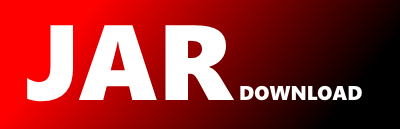
com.arangodb.InternalSimpleDriver Maven / Gradle / Ivy
package com.arangodb;
import java.util.Map;
import com.arangodb.entity.*;
import com.arangodb.impl.BaseDriverInterface;
/**
* Created by fbartels on 10/27/14.
*/
public interface InternalSimpleDriver extends BaseDriverInterface {
CursorEntity executeSimpleAll(
String database,
String collectionName, int skip, int limit,
Class> clazz) throws ArangoException;
CursorResultSet executeSimpleAllWithResultSet(
String database,
String collectionName, int skip, int limit,
Class> clazz) throws ArangoException;
CursorEntity> executeSimpleAllWithDocument(
String database,
String collectionName, int skip, int limit,
Class> clazz) throws ArangoException;
CursorResultSet> executeSimpleAllWithDocumentResultSet(
String database,
String collectionName, int skip, int limit,
Class> clazz) throws ArangoException;
CursorEntity executeSimpleByExample(
String database,
String collectionName,
Map example,
int skip, int limit,
Class> clazz
) throws ArangoException;
CursorResultSet executeSimpleByExampleWithResultSet(
String database,
String collectionName, Map example,
int skip, int limit,
Class> clazz
) throws ArangoException;
CursorEntity> executeSimpleByExampleWithDocument(
String database,
String collectionName,
Map example,
int skip, int limit,
Class> clazz
) throws ArangoException;
CursorResultSet> executeSimpleByExampleWithDocumentResultSet(
String database,
String collectionName, Map example,
int skip, int limit,
Class> clazz
) throws ArangoException;
ScalarExampleEntity executeSimpleFirstExample(
String database,
String collectionName,
Map example,
Class> clazz
) throws ArangoException;
ScalarExampleEntity executeSimpleAny(
String database,
String collectionName,
Class> clazz
) throws ArangoException;
CursorEntity executeSimpleRange(
String database,
String collectionName,
String attribute,
Object left, Object right, Boolean closed,
int skip, int limit,
Class> clazz
) throws ArangoException;
CursorResultSet executeSimpleRangeWithResultSet(
String database,
String collectionName,
String attribute,
Object left, Object right, Boolean closed,
int skip, int limit,
Class> clazz
) throws ArangoException;
CursorEntity> executeSimpleRangeWithDocument(
String database,
String collectionName,
String attribute,
Object left, Object right, Boolean closed,
int skip, int limit,
Class> clazz
) throws ArangoException;
CursorResultSet> executeSimpleRangeWithDocumentResultSet(
String database,
String collectionName,
String attribute,
Object left, Object right, Boolean closed,
int skip, int limit,
Class> clazz
) throws ArangoException;
SimpleByResultEntity executeSimpleRemoveByExample(
String database,
String collectionName,
Map example,
Boolean waitForSync,
Integer limit) throws ArangoException;
SimpleByResultEntity executeSimpleReplaceByExample(
String database,
String collectionName,
Map example,
Map newValue,
Boolean waitForSync,
Integer limit) throws ArangoException;
SimpleByResultEntity executeSimpleUpdateByExample(
String database,
String collectionName,
Map example,
Map newValue,
Boolean keepNull,
Boolean waitForSync,
Integer limit) throws ArangoException;
CursorEntity executeSimpleFulltext(
String database,
String collectionName,
String attribute, String query,
int skip, int limit,
String index,
Class> clazz
) throws ArangoException;
CursorResultSet executeSimpleFulltextWithResultSet(
String database,
String collectionName,
String attribute, String query,
int skip, int limit,
String index,
Class> clazz
) throws ArangoException;
CursorEntity> executeSimpleFulltextWithDocument(
String database,
String collectionName,
String attribute, String query,
int skip, int limit,
String index,
Class> clazz
) throws ArangoException;
CursorResultSet> executeSimpleFulltextWithDocumentResultSet(
String database,
String collectionName,
String attribute, String query,
int skip, int limit,
String index,
Class> clazz
) throws ArangoException;
DocumentResultEntity executeSimpleFirst(
String database,
String collectionName,
Integer count,
Class> clazz) throws ArangoException;
DocumentResultEntity executeSimpleLast(
String database,
String collectionName,
Integer count,
Class> clazz) throws ArangoException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy