysPullLatestImageBuildStage = STAGE_INITIALIZED;
}
return this.alwaysPullLatestImage;
}
void alwaysPullLatestImage(boolean alwaysPullLatestImage) {
this.alwaysPullLatestImage = alwaysPullLatestImage;
alwaysPullLatestImageBuildStage = STAGE_INITIALIZED;
}
private byte alwaysRemoveContainerBuildStage = STAGE_UNINITIALIZED;
private boolean alwaysRemoveContainer;
boolean isAlwaysRemoveContainer() {
if (alwaysRemoveContainerBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (alwaysRemoveContainerBuildStage == STAGE_UNINITIALIZED) {
alwaysRemoveContainerBuildStage = STAGE_INITIALIZING;
this.alwaysRemoveContainer = isAlwaysRemoveContainerInitialize();
alwaysRemoveContainerBuildStage = STAGE_INITIALIZED;
}
return this.alwaysRemoveContainer;
}
void alwaysRemoveContainer(boolean alwaysRemoveContainer) {
this.alwaysRemoveContainer = alwaysRemoveContainer;
alwaysRemoveContainerBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (containerConfigurerBuildStage == STAGE_INITIALIZING) attributes.add("containerConfigurer");
if (hostConfigurerBuildStage == STAGE_INITIALIZING) attributes.add("hostConfigurer");
if (startedListenerBuildStage == STAGE_INITIALIZING) attributes.add("startedListener");
if (allowRunningBetweenUnitTestsBuildStage == STAGE_INITIALIZING) attributes.add("allowRunningBetweenUnitTests");
if (alwaysPullLatestImageBuildStage == STAGE_INITIALIZING) attributes.add("alwaysPullLatestImage");
if (alwaysRemoveContainerBuildStage == STAGE_INITIALIZING) attributes.add("alwaysRemoveContainer");
return "Cannot build DockerConfig, attribute initializers form cycle " + attributes;
}
}
private List getContainerConfigurerInitialize() {
return DockerConfig.super.getContainerConfigurer();
}
private List getHostConfigurerInitialize() {
return DockerConfig.super.getHostConfigurer();
}
private List getStartedListenerInitialize() {
return DockerConfig.super.getStartedListener();
}
private boolean isAllowRunningBetweenUnitTestsInitialize() {
return DockerConfig.super.isAllowRunningBetweenUnitTests();
}
private boolean isAlwaysPullLatestImageInitialize() {
return DockerConfig.super.isAlwaysPullLatestImage();
}
private boolean isAlwaysRemoveContainerInitialize() {
return DockerConfig.super.isAlwaysRemoveContainer();
}
/**
* @return The value of the {@code containerConfigurer} attribute
*/
@Override
public ImmutableList getContainerConfigurer() {
InitShim shim = this.initShim;
return shim != null
? shim.getContainerConfigurer()
: this.containerConfigurer;
}
/**
* @return The value of the {@code hostConfigurer} attribute
*/
@Override
public ImmutableList getHostConfigurer() {
InitShim shim = this.initShim;
return shim != null
? shim.getHostConfigurer()
: this.hostConfigurer;
}
/**
* Returns the name of the docker image.
* @return name of the docker image
*/
@Override
public String getImage() {
return image;
}
/**
* Returns the container name (as would be displayed in "NAMES" column of "docker ps" command).
* @return the container name.
*/
@Override
public String getName() {
return name;
}
/**
* Returns the list of ports that this container will make public.
* @return list of ports that this container will make public.
*/
@Override
public String[] getPorts() {
return ports.clone();
}
/**
* Returns a list of listeners which are called when the container is started.
*
* @return list of listeners which are called when the container is started
*/
@Override
public ImmutableList getStartedListener() {
InitShim shim = this.initShim;
return shim != null
? shim.getStartedListener()
: this.startedListener;
}
/**
* Returns true if container is allowed to continue running between separate JUnit tests.
*
* Note that all containers are automatically stopped when the JVM is exited (e.g. when Gradle
* tests complete, or when JUnit tests complete inside Eclipse). This is a performance
* enhancement that allows individual unit tests to complete much faster.
*
* @return true if container is allowed to continue running between separate JUnit tests
*/
@Override
public boolean isAllowRunningBetweenUnitTests() {
InitShim shim = this.initShim;
return shim != null
? shim.isAllowRunningBetweenUnitTests()
: this.allowRunningBetweenUnitTests;
}
/**
* Returns true if we should always pull the latest image, even if we already have a copy
* locally.
* @return true if we should always pull the latest image
*/
@Override
public boolean isAlwaysPullLatestImage() {
InitShim shim = this.initShim;
return shim != null
? shim.isAlwaysPullLatestImage()
: this.alwaysPullLatestImage;
}
/**
* Returns true if container should always be removed between executions.
*
* @return true if container should always be removed between executions.
*/
@Override
public boolean isAlwaysRemoveContainer() {
InitShim shim = this.initShim;
return shim != null
? shim.isAlwaysRemoveContainer()
: this.alwaysRemoveContainer;
}
/**
* This instance is equal to all instances of {@code ImmutableDockerConfig} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableDockerConfig
&& equalTo((ImmutableDockerConfig) another);
}
private boolean equalTo(ImmutableDockerConfig another) {
return name.equals(another.name);
}
/**
* Computes a hash code from attributes: {@code name}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + name.hashCode();
return h;
}
/**
* Prints the immutable value {@code DockerConfig} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("DockerConfig")
.omitNullValues()
.add("name", name)
.toString();
}
/**
* Creates a builder for {@link ImmutableDockerConfig ImmutableDockerConfig}.
*
* ImmutableDockerConfig.builder()
* .addContainerConfigurer|addAllContainerConfigurer(com.arakelian.docker.junit.model.ContainerConfigurer) // {@link DockerConfig#getContainerConfigurer() containerConfigurer} elements
* .addHostConfigurer|addAllHostConfigurer(com.arakelian.docker.junit.model.HostConfigurer) // {@link DockerConfig#getHostConfigurer() hostConfigurer} elements
* .image(String) // required {@link DockerConfig#getImage() image}
* .name(String) // required {@link DockerConfig#getName() name}
* .ports(String) // required {@link DockerConfig#getPorts() ports}
* .addStartedListener|addAllStartedListener(com.arakelian.docker.junit.model.StartedListener) // {@link DockerConfig#getStartedListener() startedListener} elements
* .allowRunningBetweenUnitTests(boolean) // optional {@link DockerConfig#isAllowRunningBetweenUnitTests() allowRunningBetweenUnitTests}
* .alwaysPullLatestImage(boolean) // optional {@link DockerConfig#isAlwaysPullLatestImage() alwaysPullLatestImage}
* .alwaysRemoveContainer(boolean) // optional {@link DockerConfig#isAlwaysRemoveContainer() alwaysRemoveContainer}
* .build();
*
* @return A new ImmutableDockerConfig builder
*/
public static ImmutableDockerConfig.Builder builder() {
return new ImmutableDockerConfig.Builder();
}
/**
* Builds instances of type {@link ImmutableDockerConfig ImmutableDockerConfig}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "DockerConfig", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_IMAGE = 0x1L;
private static final long INIT_BIT_NAME = 0x2L;
private static final long INIT_BIT_PORTS = 0x4L;
private static final long OPT_BIT_CONTAINER_CONFIGURER = 0x1L;
private static final long OPT_BIT_HOST_CONFIGURER = 0x2L;
private static final long OPT_BIT_STARTED_LISTENER = 0x4L;
private static final long OPT_BIT_ALLOW_RUNNING_BETWEEN_UNIT_TESTS = 0x8L;
private static final long OPT_BIT_ALWAYS_PULL_LATEST_IMAGE = 0x10L;
private static final long OPT_BIT_ALWAYS_REMOVE_CONTAINER = 0x20L;
private long initBits = 0x7L;
private long optBits;
private ImmutableList.Builder containerConfigurer = ImmutableList.builder();
private ImmutableList.Builder hostConfigurer = ImmutableList.builder();
private @Nullable String image;
private @Nullable String name;
private @Nullable String[] ports;
private ImmutableList.Builder startedListener = ImmutableList.builder();
private boolean allowRunningBetweenUnitTests;
private boolean alwaysPullLatestImage;
private boolean alwaysRemoveContainer;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code DockerConfig} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(DockerConfig instance) {
Objects.requireNonNull(instance, "instance");
addAllContainerConfigurer(instance.getContainerConfigurer());
addAllHostConfigurer(instance.getHostConfigurer());
image(instance.getImage());
name(instance.getName());
ports(instance.getPorts());
addAllStartedListener(instance.getStartedListener());
allowRunningBetweenUnitTests(instance.isAllowRunningBetweenUnitTests());
alwaysPullLatestImage(instance.isAlwaysPullLatestImage());
alwaysRemoveContainer(instance.isAlwaysRemoveContainer());
return this;
}
/**
* Adds one element to {@link DockerConfig#getContainerConfigurer() containerConfigurer} list.
* @param element A containerConfigurer element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addContainerConfigurer(ContainerConfigurer element) {
this.containerConfigurer.add(element);
optBits |= OPT_BIT_CONTAINER_CONFIGURER;
return this;
}
/**
* Adds elements to {@link DockerConfig#getContainerConfigurer() containerConfigurer} list.
* @param elements An array of containerConfigurer elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addContainerConfigurer(ContainerConfigurer... elements) {
this.containerConfigurer.add(elements);
optBits |= OPT_BIT_CONTAINER_CONFIGURER;
return this;
}
/**
* Sets or replaces all elements for {@link DockerConfig#getContainerConfigurer() containerConfigurer} list.
* @param elements An iterable of containerConfigurer elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder containerConfigurer(Iterable extends ContainerConfigurer> elements) {
this.containerConfigurer = ImmutableList.builder();
return addAllContainerConfigurer(elements);
}
/**
* Adds elements to {@link DockerConfig#getContainerConfigurer() containerConfigurer} list.
* @param elements An iterable of containerConfigurer elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllContainerConfigurer(Iterable extends ContainerConfigurer> elements) {
this.containerConfigurer.addAll(elements);
optBits |= OPT_BIT_CONTAINER_CONFIGURER;
return this;
}
/**
* Adds one element to {@link DockerConfig#getHostConfigurer() hostConfigurer} list.
* @param element A hostConfigurer element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addHostConfigurer(HostConfigurer element) {
this.hostConfigurer.add(element);
optBits |= OPT_BIT_HOST_CONFIGURER;
return this;
}
/**
* Adds elements to {@link DockerConfig#getHostConfigurer() hostConfigurer} list.
* @param elements An array of hostConfigurer elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addHostConfigurer(HostConfigurer... elements) {
this.hostConfigurer.add(elements);
optBits |= OPT_BIT_HOST_CONFIGURER;
return this;
}
/**
* Sets or replaces all elements for {@link DockerConfig#getHostConfigurer() hostConfigurer} list.
* @param elements An iterable of hostConfigurer elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder hostConfigurer(Iterable extends HostConfigurer> elements) {
this.hostConfigurer = ImmutableList.builder();
return addAllHostConfigurer(elements);
}
/**
* Adds elements to {@link DockerConfig#getHostConfigurer() hostConfigurer} list.
* @param elements An iterable of hostConfigurer elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllHostConfigurer(Iterable extends HostConfigurer> elements) {
this.hostConfigurer.addAll(elements);
optBits |= OPT_BIT_HOST_CONFIGURER;
return this;
}
/**
* Initializes the value for the {@link DockerConfig#getImage() image} attribute.
* @param image The value for image
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder image(String image) {
this.image = Objects.requireNonNull(image, "image");
initBits &= ~INIT_BIT_IMAGE;
return this;
}
/**
* Initializes the value for the {@link DockerConfig#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link DockerConfig#getPorts() ports} attribute.
* @param ports The elements for ports
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder ports(String... ports) {
this.ports = ports.clone();
initBits &= ~INIT_BIT_PORTS;
return this;
}
/**
* Adds one element to {@link DockerConfig#getStartedListener() startedListener} list.
* @param element A startedListener element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addStartedListener(StartedListener element) {
this.startedListener.add(element);
optBits |= OPT_BIT_STARTED_LISTENER;
return this;
}
/**
* Adds elements to {@link DockerConfig#getStartedListener() startedListener} list.
* @param elements An array of startedListener elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addStartedListener(StartedListener... elements) {
this.startedListener.add(elements);
optBits |= OPT_BIT_STARTED_LISTENER;
return this;
}
/**
* Sets or replaces all elements for {@link DockerConfig#getStartedListener() startedListener} list.
* @param elements An iterable of startedListener elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder startedListener(Iterable extends StartedListener> elements) {
this.startedListener = ImmutableList.builder();
return addAllStartedListener(elements);
}
/**
* Adds elements to {@link DockerConfig#getStartedListener() startedListener} list.
* @param elements An iterable of startedListener elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllStartedListener(Iterable extends StartedListener> elements) {
this.startedListener.addAll(elements);
optBits |= OPT_BIT_STARTED_LISTENER;
return this;
}
/**
* Initializes the value for the {@link DockerConfig#isAllowRunningBetweenUnitTests() allowRunningBetweenUnitTests} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link DockerConfig#isAllowRunningBetweenUnitTests() allowRunningBetweenUnitTests}.
* @param allowRunningBetweenUnitTests The value for allowRunningBetweenUnitTests
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder allowRunningBetweenUnitTests(boolean allowRunningBetweenUnitTests) {
this.allowRunningBetweenUnitTests = allowRunningBetweenUnitTests;
optBits |= OPT_BIT_ALLOW_RUNNING_BETWEEN_UNIT_TESTS;
return this;
}
/**
* Initializes the value for the {@link DockerConfig#isAlwaysPullLatestImage() alwaysPullLatestImage} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link DockerConfig#isAlwaysPullLatestImage() alwaysPullLatestImage}.
* @param alwaysPullLatestImage The value for alwaysPullLatestImage
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder alwaysPullLatestImage(boolean alwaysPullLatestImage) {
this.alwaysPullLatestImage = alwaysPullLatestImage;
optBits |= OPT_BIT_ALWAYS_PULL_LATEST_IMAGE;
return this;
}
/**
* Initializes the value for the {@link DockerConfig#isAlwaysRemoveContainer() alwaysRemoveContainer} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link DockerConfig#isAlwaysRemoveContainer() alwaysRemoveContainer}.
* @param alwaysRemoveContainer The value for alwaysRemoveContainer
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder alwaysRemoveContainer(boolean alwaysRemoveContainer) {
this.alwaysRemoveContainer = alwaysRemoveContainer;
optBits |= OPT_BIT_ALWAYS_REMOVE_CONTAINER;
return this;
}
/**
* Builds a new {@link ImmutableDockerConfig ImmutableDockerConfig}.
* @return An immutable instance of DockerConfig
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDockerConfig build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableDockerConfig(this);
}
private boolean containerConfigurerIsSet() {
return (optBits & OPT_BIT_CONTAINER_CONFIGURER) != 0;
}
private boolean hostConfigurerIsSet() {
return (optBits & OPT_BIT_HOST_CONFIGURER) != 0;
}
private boolean startedListenerIsSet() {
return (optBits & OPT_BIT_STARTED_LISTENER) != 0;
}
private boolean allowRunningBetweenUnitTestsIsSet() {
return (optBits & OPT_BIT_ALLOW_RUNNING_BETWEEN_UNIT_TESTS) != 0;
}
private boolean alwaysPullLatestImageIsSet() {
return (optBits & OPT_BIT_ALWAYS_PULL_LATEST_IMAGE) != 0;
}
private boolean alwaysRemoveContainerIsSet() {
return (optBits & OPT_BIT_ALWAYS_REMOVE_CONTAINER) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_IMAGE) != 0) attributes.add("image");
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_PORTS) != 0) attributes.add("ports");
return "Cannot build DockerConfig, some of required attributes are not set " + attributes;
}
}
}