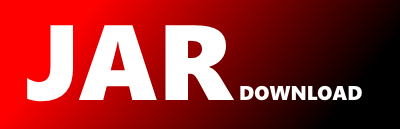
com.arangodb.shaded.vertx.ext.auth.impl.asn.ASN1 Maven / Gradle / Ivy
/*
* Copyright 2019 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package com.arangodb.shaded.vertx.ext.auth.impl.asn;
import com.arangodb.shaded.vertx.core.buffer.Buffer;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
public class ASN1 {
// Tag and data types
public final static int ANY = 0x00;
public final static int BOOLEAN = 0x01;
public final static int INTEGER = 0x02;
public final static int BIT_STRING = 0x03;
public final static int OCTET_STRING = 0x04;
public final static int NULL = 0x05;
public final static int OBJECT_IDENTIFIER = 0x06;
public final static int REAL = 0x09;
public final static int ENUMERATED = 0x0A;
public final static int UTF8_STRING = 0x0C;
public final static int SEQUENCE = 0x10;
public final static int SET = 0x11;
public final static int NUMERIC_STRING = 0x12;
public final static int PRINTABLE_STRING = 0x13;
public final static int VIDEOTEX_STRING = 0x15;
public final static int IA5_STRING = 0x16;
public final static int UTC_TIME = 0x17;
public final static int GRAPHIC_STRING = 0x19;
public final static int ISO646_STRING = 0x1A;
public final static int GENERAL_STRING = 0x1B;
public final static int UNIVERSAL_STRING = 0x1C;
public final static int BMP_STRING = 0x1E;
public final static int CONSTRUCTED = 0x20;
public final static int CONTEXT_SPECIFIC = 0x80;
public static byte[] length(int x) {
if (x <= 127) {
return new byte[]{(byte) x};
} else if (x < 256) {
return new byte[]{(byte) 0x81, (byte) x};
}
throw new IllegalArgumentException("length >= 256");
}
public static byte[] sequence(byte[] data) {
final byte sequenceTag = (byte) 0x30;
return Buffer.buffer()
.appendByte(sequenceTag)
.appendBytes(length(data.length))
.appendBytes(data)
.getBytes();
}
public static class ASN {
public final ASNTag tag;
public final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy