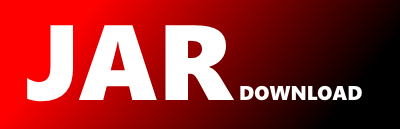
com.arangodb.ArangoDriver Maven / Gradle / Ivy
/*
* Copyright (C) 2012 tamtam180
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.arangodb;
import java.lang.reflect.Proxy;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import com.arangodb.entity.AdminLogEntity;
import com.arangodb.entity.AqlFunctionsEntity;
import com.arangodb.entity.ArangoUnixTime;
import com.arangodb.entity.ArangoVersion;
import com.arangodb.entity.BatchResponseEntity;
import com.arangodb.entity.BooleanResultEntity;
import com.arangodb.entity.CollectionEntity;
import com.arangodb.entity.CollectionOptions;
import com.arangodb.entity.CollectionsEntity;
import com.arangodb.entity.CursorEntity;
import com.arangodb.entity.DatabaseEntity;
import com.arangodb.entity.DefaultEntity;
import com.arangodb.entity.DeletedEntity;
import com.arangodb.entity.DocumentEntity;
import com.arangodb.entity.DocumentResultEntity;
import com.arangodb.entity.EdgeDefinitionEntity;
import com.arangodb.entity.EdgeEntity;
import com.arangodb.entity.Endpoint;
import com.arangodb.entity.GraphEntity;
import com.arangodb.entity.GraphsEntity;
import com.arangodb.entity.ImportResultEntity;
import com.arangodb.entity.IndexEntity;
import com.arangodb.entity.IndexType;
import com.arangodb.entity.IndexesEntity;
import com.arangodb.entity.JobsEntity;
import com.arangodb.entity.PlainEdgeEntity;
import com.arangodb.entity.Policy;
import com.arangodb.entity.ReplicationApplierConfigEntity;
import com.arangodb.entity.ReplicationApplierStateEntity;
import com.arangodb.entity.ReplicationInventoryEntity;
import com.arangodb.entity.ReplicationLoggerConfigEntity;
import com.arangodb.entity.ReplicationLoggerStateEntity;
import com.arangodb.entity.ReplicationSyncEntity;
import com.arangodb.entity.RestrictType;
import com.arangodb.entity.ScalarExampleEntity;
import com.arangodb.entity.SimpleByResultEntity;
import com.arangodb.entity.StatisticsDescriptionEntity;
import com.arangodb.entity.StatisticsEntity;
import com.arangodb.entity.StringsResultEntity;
import com.arangodb.entity.TransactionEntity;
import com.arangodb.entity.UserEntity;
import com.arangodb.http.BatchHttpManager;
import com.arangodb.http.BatchPart;
import com.arangodb.http.HttpManager;
import com.arangodb.http.InvocationHandlerImpl;
import com.arangodb.impl.ImplFactory;
import com.arangodb.impl.InternalBatchDriverImpl;
import com.arangodb.util.DumpHandler;
import com.arangodb.util.MapBuilder;
import com.arangodb.util.ResultSetUtils;
/**
* ArangoDB driver. Most of the functionality to use ArangoDB is provided via
* this class.
*
* @author tamtam180 - kirscheless at gmail.com
* @author gschwab
*/
public class ArangoDriver extends BaseArangoDriver {
// TODO Cas Operation as eTAG
// TODO Should fixed a Double check args.
// TODO Null check httpResponse.
// TODO コマンド式の実装に変更する。引数が増える度にメソッド数が爆発するのと、そうしないとバッチ処理も上手く書けないため。
// driver.execute(createDocumentCommand)
// class createDocumentCommand extends Command { }
private ArangoConfigure configure;
private BatchHttpManager httpManager;
private String baseUrl;
private InternalCursorDriver cursorDriver;
private InternalBatchDriverImpl batchDriver;
private InternalCollectionDriver collectionDriver;
private InternalDocumentDriver documentDriver;
// private InternalKVSDriverImpl kvsDriver;
private InternalIndexDriver indexDriver;
// private InternalEdgeDriverImpl edgeDriver;
private InternalAdminDriver adminDriver;
private InternalJobsDriver jobsDriver;
private InternalAqlFunctionsDriver aqlFunctionsDriver;
private InternalSimpleDriver simpleDriver;
private InternalUsersDriver usersDriver;
private InternalImportDriver importDriver;
private InternalDatabaseDriver databaseDriver;
private InternalEndpointDriver endpointDriver;
private InternalReplicationDriver replicationDriver;
private InternalGraphDriver graphDriver;
private InternalTransactionDriver transactionDriver;
private String database;
/**
* Constructor
*
* @param configure
*/
public ArangoDriver(ArangoConfigure configure) {
this(configure, null);
}
/**
* Constructor
*
* @param configure
* @param database
*/
public ArangoDriver(ArangoConfigure configure, String database) {
this.database = configure.getDefaultDatabase();
if (database != null) {
this.database = database;
}
this.configure = configure;
this.httpManager = configure.getHttpManager();
this.createModuleDrivers(false);
this.baseUrl = configure.getBaseUrl();
}
private void createModuleDrivers(boolean createProxys) {
if (!createProxys) {
this.cursorDriver = ImplFactory.createCursorDriver(configure, this.httpManager);
this.batchDriver = ImplFactory.createBatchDriver(configure, this.httpManager);
this.collectionDriver = ImplFactory.createCollectionDriver(configure, this.httpManager);
this.documentDriver = ImplFactory.createDocumentDriver(configure, this.httpManager);
this.indexDriver = ImplFactory.createIndexDriver(configure, this.httpManager);
this.adminDriver = ImplFactory.createAdminDriver(configure, this.httpManager);
this.aqlFunctionsDriver = ImplFactory.createAqlFunctionsDriver(configure, this.httpManager);
this.simpleDriver = ImplFactory.createSimpleDriver(configure, cursorDriver, this.httpManager);
this.usersDriver = ImplFactory.createUsersDriver(configure, this.httpManager);
this.importDriver = ImplFactory.createImportDriver(configure, this.httpManager);
this.databaseDriver = ImplFactory.createDatabaseDriver(configure, this.httpManager);
this.endpointDriver = ImplFactory.createEndpointDriver(configure, this.httpManager);
this.replicationDriver = ImplFactory.createReplicationDriver(configure, this.httpManager);
this.graphDriver = ImplFactory.createGraphDriver(configure, cursorDriver, this.httpManager);
this.jobsDriver = ImplFactory.createJobsDriver(configure, this.httpManager);
this.transactionDriver = ImplFactory.createTransactionDriver(configure, this.httpManager);
} else {
this.transactionDriver = (InternalTransactionDriver) Proxy.newProxyInstance(
InternalTransactionDriver.class.getClassLoader(),
new Class>[] { InternalTransactionDriver.class },
new InvocationHandlerImpl(this.transactionDriver));
this.jobsDriver = (InternalJobsDriver) Proxy.newProxyInstance(
InternalJobsDriver.class.getClassLoader(),
new Class>[] { InternalJobsDriver.class },
new InvocationHandlerImpl(this.jobsDriver));
this.cursorDriver = (InternalCursorDriver) Proxy.newProxyInstance(
InternalCursorDriver.class.getClassLoader(),
new Class>[] { InternalCursorDriver.class },
new InvocationHandlerImpl(this.cursorDriver));
this.collectionDriver = (InternalCollectionDriver) Proxy.newProxyInstance(
InternalCollectionDriver.class.getClassLoader(),
new Class>[] { InternalCollectionDriver.class },
new InvocationHandlerImpl(this.collectionDriver));
this.documentDriver = (InternalDocumentDriver) Proxy.newProxyInstance(
InternalDocumentDriver.class.getClassLoader(),
new Class>[] { InternalDocumentDriver.class },
new InvocationHandlerImpl(this.documentDriver));
this.indexDriver = (InternalIndexDriver) Proxy.newProxyInstance(
InternalIndexDriver.class.getClassLoader(),
new Class>[] { InternalIndexDriver.class },
new InvocationHandlerImpl(this.indexDriver));
this.adminDriver = (InternalAdminDriver) Proxy.newProxyInstance(
InternalAdminDriver.class.getClassLoader(),
new Class>[] { InternalAdminDriver.class },
new InvocationHandlerImpl(this.adminDriver));
this.aqlFunctionsDriver = (InternalAqlFunctionsDriver) Proxy.newProxyInstance(
InternalAqlFunctionsDriver.class.getClassLoader(),
new Class>[] { InternalAqlFunctionsDriver.class },
new InvocationHandlerImpl(this.aqlFunctionsDriver));
this.simpleDriver = (InternalSimpleDriver) Proxy.newProxyInstance(
InternalSimpleDriver.class.getClassLoader(),
new Class>[] { InternalSimpleDriver.class },
new InvocationHandlerImpl(this.simpleDriver));
this.usersDriver = (InternalUsersDriver) Proxy.newProxyInstance(
InternalUsersDriver.class.getClassLoader(),
new Class>[] { InternalUsersDriver.class },
new InvocationHandlerImpl(this.usersDriver));
this.importDriver = (InternalImportDriver) Proxy.newProxyInstance(
InternalImportDriver.class.getClassLoader(),
new Class>[] { InternalImportDriver.class },
new InvocationHandlerImpl(this.importDriver));
this.databaseDriver = (InternalDatabaseDriver) Proxy.newProxyInstance(
InternalDatabaseDriver.class.getClassLoader(),
new Class>[] { InternalDatabaseDriver.class },
new InvocationHandlerImpl(this.databaseDriver));
this.endpointDriver = (InternalEndpointDriver) Proxy.newProxyInstance(
InternalEndpointDriver.class.getClassLoader(),
new Class>[] { InternalEndpointDriver.class },
new InvocationHandlerImpl(this.endpointDriver));
this.replicationDriver = (InternalReplicationDriver) Proxy.newProxyInstance(
InternalReplicationDriver.class.getClassLoader(),
new Class>[] { InternalReplicationDriver.class },
new InvocationHandlerImpl(this.replicationDriver));
this.graphDriver = (InternalGraphDriver) Proxy.newProxyInstance(
InternalGraphDriver.class.getClassLoader(),
new Class>[] { InternalGraphDriver.class },
new InvocationHandlerImpl(this.graphDriver));
}
}
public void startBatchMode() throws ArangoException {
if (this.httpManager.isBatchModeActive()) {
throw new ArangoException("BatchMode is already active.");
}
this.httpManager.setBatchModeActive(true);
this.createModuleDrivers(true);
}
public void startAsyncMode(boolean fireAndForget) throws ArangoException {
if (this.httpManager.getHttpMode().equals(HttpManager.HttpMode.ASYNC)
|| this.httpManager.getHttpMode().equals(HttpManager.HttpMode.FIREANDFORGET)) {
throw new ArangoException("Arango driver already set to asynchronous mode.");
}
HttpManager.HttpMode mode = fireAndForget ? HttpManager.HttpMode.FIREANDFORGET : HttpManager.HttpMode.ASYNC;
this.httpManager.setHttpMode(mode);
this.createModuleDrivers(true);
this.httpManager.resetJobs();
}
public void stopAsyncMode() throws ArangoException {
if (this.httpManager.getHttpMode().equals(HttpManager.HttpMode.SYNC)) {
throw new ArangoException("Arango driver already set to synchronous mode.");
}
this.httpManager.setHttpMode(HttpManager.HttpMode.SYNC);
this.createModuleDrivers(false);
}
public String getLastJobId() {
return this.httpManager.getLastJobId();
}
public List getJobIds() {
return this.httpManager.getJobIds();
}
public List getJobs(JobsEntity.JobState jobState, int count) throws ArangoException {
return this.jobsDriver.getJobs(getDefaultDatabase(), jobState, count);
}
public List getJobs(JobsEntity.JobState jobState) throws ArangoException {
return this.jobsDriver.getJobs(getDefaultDatabase(), jobState);
}
public void deleteAllJobs() throws ArangoException {
this.jobsDriver.deleteAllJobs(getDefaultDatabase());
this.httpManager.resetJobs();
}
public void deleteJobById(String JobId) throws ArangoException {
this.jobsDriver.deleteJobById(getDefaultDatabase(), JobId);
}
public void deleteExpiredJobs(int timeStamp) throws ArangoException {
this.jobsDriver.deleteExpiredJobs(getDefaultDatabase(), timeStamp);
}
public T getJobResult(String jobId) throws ArangoException {
return this.jobsDriver.getJobResult(getDefaultDatabase(), jobId);
}
public DefaultEntity executeBatch() throws ArangoException {
if (!this.httpManager.isBatchModeActive()) {
throw new ArangoException("BatchMode is not active.");
}
List callStack = this.httpManager.getCallStack();
this.cancelBatchMode();
DefaultEntity result = this.batchDriver.executeBatch(callStack, this.getDefaultDatabase());
return result;
}
public T getBatchResponseByRequestId(String requestId) throws ArangoException {
BatchResponseEntity batchResponseEntity = this.batchDriver.getBatchResponseListEntity().getResponseFromRequestId(
requestId);
try {
this.httpManager.setPreDefinedResponse(batchResponseEntity.getHttpResponseEntity());
return (T) batchResponseEntity
.getInvocationObject()
.getMethod()
.invoke(
batchResponseEntity.getInvocationObject().getArangoDriver(),
batchResponseEntity.getInvocationObject().getArgs());
} catch (Exception e) {
throw new ArangoException(e);
}
}
public void cancelBatchMode() throws ArangoException {
if (!this.httpManager.isBatchModeActive()) {
throw new ArangoException("BatchMode is not active.");
}
this.httpManager.setBatchModeActive(false);
this.createModuleDrivers(false);
}
public String getDefaultDatabase() {
return database;
}
public void setDefaultDatabase(String database) {
this.database = database;
}
// ***************************************
// *** start of collection ***************
// ***************************************
public CollectionEntity createCollection(String name) throws ArangoException {
return collectionDriver.createCollection(getDefaultDatabase(), name, new CollectionOptions());
}
public CollectionEntity createCollection(String name, CollectionOptions collectionOptions) throws ArangoException {
return collectionDriver.createCollection(getDefaultDatabase(), name, collectionOptions);
}
public CollectionEntity getCollection(long id) throws ArangoException {
return getCollection(String.valueOf(id));
}
public CollectionEntity getCollection(String name) throws ArangoException {
return collectionDriver.getCollection(getDefaultDatabase(), name);
}
public CollectionEntity getCollectionProperties(long id) throws ArangoException {
return getCollectionProperties(String.valueOf(id));
}
public CollectionEntity getCollectionProperties(String name) throws ArangoException {
return collectionDriver.getCollectionProperties(getDefaultDatabase(), name);
}
public CollectionEntity getCollectionRevision(long id) throws ArangoException {
return getCollectionRevision(String.valueOf(id));
}
public CollectionEntity getCollectionRevision(String name) throws ArangoException {
return collectionDriver.getCollectionRevision(getDefaultDatabase(), name);
}
public CollectionEntity getCollectionCount(long id) throws ArangoException {
return getCollectionCount(String.valueOf(id));
}
public CollectionEntity getCollectionCount(String name) throws ArangoException {
return collectionDriver.getCollectionCount(getDefaultDatabase(), name);
}
public CollectionEntity getCollectionFigures(long id) throws ArangoException {
return getCollectionFigures(String.valueOf(id));
}
public CollectionEntity getCollectionFigures(String name) throws ArangoException {
return collectionDriver.getCollectionFigures(getDefaultDatabase(), name);
}
public CollectionEntity getCollectionChecksum(String name, Boolean withRevisions, Boolean withData)
throws ArangoException {
return collectionDriver.getCollectionChecksum(getDefaultDatabase(), name, withRevisions, withData);
}
public CollectionsEntity getCollections() throws ArangoException {
return collectionDriver.getCollections(getDefaultDatabase(), null);
}
public CollectionsEntity getCollections(Boolean excludeSystem) throws ArangoException {
return collectionDriver.getCollections(getDefaultDatabase(), excludeSystem);
}
public CollectionEntity loadCollection(long id) throws ArangoException {
return collectionDriver.loadCollection(getDefaultDatabase(), String.valueOf(id), null);
}
public CollectionEntity loadCollection(String name) throws ArangoException {
return collectionDriver.loadCollection(getDefaultDatabase(), name, null);
}
public CollectionEntity loadCollection(long id, Boolean count) throws ArangoException {
return collectionDriver.loadCollection(getDefaultDatabase(), String.valueOf(id), count);
}
public CollectionEntity loadCollection(String name, Boolean count) throws ArangoException {
return collectionDriver.loadCollection(getDefaultDatabase(), name, count);
}
public CollectionEntity unloadCollection(long id) throws ArangoException {
return unloadCollection(String.valueOf(id));
}
public CollectionEntity unloadCollection(String name) throws ArangoException {
return collectionDriver.unloadCollection(getDefaultDatabase(), name);
}
public CollectionEntity truncateCollection(long id) throws ArangoException {
return truncateCollection(String.valueOf(id));
}
public CollectionEntity truncateCollection(String name) throws ArangoException {
return collectionDriver.truncateCollection(getDefaultDatabase(), name);
}
public CollectionEntity setCollectionProperties(long id, Boolean newWaitForSync, Long journalSize)
throws ArangoException {
return collectionDriver.setCollectionProperties(
getDefaultDatabase(),
String.valueOf(id),
newWaitForSync,
journalSize);
}
public CollectionEntity setCollectionProperties(String name, Boolean newWaitForSync, Long journalSize)
throws ArangoException {
return collectionDriver.setCollectionProperties(getDefaultDatabase(), name, newWaitForSync, journalSize);
}
public CollectionEntity renameCollection(long id, String newName) throws ArangoException {
return renameCollection(String.valueOf(id), newName);
}
public CollectionEntity renameCollection(String name, String newName) throws ArangoException {
return collectionDriver.renameCollection(getDefaultDatabase(), name, newName);
}
public CollectionEntity deleteCollection(long id) throws ArangoException {
return deleteCollection(String.valueOf(id));
}
public CollectionEntity deleteCollection(String name) throws ArangoException {
return collectionDriver.deleteCollection(getDefaultDatabase(), name);
}
// ***************************************
// *** end of collection *****************
// ***************************************
// ***************************************
// *** start of document *****************
// ***************************************
public DocumentEntity> createDocument(long collectionId, Object value) throws ArangoException {
return createDocument(String.valueOf(collectionId), value, null, null);
}
public DocumentEntity createDocument(String collectionName, Object value) throws ArangoException {
return documentDriver.createDocument(getDefaultDatabase(), collectionName, null, value, null, null);
}
public DocumentEntity> createDocument(long collectionId, String documentKey, Object value) throws ArangoException {
return createDocument(String.valueOf(collectionId), documentKey, value, null, null);
}
public DocumentEntity createDocument(String collectionName, String documentKey, Object value)
throws ArangoException {
return documentDriver.createDocument(getDefaultDatabase(), collectionName, documentKey, value, null, null);
}
public DocumentEntity>
createDocument(long collectionId, Object value, Boolean createCollection, Boolean waitForSync)
throws ArangoException {
return createDocument(String.valueOf(collectionId), value, createCollection, waitForSync);
}
public DocumentEntity createDocument(
String collectionName,
Object value,
Boolean createCollection,
Boolean waitForSync) throws ArangoException {
return documentDriver.createDocument(
getDefaultDatabase(),
collectionName,
null,
value,
createCollection,
waitForSync);
}
public DocumentEntity> createDocument(
long collectionId,
String documentKey,
Object value,
Boolean createCollection,
Boolean waitForSync) throws ArangoException {
return createDocument(String.valueOf(collectionId), documentKey, value, createCollection, waitForSync);
}
public DocumentEntity createDocument(
String collectionName,
String documentKey,
Object value,
Boolean createCollection,
Boolean waitForSync) throws ArangoException {
return documentDriver.createDocument(
getDefaultDatabase(),
collectionName,
documentKey,
value,
createCollection,
waitForSync);
}
public DocumentEntity> replaceDocument(long collectionId, long documentId, Object value) throws ArangoException {
return replaceDocument(createDocumentHandle(collectionId, String.valueOf(documentId)), value, null, null, null);
}
public DocumentEntity> replaceDocument(String collectionName, long documentId, Object value) throws ArangoException {
return replaceDocument(createDocumentHandle(collectionName, String.valueOf(documentId)), value, null, null, null);
}
public DocumentEntity> replaceDocument(long collectionId, String documentKey, Object value) throws ArangoException {
return replaceDocument(createDocumentHandle(collectionId, documentKey), value, null, null, null);
}
public DocumentEntity> replaceDocument(String collectionName, String documentKey, Object value)
throws ArangoException {
return replaceDocument(createDocumentHandle(collectionName, documentKey), value, null, null, null);
}
public DocumentEntity replaceDocument(String documentHandle, Object value) throws ArangoException {
return documentDriver.replaceDocument(getDefaultDatabase(), documentHandle, value, null, null, null);
}
public DocumentEntity> replaceDocument(
long collectionId,
long documentId,
Object value,
Long rev,
Policy policy,
Boolean waitForSync) throws ArangoException {
return replaceDocument(
createDocumentHandle(collectionId, String.valueOf(documentId)),
value,
rev,
policy,
waitForSync);
}
public DocumentEntity> replaceDocument(
String collectionName,
long documentId,
Object value,
Long rev,
Policy policy,
Boolean waitForSync) throws ArangoException {
return replaceDocument(
createDocumentHandle(collectionName, String.valueOf(documentId)),
value,
rev,
policy,
waitForSync);
}
public DocumentEntity> replaceDocument(
long collectionId,
String documentKey,
Object value,
Long rev,
Policy policy,
Boolean waitForSync) throws ArangoException {
return replaceDocument(createDocumentHandle(collectionId, documentKey), value, rev, policy, waitForSync);
}
public DocumentEntity> replaceDocument(
String collectionName,
String documentKey,
Object value,
Long rev,
Policy policy,
Boolean waitForSync) throws ArangoException {
return replaceDocument(createDocumentHandle(collectionName, documentKey), value, rev, policy, waitForSync);
}
public DocumentEntity replaceDocument(
String documentHandle,
Object value,
Long rev,
Policy policy,
Boolean waitForSync) throws ArangoException {
return documentDriver.replaceDocument(getDefaultDatabase(), documentHandle, value, rev, policy, waitForSync);
}
public DocumentEntity> updateDocument(long collectionId, long documentId, Object value) throws ArangoException {
return updateDocument(createDocumentHandle(collectionId, String.valueOf(documentId)), value, null, null, null, null);
}
public DocumentEntity> updateDocument(String collectionName, long documentId, Object value) throws ArangoException {
return updateDocument(
createDocumentHandle(collectionName, String.valueOf(documentId)),
value,
null,
null,
null,
null);
}
public DocumentEntity> updateDocument(long collectionId, String documentKey, Object value) throws ArangoException {
return updateDocument(createDocumentHandle(collectionId, documentKey), value, null, null, null, null);
}
public DocumentEntity> updateDocument(String collectionName, String documentKey, Object value)
throws ArangoException {
return updateDocument(createDocumentHandle(collectionName, documentKey), value, null, null, null, null);
}
public DocumentEntity updateDocument(String documentHandle, Object value) throws ArangoException {
return documentDriver.updateDocument(getDefaultDatabase(), documentHandle, value, null, null, null, null);
}
public DocumentEntity> updateDocument(long collectionId, long documentId, Object value, Boolean keepNull)
throws ArangoException {
return updateDocument(
createDocumentHandle(collectionId, String.valueOf(documentId)),
value,
null,
null,
null,
keepNull);
}
public DocumentEntity> updateDocument(String collectionName, long documentId, Object value, Boolean keepNull)
throws ArangoException {
return updateDocument(
createDocumentHandle(collectionName, String.valueOf(documentId)),
value,
null,
null,
null,
keepNull);
}
public DocumentEntity> updateDocument(long collectionId, String documentKey, Object value, Boolean keepNull)
throws ArangoException {
return updateDocument(createDocumentHandle(collectionId, documentKey), value, null, null, null, keepNull);
}
public DocumentEntity> updateDocument(String collectionName, String documentKey, Object value, Boolean keepNull)
throws ArangoException {
return updateDocument(createDocumentHandle(collectionName, documentKey), value, null, null, null, keepNull);
}
public DocumentEntity updateDocument(String documentHandle, Object value, Boolean keepNull)
throws ArangoException {
return documentDriver.updateDocument(getDefaultDatabase(), documentHandle, value, null, null, null, keepNull);
}
public DocumentEntity> updateDocument(
long collectionId,
long documentId,
Object value,
Long rev,
Policy policy,
Boolean waitForSync,
Boolean keepNull) throws ArangoException {
return updateDocument(
createDocumentHandle(collectionId, String.valueOf(documentId)),
value,
rev,
policy,
waitForSync,
keepNull);
}
public DocumentEntity> updateDocument(
String collectionName,
long documentId,
Object value,
Long rev,
Policy policy,
Boolean waitForSync,
Boolean keepNull) throws ArangoException {
return updateDocument(
createDocumentHandle(collectionName, String.valueOf(documentId)),
value,
rev,
policy,
waitForSync,
keepNull);
}
public DocumentEntity> updateDocument(
long collectionId,
String documentKey,
Object value,
Long rev,
Policy policy,
Boolean waitForSync,
Boolean keepNull) throws ArangoException {
return updateDocument(createDocumentHandle(collectionId, documentKey), value, rev, policy, waitForSync, keepNull);
}
public DocumentEntity> updateDocument(
String collectionName,
String documentKey,
Object value,
Long rev,
Policy policy,
Boolean waitForSync,
Boolean keepNull) throws ArangoException {
return updateDocument(createDocumentHandle(collectionName, documentKey), value, rev, policy, waitForSync, keepNull);
}
public DocumentEntity updateDocument(
String documentHandle,
Object value,
Long rev,
Policy policy,
Boolean waitForSync,
Boolean keepNull) throws ArangoException {
return documentDriver.updateDocument(
getDefaultDatabase(),
documentHandle,
value,
rev,
policy,
waitForSync,
keepNull);
}
public List getDocuments(long collectionId) throws ArangoException {
return getDocuments(String.valueOf(collectionId), false);
}
public List getDocuments(String collectionName) throws ArangoException {
return documentDriver.getDocuments(getDefaultDatabase(), collectionName, false);
}
public List getDocuments(long collectionId, boolean handleConvert) throws ArangoException {
return getDocuments(String.valueOf(collectionId), handleConvert);
}
public List getDocuments(String collectionName, boolean handleConvert) throws ArangoException {
return documentDriver.getDocuments(getDefaultDatabase(), collectionName, handleConvert);
}
public long checkDocument(long collectionId, long documentId) throws ArangoException {
return checkDocument(createDocumentHandle(collectionId, String.valueOf(documentId)));
}
public long checkDocument(String collectionName, long documentId) throws ArangoException {
return checkDocument(createDocumentHandle(collectionName, String.valueOf(documentId)));
}
public long checkDocument(long collectionId, String documentKey) throws ArangoException {
return checkDocument(createDocumentHandle(collectionId, documentKey));
}
public long checkDocument(String collectionName, String documentKey) throws ArangoException {
return checkDocument(createDocumentHandle(collectionName, documentKey));
}
public long checkDocument(String documentHandle) throws ArangoException {
return documentDriver.checkDocument(getDefaultDatabase(), documentHandle);
}
public DocumentEntity getDocument(long collectionId, long documentId, Class> clazz) throws ArangoException {
return getDocument(createDocumentHandle(collectionId, String.valueOf(documentId)), clazz);
}
public DocumentEntity getDocument(String collectionName, long documentId, Class> clazz)
throws ArangoException {
return getDocument(createDocumentHandle(collectionName, String.valueOf(documentId)), clazz);
}
public DocumentEntity getDocument(long collectionId, String documentKey, Class> clazz)
throws ArangoException {
return getDocument(createDocumentHandle(collectionId, documentKey), clazz);
}
public DocumentEntity getDocument(String collectionName, String documentKey, Class> clazz)
throws ArangoException {
return getDocument(createDocumentHandle(collectionName, documentKey), clazz);
}
public DocumentEntity getDocument(String documentHandle, Class> clazz) throws ArangoException {
return documentDriver.getDocument(getDefaultDatabase(), documentHandle, clazz, null, null);
}
public DocumentEntity getDocument(
String documentHandle,
Class> clazz,
Long ifNoneMatchRevision,
Long ifMatchRevision) throws ArangoException {
return documentDriver
.getDocument(getDefaultDatabase(), documentHandle, clazz, ifNoneMatchRevision, ifMatchRevision);
}
public DocumentEntity> deleteDocument(long collectionId, long documentId) throws ArangoException {
return deleteDocument(createDocumentHandle(collectionId, String.valueOf(documentId)), null, null);
}
public DocumentEntity> deleteDocument(String collectionName, long documentId) throws ArangoException {
return deleteDocument(createDocumentHandle(collectionName, String.valueOf(documentId)), null, null);
}
public DocumentEntity> deleteDocument(long collectionId, String documentKey) throws ArangoException {
return deleteDocument(createDocumentHandle(collectionId, documentKey), null, null);
}
public DocumentEntity> deleteDocument(String collectionName, String documentKey) throws ArangoException {
return deleteDocument(createDocumentHandle(collectionName, documentKey), null, null);
}
public DocumentEntity> deleteDocument(String documentHandle) throws ArangoException {
return documentDriver.deleteDocument(getDefaultDatabase(), documentHandle, null, null);
}
public DocumentEntity> deleteDocument(long collectionId, long documentId, Long rev, Policy policy)
throws ArangoException {
return deleteDocument(createDocumentHandle(collectionId, String.valueOf(documentId)), rev, policy);
}
public DocumentEntity> deleteDocument(String collectionName, long documentId, Long rev, Policy policy)
throws ArangoException {
return deleteDocument(createDocumentHandle(collectionName, String.valueOf(documentId)), rev, policy);
}
public DocumentEntity> deleteDocument(long collectionId, String documentKey, Long rev, Policy policy)
throws ArangoException {
return deleteDocument(createDocumentHandle(collectionId, documentKey), rev, policy);
}
public DocumentEntity> deleteDocument(String collectionName, String documentKey, Long rev, Policy policy)
throws ArangoException {
return deleteDocument(createDocumentHandle(collectionName, documentKey), rev, policy);
}
public DocumentEntity> deleteDocument(String documentHandle, Long rev, Policy policy) throws ArangoException {
return documentDriver.deleteDocument(getDefaultDatabase(), documentHandle, rev, policy);
}
// ***************************************
// *** end of document *******************
// ***************************************
// ***************************************
// *** start of cursor *******************
// ***************************************
public CursorEntity> validateQuery(String query) throws ArangoException {
return cursorDriver.validateQuery(getDefaultDatabase(), query);
}
public CursorEntity executeQuery(
String query,
Map bindVars,
Class clazz,
Boolean calcCount,
Integer batchSize) throws ArangoException {
return cursorDriver.executeQuery(getDefaultDatabase(), query, bindVars, clazz, calcCount, batchSize);
}
public CursorEntity continueQuery(long cursorId, Class>... clazz) throws ArangoException {
return cursorDriver.continueQuery(getDefaultDatabase(), cursorId, clazz);
}
public DefaultEntity finishQuery(long cursorId) throws ArangoException {
return cursorDriver.finishQuery(getDefaultDatabase(), cursorId);
}
public CursorResultSet executeQueryWithResultSet(
String query,
Map bindVars,
Class clazz,
Boolean calcCount,
Integer batchSize) throws ArangoException {
return cursorDriver.executeQueryWithResultSet(getDefaultDatabase(), query, bindVars, clazz, calcCount, batchSize);
}
public IndexEntity createIndex(long collectionId, IndexType type, boolean unique, String... fields)
throws ArangoException {
return createIndex(String.valueOf(collectionId), type, unique, fields);
}
public IndexEntity createIndex(String collectionName, IndexType type, boolean unique, String... fields)
throws ArangoException {
return indexDriver.createIndex(getDefaultDatabase(), collectionName, type, unique, fields);
}
public IndexEntity createHashIndex(String collectionName, boolean unique, String... fields) throws ArangoException {
return indexDriver.createIndex(getDefaultDatabase(), collectionName, IndexType.HASH, unique, fields);
}
public IndexEntity createGeoIndex(String collectionName, boolean unique, String... fields) throws ArangoException {
return indexDriver.createIndex(getDefaultDatabase(), collectionName, IndexType.GEO, unique, fields);
}
public IndexEntity createSkipListIndex(String collectionName, boolean unique, String... fields)
throws ArangoException {
return indexDriver.createIndex(getDefaultDatabase(), collectionName, IndexType.SKIPLIST, unique, fields);
}
public IndexEntity createCappedIndex(long collectionId, int size) throws ArangoException {
return createCappedIndex(String.valueOf(collectionId), size);
}
public IndexEntity createCappedIndex(String collectionName, int size) throws ArangoException {
return indexDriver.createCappedIndex(getDefaultDatabase(), collectionName, size);
}
public IndexEntity createFulltextIndex(long collectionId, String... fields) throws ArangoException {
return createFulltextIndex(String.valueOf(collectionId), null, fields);
}
public IndexEntity createFulltextIndex(String collectionName, String... fields) throws ArangoException {
return createFulltextIndex(collectionName, null, fields);
}
public IndexEntity createFulltextIndex(long collectionId, Integer minLength, String... fields) throws ArangoException {
return createFulltextIndex(String.valueOf(collectionId), minLength, fields);
}
public IndexEntity createFulltextIndex(String collectionName, Integer minLength, String... fields)
throws ArangoException {
return indexDriver.createFulltextIndex(getDefaultDatabase(), collectionName, minLength, fields);
}
public IndexEntity deleteIndex(String indexHandle) throws ArangoException {
return indexDriver.deleteIndex(getDefaultDatabase(), indexHandle);
}
public IndexEntity getIndex(String indexHandle) throws ArangoException {
return indexDriver.getIndex(getDefaultDatabase(), indexHandle);
}
public IndexesEntity getIndexes(long collectionId) throws ArangoException {
return getIndexes(String.valueOf(collectionId));
}
public IndexesEntity getIndexes(String collectionName) throws ArangoException {
return indexDriver.getIndexes(getDefaultDatabase(), collectionName);
}
public AdminLogEntity getServerLog(
Integer logLevel,
Boolean logLevelUpTo,
Integer start,
Integer size,
Integer offset,
Boolean sortAsc,
String text) throws ArangoException {
return adminDriver.getServerLog(logLevel, logLevelUpTo, start, size, offset, sortAsc, text);
}
public StatisticsEntity getStatistics() throws ArangoException {
return adminDriver.getStatistics();
}
public StatisticsDescriptionEntity getStatisticsDescription() throws ArangoException {
return adminDriver.getStatisticsDescription();
}
public ArangoVersion getVersion() throws ArangoException {
return adminDriver.getVersion();
}
public ArangoUnixTime getTime() throws ArangoException {
return adminDriver.getTime();
}
public DefaultEntity reloadRouting() throws ArangoException {
return adminDriver.reloadRouting();
}
public DefaultEntity executeScript(String jsCode) throws ArangoException {
return adminDriver.executeScript(getDefaultDatabase(), jsCode);
}
// ***************************************
// *** end of admin **********************
// ***************************************
// ***************************************
// *** start of simple *******************
// ***************************************
public CursorEntity executeSimpleByExample(
String collectionName,
Map example,
int skip,
int limit,
Class clazz) throws ArangoException {
return simpleDriver.executeSimpleByExample(getDefaultDatabase(), collectionName, example, skip, limit, clazz);
}
public CursorResultSet executeSimpleByExampleWithResusltSet(
String collectionName,
Map example,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleByExampleWithResultSet(
getDefaultDatabase(),
collectionName,
example,
skip,
limit,
clazz);
}
public CursorEntity> executeSimpleByExampleWithDocument(
String collectionName,
Map example,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleByExampleWithDocument(
getDefaultDatabase(),
collectionName,
example,
skip,
limit,
clazz);
}
public CursorResultSet> executeSimpleByExampleWithDocumentResusltSet(
String collectionName,
Map example,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleByExampleWithDocumentResultSet(
getDefaultDatabase(),
collectionName,
example,
skip,
limit,
clazz);
}
public CursorEntity executeSimpleAll(String collectionName, int skip, int limit, Class> clazz)
throws ArangoException {
return simpleDriver.executeSimpleAll(getDefaultDatabase(), collectionName, skip, limit, clazz);
}
public CursorResultSet
executeSimpleAllWithResultSet(String collectionName, int skip, int limit, Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleAllWithResultSet(getDefaultDatabase(), collectionName, skip, limit, clazz);
}
public CursorEntity> executeSimpleAllWithDocument(
String collectionName,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleAllWithDocument(getDefaultDatabase(), collectionName, skip, limit, clazz);
}
public CursorResultSet> executeSimpleAllWithDocumentResultSet(
String collectionName,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleAllWithDocumentResultSet(getDefaultDatabase(), collectionName, skip, limit, clazz);
}
public ScalarExampleEntity executeSimpleFirstExample(
String collectionName,
Map example,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleFirstExample(getDefaultDatabase(), collectionName, example, clazz);
}
public ScalarExampleEntity executeSimpleAny(String collectionName, Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleAny(getDefaultDatabase(), collectionName, clazz);
}
public CursorEntity executeSimpleRange(
String collectionName,
String attribute,
Object left,
Object right,
Boolean closed,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleRange(
getDefaultDatabase(),
collectionName,
attribute,
left,
right,
closed,
skip,
limit,
clazz);
}
public CursorResultSet executeSimpleRangeWithResultSet(
String collectionName,
String attribute,
Object left,
Object right,
Boolean closed,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleRangeWithResultSet(
getDefaultDatabase(),
collectionName,
attribute,
left,
right,
closed,
skip,
limit,
clazz);
}
public CursorEntity> executeSimpleRangeWithDocument(
String collectionName,
String attribute,
Object left,
Object right,
Boolean closed,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleRangeWithDocument(
getDefaultDatabase(),
collectionName,
attribute,
left,
right,
closed,
skip,
limit,
clazz);
}
public CursorResultSet> executeSimpleRangeWithDocumentResultSet(
String collectionName,
String attribute,
Object left,
Object right,
Boolean closed,
int skip,
int limit,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleRangeWithDocumentResultSet(
getDefaultDatabase(),
collectionName,
attribute,
left,
right,
closed,
skip,
limit,
clazz);
}
public CursorEntity executeSimpleFulltext(
String collectionName,
String attribute,
String query,
int skip,
int limit,
String index,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleFulltext(
getDefaultDatabase(),
collectionName,
attribute,
query,
skip,
limit,
index,
clazz);
}
public CursorResultSet executeSimpleFulltextWithResultSet(
String collectionName,
String attribute,
String query,
int skip,
int limit,
String index,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleFulltextWithResultSet(
getDefaultDatabase(),
collectionName,
attribute,
query,
skip,
limit,
index,
clazz);
}
public CursorEntity> executeSimpleFulltextWithDocument(
String collectionName,
String attribute,
String query,
int skip,
int limit,
String index,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleFulltextWithDocument(
getDefaultDatabase(),
collectionName,
attribute,
query,
skip,
limit,
index,
clazz);
}
public CursorResultSet> executeSimpleFulltextWithDocumentResultSet(
String collectionName,
String attribute,
String query,
int skip,
int limit,
String index,
Class> clazz) throws ArangoException {
return simpleDriver.executeSimpleFulltextWithDocumentResultSet(
getDefaultDatabase(),
collectionName,
attribute,
query,
skip,
limit,
index,
clazz);
}
public SimpleByResultEntity executeSimpleRemoveByExample(
String collectionName,
Map example,
Boolean waitForSync,
Integer limit) throws ArangoException {
return simpleDriver.executeSimpleRemoveByExample(getDefaultDatabase(), collectionName, example, waitForSync, limit);
}
public SimpleByResultEntity executeSimpleReplaceByExample(
String collectionName,
Map example,
Map newValue,
Boolean waitForSync,
Integer limit) throws ArangoException {
return simpleDriver.executeSimpleReplaceByExample(
getDefaultDatabase(),
collectionName,
example,
newValue,
waitForSync,
limit);
}
public SimpleByResultEntity executeSimpleUpdateByExample(
String collectionName,
Map example,
Map newValue,
Boolean keepNull,
Boolean waitForSync,
Integer limit) throws ArangoException {
return simpleDriver.executeSimpleUpdateByExample(
getDefaultDatabase(),
collectionName,
example,
newValue,
keepNull,
waitForSync,
limit);
}
/**
* @param collectionName
* @param count
* @param clazz
* @return
* @throws ArangoException
* @since 1.4.0
*/
public DocumentResultEntity executeSimpleFirst(String collectionName, Integer count, Class> clazz)
throws ArangoException {
return simpleDriver.executeSimpleFirst(getDefaultDatabase(), collectionName, count, clazz);
}
/**
* @param collectionName
* @param count
* @param clazz
* @return
* @throws ArangoException
* @since 1.4.0
*/
public DocumentResultEntity executeSimpleLast(String collectionName, Integer count, Class> clazz)
throws ArangoException {
return simpleDriver.executeSimpleLast(getDefaultDatabase(), collectionName, count, clazz);
}
// ***************************************
// *** end of simple *********************
// ***************************************
// ***************************************
// *** start of users ********************
// ***************************************
public DefaultEntity createUser(String username, String passwd, Boolean active, Map extra)
throws ArangoException {
return usersDriver.createUser(getDefaultDatabase(), username, passwd, active, extra);
}
public DefaultEntity replaceUser(String username, String passwd, Boolean active, Map extra)
throws ArangoException {
return usersDriver.replaceUser(getDefaultDatabase(), username, passwd, active, extra);
}
public DefaultEntity updateUser(String username, String passwd, Boolean active, Map extra)
throws ArangoException {
return usersDriver.updateUser(getDefaultDatabase(), username, passwd, active, extra);
}
public DefaultEntity deleteUser(String username) throws ArangoException {
return usersDriver.deleteUser(getDefaultDatabase(), username);
}
public UserEntity getUser(String username) throws ArangoException {
return usersDriver.getUser(getDefaultDatabase(), username);
}
// Original (ArangoDB does not implements this API)
public List> getUsersDocument() throws ArangoException {
CursorResultSet> rs = executeSimpleAllWithDocumentResultSet(
"_users",
0,
0,
UserEntity.class);
return ResultSetUtils.toList(rs);
}
// Original (ArangoDB does not implements this API)
public List getUsers() throws ArangoException {
CursorResultSet rs = executeSimpleAllWithResultSet("_users", 0, 0, UserEntity.class);
return ResultSetUtils.toList(rs);
}
// ***************************************
// *** end of users **********************
// ***************************************
// ***************************************
// *** start of import *******************
// ***************************************
public ImportResultEntity importDocuments(String collection, Boolean createCollection, Collection> values)
throws ArangoException {
return importDriver.importDocuments(getDefaultDatabase(), collection, createCollection, values);
}
// public void importDocuments(String collection, Boolean createCollection,
// Iterator> itr) throws ArangoException {
// importDriver.importDocuments(collection, createCollection, itr);
// }
public ImportResultEntity importDocumentsByHeaderValues(
String collection,
Boolean createCollection,
Collection extends Collection>> headerValues) throws ArangoException {
return importDriver.importDocumentsByHeaderValues(getDefaultDatabase(), collection, createCollection, headerValues);
}
// ***************************************
// *** end of import *********************
// ***************************************
// ***************************************
// *** start of database *****************
// ***************************************
/**
* @return
* @throws ArangoException
* @see http://www.arangodb.com/manuals/current/HttpDatabase.html#
* HttpDatabaseCurrent
* @since 1.4.0
*/
public DatabaseEntity getCurrentDatabase() throws ArangoException {
return databaseDriver.getCurrentDatabase();
}
/**
* @return
* @throws ArangoException
* @see http
* ://www.arangodb.com/manuals/current/HttpDatabase.html#HttpDatabaseList2
* @since 1.4.0
*/
public StringsResultEntity getDatabases() throws ArangoException {
return getDatabases(false);
}
/**
* @param currentUserAccessableOnly
* @return
* @throws ArangoException
* @see http
* ://www.arangodb.com/manuals/current/HttpDatabase.html#HttpDatabaseList
* @since 1.4.1
*/
public StringsResultEntity getDatabases(boolean currentUserAccessableOnly) throws ArangoException {
return databaseDriver.getDatabases(currentUserAccessableOnly, null, null);
}
/**
* @param username
* @param password
* @return
* @throws ArangoException
* @since 1.4.1
*/
public StringsResultEntity getDatabases(String username, String password) throws ArangoException {
return databaseDriver.getDatabases(true, username, password);
}
/**
* @param database
* @param users
* @return
* @throws ArangoException
* @see http
* ://www.arangodb.com/manuals/current/HttpDatabase.html#HttpDatabaseCreate
* @since 1.4.0
*/
public BooleanResultEntity createDatabase(String database, UserEntity... users) throws ArangoException {
return databaseDriver.createDatabase(database, users);
}
/**
* @param database
* @return
* @throws ArangoException
* @see http
* ://www.arangodb.com/manuals/current/HttpDatabase.html#HttpDatabaseDelete
* @since 1.4.0
*/
public BooleanResultEntity deleteDatabase(String database) throws ArangoException {
return databaseDriver.deleteDatabase(database);
}
// ***************************************
// *** end of database *******************
// ***************************************
// ***************************************
// *** start of endpoint *****************
// ***************************************
/**
* @param endpoint
* @param databases
* @return
* @throws ArangoException
* @since 1.4.0
*/
public BooleanResultEntity createEndpoint(String endpoint, String... databases) throws ArangoException {
return endpointDriver.createEndpoint(endpoint, databases);
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public List getEndpoints() throws ArangoException {
return endpointDriver.getEndpoints();
}
/**
* @param endpoint
* @return
* @throws ArangoException
* @since 1.4.0
*/
public BooleanResultEntity deleteEndpoint(String endpoint) throws ArangoException {
return endpointDriver.deleteEndpoint(endpoint);
}
// ***************************************
// *** end of endpoint *******************
// ***************************************
// ***************************************
// *** start of replication **************
// ***************************************
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationInventoryEntity getReplicationInventory() throws ArangoException {
return replicationDriver.getReplicationInventory(getDefaultDatabase(), null);
}
/**
* @param includeSystem
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationInventoryEntity getReplicationInventory(boolean includeSystem) throws ArangoException {
return replicationDriver.getReplicationInventory(getDefaultDatabase(), includeSystem);
}
/**
* @param collectionName
* @param from
* @param to
* @param chunkSize
* @param ticks
* @param clazz
* @param handler
* @throws ArangoException
* @since 1.4.0
*/
public void getReplicationDump(
String collectionName,
Long from,
Long to,
Integer chunkSize,
Boolean ticks,
Class clazz,
DumpHandler handler) throws ArangoException {
replicationDriver.getReplicationDump(
getDefaultDatabase(),
collectionName,
from,
to,
chunkSize,
ticks,
clazz,
handler);
}
/**
* @param endpoint
* @param database
* @param username
* @param password
* @param restrictType
* @param restrictCollections
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationSyncEntity syncReplication(
String endpoint,
String database,
String username,
String password,
RestrictType restrictType,
String... restrictCollections) throws ArangoException {
return replicationDriver.syncReplication(
getDefaultDatabase(),
endpoint,
database,
username,
password,
restrictType,
restrictCollections);
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public String getReplicationServerId() throws ArangoException {
return replicationDriver.getReplicationServerId();
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public boolean startReplicationLogger() throws ArangoException {
return replicationDriver.startReplicationLogger(getDefaultDatabase());
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public boolean stopReplicationLogger() throws ArangoException {
return replicationDriver.stopReplicationLogger(getDefaultDatabase());
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationLoggerStateEntity getReplicationLoggerState() throws ArangoException {
return replicationDriver.getReplicationLoggerState(getDefaultDatabase());
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationLoggerConfigEntity getReplicationLoggerConfig() throws ArangoException {
return replicationDriver.getReplicationLoggerConfig(getDefaultDatabase());
}
/**
* @param autoStart
* @param logRemoteChanges
* @param maxEvents
* @param maxEventsSize
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationLoggerConfigEntity setReplicationLoggerConfig(
Boolean autoStart,
Boolean logRemoteChanges,
Long maxEvents,
Long maxEventsSize) throws ArangoException {
return replicationDriver.setReplicationLoggerConfig(
getDefaultDatabase(),
autoStart,
logRemoteChanges,
maxEvents,
maxEventsSize);
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationApplierConfigEntity getReplicationApplierConfig() throws ArangoException {
return replicationDriver.getReplicationApplierConfig(getDefaultDatabase());
}
/**
* @param endpoint
* @param database
* @param username
* @param password
* @param maxConnectRetries
* @param connectTimeout
* @param requestTimeout
* @param chunkSize
* @param autoStart
* @param adaptivePolling
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationApplierConfigEntity setReplicationApplierConfig(
String endpoint,
String database,
String username,
String password,
Integer maxConnectRetries,
Integer connectTimeout,
Integer requestTimeout,
Integer chunkSize,
Boolean autoStart,
Boolean adaptivePolling) throws ArangoException {
return replicationDriver.setReplicationApplierConfig(
getDefaultDatabase(),
endpoint,
database,
username,
password,
maxConnectRetries,
connectTimeout,
requestTimeout,
chunkSize,
autoStart,
adaptivePolling);
}
/**
* @param param
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationApplierConfigEntity setReplicationApplierConfig(ReplicationApplierConfigEntity param)
throws ArangoException {
return replicationDriver.setReplicationApplierConfig(getDefaultDatabase(), param);
}
/**
* @param from
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationApplierStateEntity startReplicationApplier(Long from) throws ArangoException {
return replicationDriver.startReplicationApplier(getDefaultDatabase(), from);
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationApplierStateEntity stopReplicationApplier() throws ArangoException {
return replicationDriver.stopReplicationApplier(getDefaultDatabase());
}
/**
* @return
* @throws ArangoException
* @since 1.4.0
*/
public ReplicationApplierStateEntity getReplicationApplierState() throws ArangoException {
return replicationDriver.getReplicationApplierState(getDefaultDatabase());
}
// ***************************************
// *** end of replication ****************
// ***************************************
// ***************************************
// *** start of graph ********************
// ***************************************
/**
* Returns a GraphsEntity containing all graph as GraphEntity object of the
* default database.
*
* @return GraphsEntity
* @throws ArangoException
*/
public GraphsEntity getGraphs() throws ArangoException {
return graphDriver.getGraphs(getDefaultDatabase());
}
/**
* Creates a list of the names of all available graphs of the default
* database.
*
* @return List
* @throws ArangoException
*/
public List getGraphList() throws ArangoException {
return graphDriver.getGraphList(getDefaultDatabase());
}
/**
* Creates a graph.
*
* @param graphName
* @param edgeDefinitions
* @param orphanCollections
* @param waitForSync
* @return GraphEntity
* @throws ArangoException
*/
public GraphEntity createGraph(
String graphName,
List edgeDefinitions,
List orphanCollections,
Boolean waitForSync) throws ArangoException {
return graphDriver.createGraph(getDefaultDatabase(), graphName, edgeDefinitions, orphanCollections, waitForSync);
}
/**
* Creates an empty graph.
*
* @param graphName
* @param waitForSync
* @return GraphEntity
* @throws ArangoException
*/
public GraphEntity createGraph(String graphName, Boolean waitForSync) throws ArangoException {
return graphDriver.createGraph(getDefaultDatabase(), graphName, waitForSync);
}
/**
* Get graph object by name, including its edge definitions and vertex
* collections.
*
* @param graphName
* @return GraphEntity
* @throws ArangoException
*/
public GraphEntity getGraph(String graphName) throws ArangoException {
return graphDriver.getGraph(getDefaultDatabase(), graphName);
}
/**
* Delete a graph by its name. The collections of the graph will not be
* dropped.
*
* @param graphName
* @throws ArangoException
*/
public DeletedEntity deleteGraph(String graphName) throws ArangoException {
return graphDriver.deleteGraph(getDefaultDatabase(), graphName, false);
}
/**
* Delete a graph by its name. If dropCollections is true, all collections of
* the graph will be dropped, if they are not used in another graph.
*
* @param graphName
* @param dropCollections
* @throws ArangoException
*/
public void deleteGraph(String graphName, Boolean dropCollections) throws ArangoException {
graphDriver.deleteGraph(getDefaultDatabase(), graphName, dropCollections);
}
/**
* Returns a list of all vertex collection of a graph that are defined in the
* graphs edgeDefinitions (in "from", "to", and "orphanCollections")
*
* @param graphName
* @return List
* @throws ArangoException
*/
public List graphGetVertexCollections(String graphName) throws ArangoException {
return graphDriver.getVertexCollections(getDefaultDatabase(), graphName);
}
/**
* Removes a vertex collection from the graph and optionally deletes the
* collection, if it is not used in any other graph.
*
* @param graphName
* @param collectionName
* @param dropCollection
* @throws ArangoException
*/
public DeletedEntity graphDeleteVertexCollection(String graphName, String collectionName, Boolean dropCollection)
throws ArangoException {
return graphDriver.deleteVertexCollection(getDefaultDatabase(), graphName, collectionName, dropCollection);
}
/**
* Creates a vertex collection
*
* @param graphName
* @param collectionName
* @return GraphEntity
* @throws ArangoException
*/
public GraphEntity graphCreateVertexCollection(String graphName, String collectionName) throws ArangoException {
return graphDriver.createVertexCollection(getDefaultDatabase(), graphName, collectionName);
}
/**
* Returns a list of all edge collection of a graph that are defined in the
* graphs edgeDefinitions
*
* @param graphName
* @return List
* @throws ArangoException
*/
public List graphGetEdgeCollections(String graphName) throws ArangoException {
return graphDriver.getEdgeCollections(getDefaultDatabase(), graphName);
}
/**
* Adds a new edge definition to an existing graph
*
* @param graphName
* @param edgeDefinition
* @return GraphEntity
* @throws ArangoException
*/
public GraphEntity graphCreateEdgeDefinition(String graphName, EdgeDefinitionEntity edgeDefinition)
throws ArangoException {
return graphDriver.createEdgeDefinition(getDefaultDatabase(), graphName, edgeDefinition);
}
/**
* Replaces an existing edge definition to an existing graph. This will also
* change the edge definitions of all other graphs using this definition as
* well.
*
* @param graphName
* @param edgeCollectionName
* @param edgeDefinition
* @return GraphEntity
* @throws ArangoException
*/
public GraphEntity graphReplaceEdgeDefinition(
String graphName,
String edgeCollectionName,
EdgeDefinitionEntity edgeDefinition) throws ArangoException {
return graphDriver.replaceEdgeDefinition(getDefaultDatabase(), graphName, edgeCollectionName, edgeDefinition);
}
/**
* Removes an existing edge definition from this graph. All data stored in the
* collections is dropped as well as long as it is not used in other graphs.
*
* @param graphName
* @param edgeCollectionName
* @return
*/
public GraphEntity graphDeleteEdgeDefinition(String graphName, String edgeCollectionName, Boolean dropCollection)
throws ArangoException {
return graphDriver.deleteEdgeDefinition(getDefaultDatabase(), graphName, edgeCollectionName, dropCollection);
}
/**
* Stores a new vertex with the information contained within the document into
* the given collection.
*
* @param graphName
* @param vertex
* @param waitForSync
* @return DocumentEntity
* @throws ArangoException
*/
public DocumentEntity
graphCreateVertex(String graphName, String collectionName, T vertex, Boolean waitForSync) throws ArangoException {
return graphDriver.createVertex(getDefaultDatabase(), graphName, collectionName, vertex, waitForSync);
}
/**
* Gets a vertex with the given key if it is contained within your graph.
*
* @param graphName
* @param collectionName
* @param key
* @param clazz
* @return DocumentEntity
* @throws ArangoException
*/
public DocumentEntity graphGetVertex(String graphName, String collectionName, String key, Class> clazz)
throws ArangoException {
return graphDriver.getVertex(getDefaultDatabase(), graphName, collectionName, key, clazz, null, null, null);
}
/**
* Gets a vertex with the given key if it is contained within your graph.
*
* @param graphName
* @param collectionName
* @param key
* @param clazz
* @param rev
* @param ifNoneMatchRevision
* @param ifMatchRevision
* @return DocumentEntity
* @throws ArangoException
*/
public DocumentEntity graphGetVertex(
String graphName,
String collectionName,
String key,
Class> clazz,
Long rev,
Long ifNoneMatchRevision,
Long ifMatchRevision) throws ArangoException {
return graphDriver.getVertex(
getDefaultDatabase(),
graphName,
collectionName,
key,
clazz,
rev,
ifNoneMatchRevision,
ifMatchRevision);
}
/**
* Deletes a vertex with the given key, if it is contained within the graph.
* Furthermore all edges connected to this vertex will be deleted.
*
* @param graphName
* @param collectionName
* @param key
* @return DeletedEntity
* @throws ArangoException
*/
public DeletedEntity graphDeleteVertex(String graphName, String collectionName, String key) throws ArangoException {
return graphDriver.deleteVertex(getDefaultDatabase(), graphName, collectionName, key, null, null, null);
}
/**
* Deletes a vertex with the given key, if it is contained within the graph.
* Furthermore all edges connected to this vertex will be deleted.
*
* @param graphName
* @param collectionName
* @param key
* @param waitForSync
* @return DeletedEntity
* @throws ArangoException
*/
public DeletedEntity graphDeleteVertex(String graphName, String collectionName, String key, Boolean waitForSync)
throws ArangoException {
return graphDriver.deleteVertex(getDefaultDatabase(), graphName, collectionName, key, waitForSync, null, null);
}
/**
* Deletes a vertex with the given key, if it is contained within the graph.
* Furthermore all edges connected to this vertex will be deleted.
*
* @param graphName
* @param collectionName
* @param key
* @param waitForSync
* @param rev
* @param ifMatchRevision
* @return DeletedEntity
* @throws ArangoException
*/
public DeletedEntity graphDeleteVertex(
String graphName,
String collectionName,
String key,
Boolean waitForSync,
Long rev,
Long ifMatchRevision) throws ArangoException {
return graphDriver.deleteVertex(
getDefaultDatabase(),
graphName,
collectionName,
key,
waitForSync,
rev,
ifMatchRevision);
}
/**
* Replaces a vertex with the given key by the content in the body. This will
* only run successfully if the vertex is contained within the graph.
*
* @param graphName
* @param collectionName
* @param key
* @param vertex
* @return DocumentEntity
* @throws ArangoException
*/
public DocumentEntity graphReplaceVertex(String graphName, String collectionName, String key, Object vertex)
throws ArangoException {
return graphDriver.replaceVertex(getDefaultDatabase(), graphName, collectionName, key, vertex, null, null, null);
}
/**
* Replaces a vertex with the given key by the content in the body. This will
* only run successfully if the vertex is contained within the graph.
*
* @param graphName
* @param collectionName
* @param key
* @param vertex
* @param waitForSync
* @param rev
* @param ifMatchRevision
* @return DocumentEntity
* @throws ArangoException
*/
public DocumentEntity graphReplaceVertex(
String graphName,
String collectionName,
String key,
Object vertex,
Boolean waitForSync,
Long rev,
Long ifMatchRevision) throws ArangoException {
return graphDriver.replaceVertex(
getDefaultDatabase(),
graphName,
collectionName,
key,
vertex,
waitForSync,
rev,
ifMatchRevision);
}
/**
* Updates a vertex with the given key by adding the content in the body. This
* will only run successfully if the vertex is contained within the graph.
*
* @param graphName
* @param collectionName
* @param key
* @param vertex
* @param keepNull
* @return DocumentEntity
* @throws ArangoException
*/
public DocumentEntity graphUpdateVertex(
String graphName,
String collectionName,
String key,
Object vertex,
Boolean keepNull) throws ArangoException {
return graphDriver.updateVertex(
getDefaultDatabase(),
graphName,
collectionName,
key,
vertex,
keepNull,
null,
null,
null);
}
/**
* Updates a vertex with the given key by adding the content in the body. This
* will only run successfully if the vertex is contained within the graph.
*
* @param graphName
* @param collectionName
* @param key
* @param vertex
* @param keepNull
* @param waitForSync
* @param rev
* @param ifMatchRevision
* @return DocumentEntity
* @throws ArangoException
*/
public DocumentEntity graphUpdateVertex(
String graphName,
String collectionName,
String key,
Object vertex,
Boolean keepNull,
Boolean waitForSync,
Long rev,
Long ifMatchRevision) throws ArangoException {
return graphDriver.updateVertex(
getDefaultDatabase(),
graphName,
collectionName,
key,
vertex,
keepNull,
waitForSync,
rev,
ifMatchRevision);
}
/**
* Stores a new edge with the information contained within the body into the
* given collection.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param fromHandle
* @param toHandle
* @param value
* @param label
* @param waitForSync
* @return EdgeEntity
* @throws ArangoException
*/
public EdgeEntity graphCreateEdge(
String graphName,
String edgeCollectionName,
String key,
String fromHandle,
String toHandle,
Object value,
Boolean waitForSync) throws ArangoException {
return graphDriver.createEdge(
getDefaultDatabase(),
graphName,
edgeCollectionName,
key,
fromHandle,
toHandle,
value,
waitForSync);
}
/**
* Stores a new edge with the information contained within the body into the
* given collection.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param fromHandle
* @param toHandle
* @return EdgeEntity
* @throws ArangoException
*/
public EdgeEntity graphCreateEdge(
String graphName,
String edgeCollectionName,
String key,
String fromHandle,
String toHandle) throws ArangoException {
return graphDriver.createEdge(
getDefaultDatabase(),
graphName,
edgeCollectionName,
key,
fromHandle,
toHandle,
null,
null);
}
/**
* Loads an edge with the given key if it is contained within your graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param clazz
* @param rev
* @param ifNoneMatchRevision
* @param ifMatchRevision
* @return EdgeEntity
* @throws ArangoException
*/
public EdgeEntity graphGetEdge(
String graphName,
String edgeCollectionName,
String key,
Class> clazz,
Long rev,
Long ifNoneMatchRevision,
Long ifMatchRevision) throws ArangoException {
return graphDriver.getEdge(
getDefaultDatabase(),
graphName,
edgeCollectionName,
key,
clazz,
rev,
ifNoneMatchRevision,
ifMatchRevision);
}
/**
* Loads an edge with the given key if it is contained within your graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param clazz
* @return EdgeEntity
* @throws ArangoException
*/
public EdgeEntity graphGetEdge(String graphName, String edgeCollectionName, String key, Class> clazz)
throws ArangoException {
return graphDriver.getEdge(getDefaultDatabase(), graphName, edgeCollectionName, key, clazz, null, null, null);
}
/**
* Deletes an edge with the given id, if it is contained within the graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @return
* @throws ArangoException
*/
public DeletedEntity graphDeleteEdge(String graphName, String edgeCollectionName, String key) throws ArangoException {
return graphDriver.deleteEdge(getDefaultDatabase(), graphName, edgeCollectionName, key, null, null, null);
}
/**
* Deletes an edge with the given id, if it is contained within the graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param waitForSync
* @return
* @throws ArangoException
*/
public DeletedEntity graphDeleteEdge(String graphName, String edgeCollectionName, String key, Boolean waitForSync)
throws ArangoException {
return graphDriver.deleteEdge(getDefaultDatabase(), graphName, edgeCollectionName, key, waitForSync, null, null);
}
/**
* Deletes an edge with the given id, if it is contained within the graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param waitForSync
* @param rev
* @param ifMatchRevision
* @return
* @throws ArangoException
*/
public DeletedEntity graphDeleteEdge(
String graphName,
String edgeCollectionName,
String key,
Boolean waitForSync,
Long rev,
Long ifMatchRevision) throws ArangoException {
return graphDriver.deleteEdge(
getDefaultDatabase(),
graphName,
edgeCollectionName,
key,
waitForSync,
rev,
ifMatchRevision);
}
/**
* Replaces an edge with the given key by the content in the body. This will
* only run successfully if the edge is contained within the graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param value
* @return
* @throws ArangoException
*/
public EdgeEntity graphReplaceEdge(String graphName, String edgeCollectionName, String key, Object value)
throws ArangoException {
return graphDriver.replaceEdge(getDefaultDatabase(), graphName, edgeCollectionName, key, value, null, null, null);
}
/**
* Replaces an edge with the given key by the content in the body. This will
* only run successfully if the edge is contained within the graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param value
* @param waitForSync
* @param rev
* @param ifMatchRevision
* @return
* @throws ArangoException
*/
public EdgeEntity graphReplaceEdge(
String graphName,
String edgeCollectionName,
String key,
Object value,
Boolean waitForSync,
Long rev,
Long ifMatchRevision) throws ArangoException {
return graphDriver.replaceEdge(
getDefaultDatabase(),
graphName,
edgeCollectionName,
key,
value,
waitForSync,
rev,
ifMatchRevision);
}
/**
* Updates an edge with the given key by adding the content in the body. This
* will only run successfully if the edge is contained within the graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param value
* @param keepNull
* @return
* @throws ArangoException
*/
public EdgeEntity graphUpdateEdge(
String graphName,
String edgeCollectionName,
String key,
Object value,
Boolean keepNull) throws ArangoException {
return graphDriver.updateEdge(
getDefaultDatabase(),
graphName,
edgeCollectionName,
key,
value,
null,
keepNull,
null,
null);
}
/**
* Updates an edge with the given key by adding the content in the body. This
* will only run successfully if the edge is contained within the graph.
*
* @param graphName
* @param edgeCollectionName
* @param key
* @param value
* @param waitForSync
* @param keepNull
* @param rev
* @param ifMatchRevision
* @return
* @throws ArangoException
*/
public EdgeEntity graphUpdateEdge(
String graphName,
String edgeCollectionName,
String key,
Object value,
Boolean waitForSync,
Boolean keepNull,
Long rev,
Long ifMatchRevision) throws ArangoException {
return graphDriver.updateEdge(
getDefaultDatabase(),
graphName,
edgeCollectionName,
key,
value,
waitForSync,
keepNull,
rev,
ifMatchRevision);
}
// Some methods not using the graph api
/**
* Returns all Edges of a graph, each edge as a PlainEdgeEntity.
*
* @param graphName
* @return CursorEntity
* @throws ArangoException
*/
public CursorEntity graphGetEdges(String graphName) throws ArangoException {
validateCollectionName(graphName);
String query = "for i in graph_edges(@graphName, null) return i";
Map bindVars = new MapBuilder().put("graphName", graphName).get();
CursorEntity result = this.executeQuery(query, bindVars, PlainEdgeEntity.class, true, 20);
return result;
}
/**
* Returns all Edges of a given vertex.
*
* @param graphName
* @param clazz
* @param vertexDocumentHandle
* @return CursorEntity
* @throws ArangoException
*/
public CursorEntity graphGetEdges(String graphName, Class clazz, String vertexDocumentHandle)
throws ArangoException {
validateCollectionName(graphName);
String query = "for i in graph_edges(@graphName, @vertexDocumentHandle) return i";
Map bindVars = new MapBuilder().put("graphName", graphName)
.put("vertexDocumentHandle", vertexDocumentHandle).get();
CursorEntity result = this.executeQuery(query, bindVars, clazz, true, 20);
return result;
}
/**
* Returns all Edges of vertices matching the example object (non-primitive
* set to null will not be used for comparing).
*
* @param graphName
* @param clazzT
* @param vertexExample
* @return CursorEntity
* @throws ArangoException
*/
public CursorEntity graphGetEdgesByExampleObject(String graphName, Class clazzT, S vertexExample)
throws ArangoException {
validateCollectionName(graphName);
String query = "for i in graph_edges(@graphName, @vertexExample) return i";
Map bindVars = new MapBuilder().put("graphName", graphName).put("vertexExample", vertexExample)
.get();
CursorEntity result = this.executeQuery(query, bindVars, clazzT, true, 20);
return result;
}
/**
* Returns all Edges of vertices matching the map.
*
* @param graphName
* @param clazzT
* @param vertexExample
* @return CursorEntity
* @throws ArangoException
*/
public CursorEntity graphGetEdgesByExampleMap(
String graphName,
Class clazzT,
Map vertexExample) throws ArangoException {
validateCollectionName(graphName);
String query = "for i in graph_edges(@graphName, @vertexExample) return i";
Map bindVars = new MapBuilder().put("graphName", graphName).put("vertexExample", vertexExample)
.get();
CursorEntity result = this.executeQuery(query, bindVars, clazzT, true, 20);
return result;
}
// public CursorEntity> graphGetEdgesByExampleObject1(
// String graphName,
// Class clazzT,
// S vertexExample) throws ArangoException {
// validateCollectionName(graphName);
// String query = "for i in graph_edges(@graphName, @vertexExample) return i";
//
// Map bindVars = new MapBuilder().put("graphName",
// graphName).put("vertexExample", vertexExample)
// .get();
//
// CursorEntity> result = this.executeQuery(query, bindVars,
// EdgeEntity.class, true, 20);
//
// return null;
// }
// public CursorEntity> graphGetEdgesWithData(
// String graphName,
// Class clazz,
// String vertexDocumentHandle,
// int i) throws ArangoException {
//
// validateCollectionName(graphName);
// String query =
// "for i in graph_edges(@graphName, @vertexDocumentHandle) return i";
// Map bindVars = new MapBuilder().put("graphName", graphName)
// .put("vertexDocumentHandle", vertexDocumentHandle).get();
//
// CursorEntity result = this.executeQuery(query, bindVars, clazz, true,
// 20);
//
// return (CursorEntity>) result;
//
// }
// ***************************************
// *** end of graph **********************
// ***************************************
public DefaultEntity createAqlFunction(String name, String code) throws ArangoException {
return aqlFunctionsDriver.createAqlFunction(name, code);
}
public AqlFunctionsEntity getAqlFunctions(String namespace) throws ArangoException {
return aqlFunctionsDriver.getAqlFunctions(namespace);
}
public DefaultEntity deleteAqlFunction(String name, boolean isNameSpace) throws ArangoException {
return aqlFunctionsDriver.deleteAqlFunction(name, isNameSpace);
}
public TransactionEntity createTransaction(String action) {
return this.transactionDriver.createTransaction(action);
};
public T executeTransaction(TransactionEntity transactionEntity, Class clazz) throws ArangoException {
return this.transactionDriver.executeTransaction(getDefaultDatabase(), transactionEntity, clazz);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy