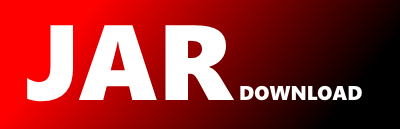
com.arangodb.async.ArangoCollectionAsync Maven / Gradle / Ivy
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb.async;
import com.arangodb.ArangoSerdeAccessor;
import com.arangodb.entity.*;
import com.arangodb.model.*;
import com.arangodb.util.RawData;
import javax.annotation.concurrent.ThreadSafe;
import java.util.Collection;
import java.util.concurrent.CompletableFuture;
/**
* Interface for operations on ArangoDB collection level.
*
* @author Mark Vollmary
* @see Collection API Documentation
* @see Documents API Documentation
*/
@ThreadSafe
public interface ArangoCollectionAsync extends ArangoSerdeAccessor {
/**
* The the handler of the database the collection is within
*
* @return database handler
*/
ArangoDatabaseAsync db();
/**
* The name of the collection
*
* @return collection name
*/
String name();
/**
* Creates a new document from the given document, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @return information about the document
* @see API
* Documentation
*/
CompletableFuture> insertDocument(final Object value);
/**
* Creates a new document from the given document, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @return information about the document
* @see API
* Documentation
*/
CompletableFuture> insertDocument(final T value, final DocumentCreateOptions options);
/**
* Creates a new document from the given document, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @param type Deserialization target type for the returned documents.
* @return information about the document
* @see API
* Documentation
*/
CompletableFuture> insertDocument(final T value, final DocumentCreateOptions options,
Class type);
/**
* Creates new documents from the given documents, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param values Raw data representing a collection of documents
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> insertDocuments(final RawData values);
/**
* Creates new documents from the given documents, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param values Raw data representing a collection of documents
* @param options Additional options
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> insertDocuments(
final RawData values,
final DocumentCreateOptions options);
/**
* Creates new documents from the given documents, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> insertDocuments(final Collection> values);
/**
* Creates new documents from the given documents, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> insertDocuments(
final Collection values,
final DocumentCreateOptions options);
/**
* Creates new documents from the given documents, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @param type Deserialization target type for the returned documents.
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> insertDocuments(
final Collection values,
final DocumentCreateOptions options,
final Class type);
/**
* Imports documents
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @return information about the import
*/
CompletableFuture importDocuments(final Collection> values);
/**
* Imports documents
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options, can be null
* @return information about the import
*/
CompletableFuture importDocuments(
final Collection> values,
final DocumentImportOptions options);
/**
* Imports documents
*
* @param values Raw data representing a collection of documents
* @return information about the import
*/
CompletableFuture importDocuments(final RawData values);
/**
* Imports documents
*
* @param values Raw data representing a collection of documents
* @param options Additional options, can be null
* @return information about the import
*/
CompletableFuture importDocuments(final RawData values, final DocumentImportOptions options);
/**
* Reads a single document
*
* @param key The key of the document
* @param type The type of the document (POJO or {@link com.arangodb.util.RawData})
* @return the document identified by the key
* @see API
* Documentation
*/
CompletableFuture getDocument(final String key, final Class type);
/**
* Reads a single document
*
* @param key The key of the document
* @param type The type of the document (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options, can be null
* @return the document identified by the key
* @see API
* Documentation
*/
CompletableFuture getDocument(final String key, final Class type, final DocumentReadOptions options);
/**
* Reads multiple documents
*
* @param keys The keys of the documents
* @param type The type of the documents (POJO or {@link com.arangodb.util.RawData})
* @return the documents and possible errors
*/
CompletableFuture> getDocuments(final Collection keys, final Class type);
/**
* Reads multiple documents
*
* @param keys The keys of the documents
* @param type The type of the documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options, can be null
* @return the documents and possible errors
*/
CompletableFuture> getDocuments(
final Collection keys,
final Class type,
DocumentReadOptions options);
/**
* Replaces the document with key with the one in the body, provided there is such a document and no precondition is
* violated
*
* @param key The key of the document
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @return information about the document
* @see
* API
* Documentation
*/
CompletableFuture> replaceDocument(final String key, final Object value);
/**
* Replaces the document with key with the one in the body, provided there is such a document and no precondition is
* violated
*
* @param key The key of the document
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @return information about the document
* @see
* API
* Documentation
*/
CompletableFuture> replaceDocument(
final String key,
final T value,
final DocumentReplaceOptions options);
/**
* Replaces the document with key with the one in the body, provided there is such a document and no precondition is
* violated
*
* @param key The key of the document
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @param type Deserialization target type for the returned documents.
* @return information about the document
* @see
* API
* Documentation
*/
CompletableFuture> replaceDocument(
final String key,
final T value,
final DocumentReplaceOptions options,
final Class type);
/**
* Replaces multiple documents in the specified collection with the ones in the values, the replaced documents are
* specified by the _key attributes in the documents in values.
*
* @param values Raw data representing a collection of documents
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> replaceDocuments(final RawData values);
/**
* Replaces multiple documents in the specified collection with the ones in the values, the replaced documents are
* specified by the _key attributes in the documents in values.
*
* @param values Raw data representing a collection of documents
* @param options Additional options
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> replaceDocuments(
final RawData values,
final DocumentReplaceOptions options);
/**
* Replaces multiple documents in the specified collection with the ones in the values, the replaced documents are
* specified by the _key attributes in the documents in values.
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> replaceDocuments(final Collection> values);
/**
* Replaces multiple documents in the specified collection with the ones in the values, the replaced documents are
* specified by the _key attributes in the documents in values.
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> replaceDocuments(
final Collection values,
final DocumentReplaceOptions options);
/**
* Replaces multiple documents in the specified collection with the ones in the values, the replaced documents are
* specified by the _key attributes in the documents in values.
*
* @param values A List of documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @param type Deserialization target type for the returned documents.
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> replaceDocuments(
final Collection values,
final DocumentReplaceOptions options,
final Class type);
/**
* Partially updates the document identified by document-key. The value must contain a document with the attributes
* to patch (the patch document). All attributes from the patch document will be added to the existing document if
* they do not yet exist, and overwritten in the existing document if they do exist there.
*
* @param key The key of the document
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @return information about the document
* @see API
* Documentation
*/
CompletableFuture> updateDocument(final String key, final Object value);
/**
* Partially updates the document identified by document-key. The value must contain a document with the attributes
* to patch (the patch document). All attributes from the patch document will be added to the existing document if
* they do not yet exist, and overwritten in the existing document if they do exist there.
*
* @param key The key of the document
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @return information about the document
* @see API
* Documentation
*/
CompletableFuture> updateDocument(
final String key,
final T value,
final DocumentUpdateOptions options);
/**
* Partially updates the document identified by document-key. The value must contain a document with the attributes
* to patch (the patch document). All attributes from the patch document will be added to the existing document if
* they do not yet exist, and overwritten in the existing document if they do exist there.
*
* @param key The key of the document
* @param value A representation of a single document (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @param returnType Type of the returned newDocument and/or oldDocument
* @return information about the document
* @see API
* Documentation
*/
CompletableFuture> updateDocument(
final String key,
final Object value,
final DocumentUpdateOptions options,
final Class returnType);
/**
* Partially updates documents, the documents to update are specified by the _key attributes in the objects on
* values. Vales must contain a list of document updates with the attributes to patch (the patch documents). All
* attributes from the patch documents will be added to the existing documents if they do not yet exist, and
* overwritten in the existing documents if they do exist there.
*
* @param values Raw data representing a collection of documents
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> updateDocuments(final RawData values);
/**
* Partially updates documents, the documents to update are specified by the _key attributes in the objects on
* values. Vales must contain a list of document updates with the attributes to patch (the patch documents). All
* attributes from the patch documents will be added to the existing documents if they do not yet exist, and
* overwritten in the existing documents if they do exist there.
*
* @param values Raw data representing a collection of documents
* @param options Additional options
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> updateDocuments(
final RawData values,
final DocumentUpdateOptions options);
/**
* Partially updates documents, the documents to update are specified by the _key attributes in the objects on
* values. Vales must contain a list of document updates with the attributes to patch (the patch documents). All
* attributes from the patch documents will be added to the existing documents if they do not yet exist, and
* overwritten in the existing documents if they do exist there.
*
* @param values A list of documents (POJO or {@link com.arangodb.util.RawData})
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> updateDocuments(final Collection> values);
/**
* Partially updates documents, the documents to update are specified by the _key attributes in the objects on
* values. Vales must contain a list of document updates with the attributes to patch (the patch documents). All
* attributes from the patch documents will be added to the existing documents if they do not yet exist, and
* overwritten in the existing documents if they do exist there.
*
* @param values A list of documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> updateDocuments(
final Collection values,
final DocumentUpdateOptions options);
/**
* Partially updates documents, the documents to update are specified by the _key attributes in the objects on
* values. Vales must contain a list of document updates with the attributes to patch (the patch documents). All
* attributes from the patch documents will be added to the existing documents if they do not yet exist, and
* overwritten in the existing documents if they do exist there.
*
* @param values A list of documents (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options
* @param returnType Type of the returned newDocument and/or oldDocument
* @return information about the documents
* @see
* API
* Documentation
*/
CompletableFuture>> updateDocuments(
final Collection> values,
final DocumentUpdateOptions options,
final Class returnType);
/**
* Removes a document
*
* @param key The key of the document
* @return information about the document
* @see
* API
* Documentation
*/
CompletableFuture> deleteDocument(final String key);
/**
* Removes a document
*
* @param key The key of the document
* @param options Additional options
* @return information about the document
* @see
* API
* Documentation
*/
CompletableFuture> deleteDocument(
final String key,
final DocumentDeleteOptions options);
/**
* Removes a document
*
* @param key The key of the document
* @param type Deserialization target type for the returned documents.
* @param options Additional options
* @return information about the document
* @see
* API
* Documentation
*/
CompletableFuture> deleteDocument(
final String key,
final DocumentDeleteOptions options,
final Class type);
/**
* Removes multiple document
*
* @param values Raw data representing the keys of the documents or the documents themselves
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> deleteDocuments(final RawData values);
/**
* Removes multiple document
*
* @param values Raw data representing the keys of the documents or the documents themselves
* @param options Additional options
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> deleteDocuments(
final RawData values,
final DocumentDeleteOptions options);
/**
* Removes multiple document
*
* @param values The keys of the documents or the documents themselves
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> deleteDocuments(final Collection> values);
/**
* Removes multiple document
*
* @param values The keys of the documents or the documents themselves
* @param options Additional options
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> deleteDocuments(
final Collection> values,
final DocumentDeleteOptions options);
/**
* Removes multiple document
*
* @param values The keys of the documents or the documents themselves
* @param type Deserialization target type for the returned documents.
* @param options Additional options
* @return information about the documents
* @see API
* Documentation
*/
CompletableFuture>> deleteDocuments(
final Collection> values,
final DocumentDeleteOptions options,
final Class type);
/**
* Checks if the document exists by reading a single document head
*
* @param key The key of the document
* @return true if the document was found, otherwise false
* @see API
* Documentation
*/
CompletableFuture documentExists(final String key);
/**
* Checks if the document exists by reading a single document head
*
* @param key The key of the document
* @param options Additional options, can be null
* @return true if the document was found, otherwise false
* @see API
* Documentation
*/
CompletableFuture documentExists(final String key, final DocumentExistsOptions options);
/**
* Returns an index
*
* Note: inverted indexes are not returned by this method. Use
* {@link ArangoCollectionAsync#getInvertedIndex(String)} instead.
*
* @param id The index-handle
* @return information about the index
* @see
* API Documentation
*/
CompletableFuture getIndex(final String id);
/**
* Returns an inverted index
*
* @param id The index-handle
* @return information about the index
* @see API Documentation
* @since ArangoDB 3.10
*/
CompletableFuture getInvertedIndex(String id);
/**
* Deletes an index
*
* @param id The index-handle
* @return the id of the index
* @see
* API Documentation
*/
CompletableFuture deleteIndex(final String id);
/**
* Creates a hash index for the collection, if it does not already exist.
*
* @param fields A list of attribute paths
* @param options Additional options, can be null
* @return information about the index
* @see
* API Documentation
* @deprecated use {@link #ensurePersistentIndex(Iterable, PersistentIndexOptions)} instead. Since ArangoDB 3.7 a
* hash index is an alias for a persistent index.
*/
@Deprecated
CompletableFuture ensureHashIndex(final Iterable fields, final HashIndexOptions options);
/**
* Creates a skip-list index for the collection, if it does not already exist.
*
* @param fields A list of attribute paths
* @param options Additional options, can be null
* @return information about the index
* @see API
* Documentation
* @deprecated use {@link #ensurePersistentIndex(Iterable, PersistentIndexOptions)} instead. Since ArangoDB 3.7 a
* skiplist index is an alias for a persistent index.
*/
@Deprecated
CompletableFuture ensureSkiplistIndex(
final Iterable fields,
final SkiplistIndexOptions options);
/**
* Creates a persistent index for the collection, if it does not already exist.
*
* @param fields A list of attribute paths
* @param options Additional options, can be null
* @return information about the index
* @see API
* Documentation
*/
CompletableFuture ensurePersistentIndex(
final Iterable fields,
final PersistentIndexOptions options);
/**
* Creates a geo-spatial index for the collection, if it does not already exist.
*
* @param fields A list of attribute paths
* @param options Additional options, can be null
* @return information about the index
* @see API
* Documentation
*/
CompletableFuture ensureGeoIndex(final Iterable fields, final GeoIndexOptions options);
/**
* Creates a fulltext index for the collection, if it does not already exist.
*
* @param fields A list of attribute paths
* @param options Additional options, can be null
* @return information about the index
* @see API
* Documentation
* @deprecated since ArangoDB 3.10, use ArangoSearch or Inverted indexes instead.
*/
@Deprecated
CompletableFuture ensureFulltextIndex(
final Iterable fields,
final FulltextIndexOptions options);
/**
* Creates a ttl index for the collection, if it does not already exist.
*
* @param fields A list of attribute paths
* @param options Additional options, can be null
* @return information about the index
* @see API
* Documentation
*/
CompletableFuture ensureTtlIndex(Iterable fields, TtlIndexOptions options);
/**
* Creates a ZKD multi-dimensional index for the collection, if it does not already exist.
* Note that zkd indexes are an experimental feature in ArangoDB 3.9.
*
* @param fields A list of attribute paths
* @param options Additional options, can be null
* @return information about the index
* @see API Documentation
* @since ArangoDB 3.9
*/
CompletableFuture ensureZKDIndex(final Iterable fields, final ZKDIndexOptions options);
/**
* Creates an inverted index for the collection, if it does not already exist.
*
* @param options index creation options
* @return information about the index
* @see API Documentation
* @since ArangoDB 3.10
*/
CompletableFuture ensureInvertedIndex(InvertedIndexOptions options);
/**
* Returns all indexes of the collection
*
* Note: inverted indexes are not returned by this method. Use
* {@link ArangoCollectionAsync#getInvertedIndexes()} instead.
*
* @return information about the indexes
* @see API
* Documentation
*/
CompletableFuture> getIndexes();
/**
* Fetches a list of all inverted indexes on this collection.
*
* @return information about the indexes
* @see API
* Documentation
* @since ArangoDB 3.10
*/
CompletableFuture> getInvertedIndexes();
/**
* Checks whether the collection exists
*
* @return true if the collection exists, otherwise false
*/
CompletableFuture exists();
/**
* Removes all documents from the collection, but leaves the indexes intact
*
* @return information about the collection
* @see API
* Documentation
*/
CompletableFuture truncate();
/**
* Removes all documents from the collection, but leaves the indexes intact
*
* @return information about the collection
* @see API
* Documentation
*/
CompletableFuture truncate(CollectionTruncateOptions options);
/**
* Counts the documents in a collection
*
* @return information about the collection, including the number of documents
* @see API
* Documentation
*/
CompletableFuture count();
/**
* Counts the documents in a collection
*
* @return information about the collection, including the number of documents
* @see API
* Documentation
*/
CompletableFuture count(CollectionCountOptions options);
/**
* Creates the collection
*
* @return information about the collection
* @see API
* Documentation
*/
CompletableFuture create();
/**
* Creates the collection
*
* @param options Additional options, can be null
* @return information about the collection
* @see API
* Documentation
*/
CompletableFuture create(final CollectionCreateOptions options);
/**
* Drops the collection
*
* @return void
* @see API
* Documentation
*/
CompletableFuture drop();
/**
* Drops the collection
*
* @param isSystem Whether or not the collection to drop is a system collection. This parameter must be set to
* true in
* order to drop a system collection.
* @return void
* @see API
* Documentation
*/
CompletableFuture drop(final boolean isSystem);
/**
* Returns information about the collection
*
* @return information about the collection
* @see API
* Documentation
*/
CompletableFuture getInfo();
/**
* Reads the properties of the specified collection
*
* @return properties of the collection
* @see API
* Documentation
*/
CompletableFuture getProperties();
/**
* Changes the properties of a collection
*
* @param options Additional options, can be null
* @return properties of the collection
* @see API
* Documentation
*/
CompletableFuture changeProperties(final CollectionPropertiesOptions options);
/**
* Renames a collection
*
* @param newName The new name
* @return information about the collection
* @see API
* Documentation
*/
CompletableFuture rename(final String newName);
/**
* Returns the responsible shard for the document.
* Please note that this API is only meaningful and available on a cluster coordinator.
*
* @param value A projection of the document containing at least the shard key (_key or a custom attribute) for
* which the responsible shard should be determined
* @return information about the responsible shard
* @see
*
* API Documentation
* @since ArangoDB 3.5.0
*/
CompletableFuture getResponsibleShard(final Object value);
/**
* Retrieve the collections revision
*
* @return information about the collection, including the collections revision
* @see
* API
* Documentation
*/
CompletableFuture getRevision();
/**
* Grants or revoke access to the collection for user user. You need permission to the _system database in order to
* execute this call.
*
* @param user The name of the user
* @param permissions The permissions the user grant
* @return void
* @see API
* Documentation
*/
CompletableFuture grantAccess(final String user, final Permissions permissions);
/**
* Revokes access to the collection for user user. You need permission to the _system database in order to execute
* this call.
*
* @param user The name of the user
* @return void
* @see API
* Documentation
*/
CompletableFuture revokeAccess(final String user);
/**
* Clear the collection access level, revert back to the default access level.
*
* @param user The name of the user
* @return void
* @see API
* Documentation
* @since ArangoDB 3.2.0
*/
CompletableFuture resetAccess(final String user);
/**
* Get the collection access level
*
* @param user The name of the user
* @return permissions of the user
* @see
*
* API Documentation
* @since ArangoDB 3.2.0
*/
CompletableFuture getPermissions(final String user);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy