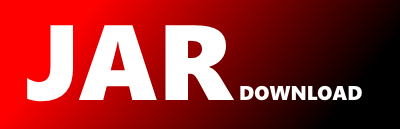
com.arangodb.ArangoCollection Maven / Gradle / Ivy
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb;
import java.util.Collection;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.arangodb.entity.CollectionEntity;
import com.arangodb.entity.CollectionPropertiesEntity;
import com.arangodb.entity.CollectionRevisionEntity;
import com.arangodb.entity.DocumentCreateEntity;
import com.arangodb.entity.DocumentDeleteEntity;
import com.arangodb.entity.DocumentUpdateEntity;
import com.arangodb.entity.IndexEntity;
import com.arangodb.entity.MultiDocumentEntity;
import com.arangodb.internal.InternalArangoCollection;
import com.arangodb.internal.ArangoExecutorSync;
import com.arangodb.internal.velocystream.ConnectionSync;
import com.arangodb.model.CollectionPropertiesOptions;
import com.arangodb.model.DocumentCreateOptions;
import com.arangodb.model.DocumentDeleteOptions;
import com.arangodb.model.DocumentExistsOptions;
import com.arangodb.model.DocumentReadOptions;
import com.arangodb.model.DocumentReplaceOptions;
import com.arangodb.model.DocumentUpdateOptions;
import com.arangodb.model.FulltextIndexOptions;
import com.arangodb.model.GeoIndexOptions;
import com.arangodb.model.HashIndexOptions;
import com.arangodb.model.PersistentIndexOptions;
import com.arangodb.model.SkiplistIndexOptions;
import com.arangodb.velocystream.Response;
/**
* @author Mark - mark at arangodb.com
*
*/
public class ArangoCollection extends InternalArangoCollection {
private static final Logger LOGGER = LoggerFactory.getLogger(ArangoCollection.class);
protected ArangoCollection(final ArangoDatabase db, final String name) {
super(db.executor(), db.name(), name);
}
/**
* Creates a new document from the given document, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @see API
* Documentation
* @param value
* A representation of a single document (POJO, VPackSlice or String for Json)
* @return information about the document
* @throws ArangoDBException
*/
public DocumentCreateEntity insertDocument(final T value) throws ArangoDBException {
return executor.execute(insertDocumentRequest(value, new DocumentCreateOptions()),
insertDocumentResponseDeserializer(value));
}
/**
* Creates a new document from the given document, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @see API
* Documentation
* @param value
* A representation of a single document (POJO, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return information about the document
* @throws ArangoDBException
*/
public DocumentCreateEntity insertDocument(final T value, final DocumentCreateOptions options)
throws ArangoDBException {
return executor.execute(insertDocumentRequest(value, options), insertDocumentResponseDeserializer(value));
}
/**
* Creates new documents from the given documents, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @see API
* Documentation
* @param values
* A List of documents (POJO, VPackSlice or String for Json)
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> insertDocuments(final Collection values)
throws ArangoDBException {
final DocumentCreateOptions params = new DocumentCreateOptions();
return executor.execute(insertDocumentsRequest(values, params),
insertDocumentsResponseDeserializer(values, params));
}
/**
* Creates new documents from the given documents, unless there is already a document with the _key given. If no
* _key is given, a new unique _key is generated automatically.
*
* @see API
* Documentation
* @param values
* A List of documents (POJO, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> insertDocuments(
final Collection values,
final DocumentCreateOptions options) throws ArangoDBException {
final DocumentCreateOptions params = (options != null ? options : new DocumentCreateOptions());
return executor.execute(insertDocumentsRequest(values, params),
insertDocumentsResponseDeserializer(values, params));
}
/**
* Reads a single document
*
* @see API
* Documentation
* @param key
* The key of the document
* @param type
* The type of the document (POJO class, VPackSlice or String for Json)
* @return the document identified by the key
* @throws ArangoDBException
*/
public T getDocument(final String key, final Class type) {
executor.validateDocumentKey(key);
try {
return executor.execute(getDocumentRequest(key, new DocumentReadOptions()), type);
} catch (final ArangoDBException e) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug(e.getMessage(), e);
}
return null;
}
}
/**
* Reads a single document
*
* @see API
* Documentation
* @param key
* The key of the document
* @param type
* The type of the document (POJO class, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return the document identified by the key
* @throws ArangoDBException
*/
public T getDocument(final String key, final Class type, final DocumentReadOptions options)
throws ArangoDBException {
executor.validateDocumentKey(key);
try {
return executor.execute(getDocumentRequest(key, options), type);
} catch (final ArangoDBException e) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug(e.getMessage(), e);
}
return null;
}
}
/**
* Replaces the document with key with the one in the body, provided there is such a document and no precondition is
* violated
*
* @see API
* Documentation
* @param key
* The key of the document
* @param value
* A representation of a single document (POJO, VPackSlice or String for Json)
* @return information about the document
* @throws ArangoDBException
*/
public DocumentUpdateEntity replaceDocument(final String key, final T value) throws ArangoDBException {
return executor.execute(replaceDocumentRequest(key, value, new DocumentReplaceOptions()),
replaceDocumentResponseDeserializer(value));
}
/**
* Replaces the document with key with the one in the body, provided there is such a document and no precondition is
* violated
*
* @see API
* Documentation
* @param key
* The key of the document
* @param value
* A representation of a single document (POJO, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return information about the document
* @throws ArangoDBException
*/
public DocumentUpdateEntity replaceDocument(
final String key,
final T value,
final DocumentReplaceOptions options) throws ArangoDBException {
return executor.execute(replaceDocumentRequest(key, value, options),
replaceDocumentResponseDeserializer(value));
}
/**
* Replaces multiple documents in the specified collection with the ones in the values, the replaced documents are
* specified by the _key attributes in the documents in values.
*
* @see API
* Documentation
* @param values
* A List of documents (POJO, VPackSlice or String for Json)
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> replaceDocuments(final Collection values)
throws ArangoDBException {
final DocumentReplaceOptions params = new DocumentReplaceOptions();
return executor.execute(replaceDocumentsRequest(values, params),
replaceDocumentsResponseDeserializer(values, params));
}
/**
* Replaces multiple documents in the specified collection with the ones in the values, the replaced documents are
* specified by the _key attributes in the documents in values.
*
* @see API
* Documentation
* @param values
* A List of documents (POJO, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> replaceDocuments(
final Collection values,
final DocumentReplaceOptions options) throws ArangoDBException {
final DocumentReplaceOptions params = (options != null ? options : new DocumentReplaceOptions());
return executor.execute(replaceDocumentsRequest(values, params),
replaceDocumentsResponseDeserializer(values, params));
}
/**
* Partially updates the document identified by document-key. The value must contain a document with the attributes
* to patch (the patch document). All attributes from the patch document will be added to the existing document if
* they do not yet exist, and overwritten in the existing document if they do exist there.
*
* @see API
* Documentation
* @param key
* The key of the document
* @param value
* A representation of a single document (POJO, VPackSlice or String for Json)
* @return information about the document
* @throws ArangoDBException
*/
public DocumentUpdateEntity updateDocument(final String key, final T value) throws ArangoDBException {
return executor.execute(updateDocumentRequest(key, value, new DocumentUpdateOptions()),
updateDocumentResponseDeserializer(value));
}
/**
* Partially updates the document identified by document-key. The value must contain a document with the attributes
* to patch (the patch document). All attributes from the patch document will be added to the existing document if
* they do not yet exist, and overwritten in the existing document if they do exist there.
*
* @see API
* Documentation
* @param key
* The key of the document
* @param value
* A representation of a single document (POJO, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return information about the document
* @throws ArangoDBException
*/
public DocumentUpdateEntity updateDocument(
final String key,
final T value,
final DocumentUpdateOptions options) throws ArangoDBException {
return executor.execute(updateDocumentRequest(key, value, options), updateDocumentResponseDeserializer(value));
}
/**
* Partially updates documents, the documents to update are specified by the _key attributes in the objects on
* values. Vales must contain a list of document updates with the attributes to patch (the patch documents). All
* attributes from the patch documents will be added to the existing documents if they do not yet exist, and
* overwritten in the existing documents if they do exist there.
*
* @see API
* Documentation
* @param values
* A list of documents (POJO, VPackSlice or String for Json)
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> updateDocuments(final Collection values)
throws ArangoDBException {
final DocumentUpdateOptions params = new DocumentUpdateOptions();
return executor.execute(updateDocumentsRequest(values, params),
updateDocumentsResponseDeserializer(values, params));
}
/**
* Partially updates documents, the documents to update are specified by the _key attributes in the objects on
* values. Vales must contain a list of document updates with the attributes to patch (the patch documents). All
* attributes from the patch documents will be added to the existing documents if they do not yet exist, and
* overwritten in the existing documents if they do exist there.
*
* @see API
* Documentation
* @param values
* A list of documents (POJO, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> updateDocuments(
final Collection values,
final DocumentUpdateOptions options) throws ArangoDBException {
final DocumentUpdateOptions params = (options != null ? options : new DocumentUpdateOptions());
return executor.execute(updateDocumentsRequest(values, params),
updateDocumentsResponseDeserializer(values, params));
}
/**
* Removes a document
*
* @see API
* Documentation
* @param key
* The key of the document
* @param type
* The type of the document (POJO class, VPackSlice or String for Json). Only necessary if
* options.returnOld is set to true, otherwise can be null.
* @param options
* Additional options, can be null
* @return information about the document
* @throws ArangoDBException
*/
public DocumentDeleteEntity deleteDocument(final String key) throws ArangoDBException {
return executor.execute(deleteDocumentRequest(key, new DocumentDeleteOptions()),
deleteDocumentResponseDeserializer(Void.class));
}
/**
* Removes a document
*
* @see API
* Documentation
* @param key
* The key of the document
* @param type
* The type of the document (POJO class, VPackSlice or String for Json). Only necessary if
* options.returnOld is set to true, otherwise can be null.
* @param options
* Additional options, can be null
* @return information about the document
* @throws ArangoDBException
*/
public DocumentDeleteEntity deleteDocument(
final String key,
final Class type,
final DocumentDeleteOptions options) throws ArangoDBException {
return executor.execute(deleteDocumentRequest(key, options), deleteDocumentResponseDeserializer(type));
}
/**
* Removes multiple document
*
* @see API
* Documentation
* @param keys
* The keys of the documents
* @param type
* The type of the documents (POJO class, VPackSlice or String for Json). Only necessary if
* options.returnOld is set to true, otherwise can be null.
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> deleteDocuments(final Collection keys)
throws ArangoDBException {
return executor.execute(deleteDocumentsRequest(keys, new DocumentDeleteOptions()),
deleteDocumentsResponseDeserializer(Void.class));
}
/**
* Removes multiple document
*
* @see API
* Documentation
* @param keys
* The keys of the documents
* @param type
* The type of the documents (POJO class, VPackSlice or String for Json). Only necessary if
* options.returnOld is set to true, otherwise can be null.
* @param options
* Additional options, can be null
* @return information about the documents
* @throws ArangoDBException
*/
public MultiDocumentEntity> deleteDocuments(
final Collection keys,
final Class type,
final DocumentDeleteOptions options) throws ArangoDBException {
return executor.execute(deleteDocumentsRequest(keys, options), deleteDocumentsResponseDeserializer(type));
}
/**
* Checks if the document exists by reading a single document head
*
* @see API
* Documentation
* @param key
* The key of the document
* @return true if the document was found, otherwise false
*/
public Boolean documentExists(final String key) {
return documentExists(key, new DocumentExistsOptions());
}
/**
* Checks if the document exists by reading a single document head
*
* @see API
* Documentation
* @param key
* The key of the document
* @param options
* Additional options, can be null
* @return true if the document was found, otherwise false
*/
public Boolean documentExists(final String key, final DocumentExistsOptions options) {
try {
executor.communication().execute(documentExistsRequest(key, options));
return true;
} catch (final ArangoDBException e) {
return false;
}
}
/**
* Creates a hash index for the collection if it does not already exist.
*
* @see API Documentation
* @param fields
* A list of attribute paths
* @param options
* Additional options, can be null
* @return information about the index
* @throws ArangoDBException
*/
public IndexEntity createHashIndex(final Collection fields, final HashIndexOptions options)
throws ArangoDBException {
return executor.execute(createHashIndexRequest(fields, options), IndexEntity.class);
}
/**
* Creates a skip-list index for the collection, if it does not already exist.
*
* @see API
* Documentation
* @param fields
* A list of attribute paths
* @param options
* Additional options, can be null
* @return information about the index
* @throws ArangoDBException
*/
public IndexEntity createSkiplistIndex(final Collection fields, final SkiplistIndexOptions options)
throws ArangoDBException {
return executor.execute(createSkiplistIndexRequest(fields, options), IndexEntity.class);
}
/**
* Creates a persistent index for the collection, if it does not already exist.
*
* @see API
* Documentation
* @param fields
* A list of attribute paths
* @param options
* Additional options, can be null
* @return information about the index
* @throws ArangoDBException
*/
public IndexEntity createPersistentIndex(final Collection fields, final PersistentIndexOptions options)
throws ArangoDBException {
return executor.execute(createPersistentIndexRequest(fields, options), IndexEntity.class);
}
/**
* Creates a geo-spatial index for the collection, if it does not already exist.
*
* @see API
* Documentation
* @param fields
* A list of attribute paths
* @param options
* Additional options, can be null
* @return information about the index
* @throws ArangoDBException
*/
public IndexEntity createGeoIndex(final Collection fields, final GeoIndexOptions options)
throws ArangoDBException {
return executor.execute(createGeoIndexRequest(fields, options), IndexEntity.class);
}
/**
* Creates a fulltext index for the collection, if it does not already exist.
*
* @see API
* Documentation
* @param fields
* A list of attribute paths
* @param options
* Additional options, can be null
* @return information about the index
* @throws ArangoDBException
*/
public IndexEntity createFulltextIndex(final Collection fields, final FulltextIndexOptions options)
throws ArangoDBException {
return executor.execute(createFulltextIndexRequest(fields, options), IndexEntity.class);
}
/**
* Returns all indexes of the collection
*
* @see API
* Documentation
* @return information about the indexes
* @throws ArangoDBException
*/
public Collection getIndexes() throws ArangoDBException {
return executor.execute(getIndexesRequest(), getIndexesResponseDeserializer());
}
/**
* Removes all documents from the collection, but leaves the indexes intact
*
* @see API
* Documentation
* @return information about the collection
* @throws ArangoDBException
*/
public CollectionEntity truncate() throws ArangoDBException {
return executor.execute(truncateRequest(), CollectionEntity.class);
}
/**
* Counts the documents in a collection
*
* @see API
* Documentation
* @return information about the collection, including the number of documents
* @throws ArangoDBException
*/
public CollectionPropertiesEntity count() throws ArangoDBException {
return executor.execute(countRequest(), CollectionPropertiesEntity.class);
}
/**
* Drops the collection
*
* @see API
* Documentation
* @throws ArangoDBException
*/
public void drop() throws ArangoDBException {
executor.execute(dropRequest(), Void.class);
}
/**
* Loads a collection into memory.
*
* @see API
* Documentation
* @return information about the collection
* @throws ArangoDBException
*/
public CollectionEntity load() throws ArangoDBException {
return executor.execute(loadRequest(), CollectionEntity.class);
}
/**
* Removes a collection from memory. This call does not delete any documents. You can use the collection afterwards;
* in which case it will be loaded into memory, again.
*
* @see API
* Documentation
* @return information about the collection
* @throws ArangoDBException
*/
public CollectionEntity unload() throws ArangoDBException {
return executor.execute(unloadRequest(), CollectionEntity.class);
}
/**
* Returns information about the collection
*
* @see API
* Documentation
* @return information about the collection
* @throws ArangoDBException
*/
public CollectionEntity getInfo() throws ArangoDBException {
return executor.execute(getInfoRequest(), CollectionEntity.class);
}
/**
* Reads the properties of the specified collection
*
* @see API
* Documentation
* @return properties of the collection
* @throws ArangoDBException
*/
public CollectionPropertiesEntity getProperties() throws ArangoDBException {
return executor.execute(getPropertiesRequest(), CollectionPropertiesEntity.class);
}
/**
* Changes the properties of a collection
*
* @see API
* Documentation
* @param options
* Additional options, can be null
* @return properties of the collection
* @throws ArangoDBException
*/
public CollectionPropertiesEntity changeProperties(final CollectionPropertiesOptions options)
throws ArangoDBException {
return executor.execute(changePropertiesRequest(options), CollectionPropertiesEntity.class);
}
/**
* Renames a collection
*
* @see API
* Documentation
* @param newName
* The new name
* @return information about the collection
* @throws ArangoDBException
*/
public CollectionEntity rename(final String newName) throws ArangoDBException {
return executor.execute(renameRequest(newName), CollectionEntity.class);
}
/**
* Retrieve the collections revision
*
* @see API
* Documentation
* @return information about the collection, including the collections revision
* @throws ArangoDBException
*/
public CollectionRevisionEntity getRevision() throws ArangoDBException {
return executor.execute(getRevisionRequest(), CollectionRevisionEntity.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy