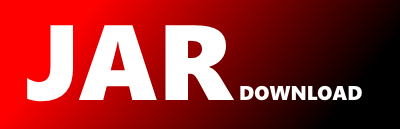
com.arangodb.ArangoDatabase Maven / Gradle / Ivy
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb;
import java.util.Collection;
import java.util.Map;
import com.arangodb.entity.AqlExecutionExplainEntity;
import com.arangodb.entity.AqlFunctionEntity;
import com.arangodb.entity.AqlParseEntity;
import com.arangodb.entity.CollectionEntity;
import com.arangodb.entity.CursorEntity;
import com.arangodb.entity.DatabaseEntity;
import com.arangodb.entity.EdgeDefinition;
import com.arangodb.entity.GraphEntity;
import com.arangodb.entity.IndexEntity;
import com.arangodb.entity.QueryCachePropertiesEntity;
import com.arangodb.entity.QueryEntity;
import com.arangodb.entity.QueryTrackingPropertiesEntity;
import com.arangodb.entity.TraversalEntity;
import com.arangodb.internal.ArangoCursorExecute;
import com.arangodb.internal.ArangoExecutorSync;
import com.arangodb.internal.CollectionCache;
import com.arangodb.internal.DocumentCache;
import com.arangodb.internal.InternalArangoDatabase;
import com.arangodb.internal.velocystream.Communication;
import com.arangodb.internal.velocystream.ConnectionSync;
import com.arangodb.model.AqlFunctionCreateOptions;
import com.arangodb.model.AqlFunctionDeleteOptions;
import com.arangodb.model.AqlFunctionGetOptions;
import com.arangodb.model.AqlQueryExplainOptions;
import com.arangodb.model.AqlQueryOptions;
import com.arangodb.model.CollectionCreateOptions;
import com.arangodb.model.CollectionsReadOptions;
import com.arangodb.model.DocumentReadOptions;
import com.arangodb.model.GraphCreateOptions;
import com.arangodb.model.TransactionOptions;
import com.arangodb.model.TraversalOptions;
import com.arangodb.velocypack.Type;
import com.arangodb.velocypack.VPack;
import com.arangodb.velocypack.VPackParser;
import com.arangodb.velocystream.Request;
import com.arangodb.velocystream.Response;
/**
* @author Mark - mark at arangodb.com
*
*/
public class ArangoDatabase extends InternalArangoDatabase {
protected ArangoDatabase(final ArangoDB arangoDB, final String name) {
super(arangoDB.executor(), name);
}
protected ArangoDatabase(final Communication communication, final VPack vpacker,
final VPack vpackerNull, final VPackParser vpackParser, final DocumentCache documentCache,
final CollectionCache collectionCache, final String name) {
super(new ArangoExecutorSync(communication, vpacker, vpackerNull, vpackParser, documentCache, collectionCache),
name);
}
protected ArangoExecutorSync executor() {
return executor;
}
/**
* Returns a handler of the collection by the given name
*
* @param name
* Name of the collection
* @return collection handler
*/
public ArangoCollection collection(final String name) {
return new ArangoCollection(this, name);
}
/**
* Creates a collection
*
* @see API
* Documentation
* @param name
* The name of the collection
* @return information about the collection
* @throws ArangoDBException
*/
public CollectionEntity createCollection(final String name) throws ArangoDBException {
return executor.execute(createCollectionRequest(name, new CollectionCreateOptions()), CollectionEntity.class);
}
/**
* Creates a collection
*
* @see API
* Documentation
* @param name
* The name of the collection
* @param options
* Additional options, can be null
* @return information about the collection
* @throws ArangoDBException
*/
public CollectionEntity createCollection(final String name, final CollectionCreateOptions options)
throws ArangoDBException {
return executor.execute(createCollectionRequest(name, options), CollectionEntity.class);
}
/**
* Returns all collections
*
* @see API
* Documentation
* @return list of information about all collections
* @throws ArangoDBException
*/
public Collection getCollections() throws ArangoDBException {
return executor.execute(getCollectionsRequest(new CollectionsReadOptions()),
getCollectionsResponseDeserializer());
}
/**
* Returns all collections
*
* @see API
* Documentation
* @param options
* Additional options, can be null
* @return list of information about all collections
* @throws ArangoDBException
*/
public Collection getCollections(final CollectionsReadOptions options) throws ArangoDBException {
return executor.execute(getCollectionsRequest(options), getCollectionsResponseDeserializer());
}
/**
* Returns an index
*
* @see API Documentation
* @param id
* The index-handle
* @return information about the index
* @throws ArangoDBException
*/
public IndexEntity getIndex(final String id) throws ArangoDBException {
return executor.execute(getIndexRequest(id), IndexEntity.class);
}
/**
* Deletes an index
*
* @see API Documentation
* @param id
* The index-handle
* @return the id of the index
* @throws ArangoDBException
*/
public String deleteIndex(final String id) throws ArangoDBException {
return executor.execute(deleteIndexRequest(id), deleteIndexResponseDeserializer());
}
/**
* Drop an existing database
*
* @see API
* Documentation
* @return true if the database was dropped successfully
* @throws ArangoDBException
*/
public Boolean drop() throws ArangoDBException {
return executor.execute(dropRequest(), createDropResponseDeserializer());
}
/**
* Grants access to the database dbname for user user. You need permission to the _system database in order to
* execute this call.
*
* @see
* API Documentation
* @param user
* The name of the user
* @throws ArangoDBException
*/
public void grantAccess(final String user) throws ArangoDBException {
executor.execute(grantAccessRequest(user), Void.class);
}
/**
* Revokes access to the database dbname for user user. You need permission to the _system database in order to
* execute this call.
*
* @see
* API Documentation
* @param user
* The name of the user
* @throws ArangoDBException
*/
public void revokeAccess(final String user) throws ArangoDBException {
executor.execute(revokeAccessRequest(user), Void.class);
}
/**
* Create a cursor and return the first results
*
* @see API
* Documentation
* @param query
* contains the query string to be executed
* @param bindVars
* key/value pairs representing the bind parameters
* @param options
* Additional options, can be null
* @param type
* The type of the result (POJO class, VPackSlice, String for Json, or Collection/List/Map)
* @return cursor of the results
* @throws ArangoDBException
*/
public ArangoCursor query(
final String query,
final Map bindVars,
final AqlQueryOptions options,
final Class type) throws ArangoDBException {
final Request request = queryRequest(query, bindVars, options);
final CursorEntity result = executor.execute(request, CursorEntity.class);
return new ArangoCursor(this, new ArangoCursorExecute() {
@Override
public CursorEntity next(final String id) {
return executor.execute(queryNextRequest(id), CursorEntity.class);
}
@Override
public void close(final String id) {
executor.execute(queryCloseRequest(id), Void.class);
}
}, type, result);
}
/**
* Explain an AQL query and return information about it
*
* @see API
* Documentation
* @param query
* the query which you want explained
* @param bindVars
* key/value pairs representing the bind parameters
* @param options
* Additional options, can be null
* @return information about the query
* @throws ArangoDBException
*/
public AqlExecutionExplainEntity explainQuery(
final String query,
final Map bindVars,
final AqlQueryExplainOptions options) throws ArangoDBException {
return executor.execute(explainQueryRequest(query, bindVars, options), AqlExecutionExplainEntity.class);
}
/**
* Parse an AQL query and return information about it This method is for query validation only. To actually query
* the database, see {@link ArangoDatabase#query(String, Map, AqlQueryOptions, Class)}
*
* @see API
* Documentation
* @param query
* the query which you want parse
* @return imformation about the query
* @throws ArangoDBException
*/
public AqlParseEntity parseQuery(final String query) throws ArangoDBException {
return executor.execute(parseQueryRequest(query), AqlParseEntity.class);
}
/**
* Clears the AQL query cache
*
* @see API
* Documentation
* @throws ArangoDBException
*/
public void clearQueryCache() throws ArangoDBException {
executor.execute(clearQueryCacheRequest(), Void.class);
}
/**
* Returns the global configuration for the AQL query cache
*
* @see API
* Documentation
* @return configuration for the AQL query cache
* @throws ArangoDBException
*/
public QueryCachePropertiesEntity getQueryCacheProperties() throws ArangoDBException {
return executor.execute(getQueryCachePropertiesRequest(), QueryCachePropertiesEntity.class);
}
/**
* Changes the configuration for the AQL query cache. Note: changing the properties may invalidate all results in
* the cache.
*
* @see API
* Documentation
* @param properties
* properties to be set
* @return current set of properties
* @throws ArangoDBException
*/
public QueryCachePropertiesEntity setQueryCacheProperties(final QueryCachePropertiesEntity properties)
throws ArangoDBException {
return executor.execute(setQueryCachePropertiesRequest(properties), QueryCachePropertiesEntity.class);
}
/**
* Returns the configuration for the AQL query tracking
*
* @see API
* Documentation
* @return configuration for the AQL query tracking
* @throws ArangoDBException
*/
public QueryTrackingPropertiesEntity getQueryTrackingProperties() throws ArangoDBException {
return executor.execute(getQueryTrackingPropertiesRequest(), QueryTrackingPropertiesEntity.class);
}
/**
* Changes the configuration for the AQL query tracking
*
* @see API
* Documentation
* @param properties
* properties to be set
* @return current set of properties
* @throws ArangoDBException
*/
public QueryTrackingPropertiesEntity setQueryTrackingProperties(final QueryTrackingPropertiesEntity properties)
throws ArangoDBException {
return executor.execute(setQueryTrackingPropertiesRequest(properties), QueryTrackingPropertiesEntity.class);
}
/**
* Returns a list of currently running AQL queries
*
* @see API
* Documentation
* @return a list of currently running AQL queries
* @throws ArangoDBException
*/
public Collection getCurrentlyRunningQueries() throws ArangoDBException {
return executor.execute(getCurrentlyRunningQueriesRequest(), new Type>() {
}.getType());
}
/**
* Returns a list of slow running AQL queries
*
* @see API
* Documentation
* @return a list of slow running AQL queries
* @throws ArangoDBException
*/
public Collection getSlowQueries() throws ArangoDBException {
return executor.execute(getSlowQueriesRequest(), new Type>() {
}.getType());
}
/**
* Clears the list of slow AQL queries
*
* @see API
* Documentation
* @throws ArangoDBException
*/
public void clearSlowQueries() throws ArangoDBException {
executor.execute(clearSlowQueriesRequest(), Void.class);
}
/**
* Kills a running query. The query will be terminated at the next cancelation point.
*
* @see API
* Documentation
* @param id
* The id of the query
* @throws ArangoDBException
*/
public void killQuery(final String id) throws ArangoDBException {
executor.execute(killQueryRequest(id), Void.class);
}
/**
* Create a new AQL user function
*
* @see API
* Documentation
* @param name
* the fully qualified name of the user functions
* @param code
* a string representation of the function body
* @param options
* Additional options, can be null
* @throws ArangoDBException
*/
public void createAqlFunction(final String name, final String code, final AqlFunctionCreateOptions options)
throws ArangoDBException {
executor.execute(createAqlFunctionRequest(name, code, options), Void.class);
}
/**
* Remove an existing AQL user function
*
* @see API
* Documentation
* @param name
* the name of the AQL user function
* @param options
* Additional options, can be null
* @throws ArangoDBException
*/
public void deleteAqlFunction(final String name, final AqlFunctionDeleteOptions options) throws ArangoDBException {
executor.execute(deleteAqlFunctionRequest(name, options), Void.class);
}
/**
* Gets all reqistered AQL user functions
*
* @see API
* Documentation
* @param options
* Additional options, can be null
* @return all reqistered AQL user functions
* @throws ArangoDBException
*/
public Collection getAqlFunctions(final AqlFunctionGetOptions options) throws ArangoDBException {
return executor.execute(getAqlFunctionsRequest(options), new Type>() {
}.getType());
}
/**
* Returns a handler of the graph by the given name
*
* @param name
* Name of the graph
* @return graph handler
*/
public ArangoGraph graph(final String name) {
return new ArangoGraph(this, name);
}
/**
* Create a new graph in the graph module. The creation of a graph requires the name of the graph and a definition
* of its edges.
*
* @see API
* Documentation
* @param name
* Name of the graph
* @param edgeDefinitions
* An array of definitions for the edge
* @return information about the graph
* @throws ArangoDBException
*/
public GraphEntity createGraph(final String name, final Collection edgeDefinitions)
throws ArangoDBException {
return executor.execute(createGraphRequest(name, edgeDefinitions, new GraphCreateOptions()),
createGraphResponseDeserializer());
}
/**
* Create a new graph in the graph module. The creation of a graph requires the name of the graph and a definition
* of its edges.
*
* @see API
* Documentation
* @param name
* Name of the graph
* @param edgeDefinitions
* An array of definitions for the edge
* @param options
* Additional options, can be null
* @return information about the graph
* @throws ArangoDBException
*/
public GraphEntity createGraph(
final String name,
final Collection edgeDefinitions,
final GraphCreateOptions options) throws ArangoDBException {
return executor.execute(createGraphRequest(name, edgeDefinitions, options), createGraphResponseDeserializer());
}
/**
* Lists all graphs known to the graph module
*
* @see API
* Documentation
* @return graphs stored in this database
* @throws ArangoDBException
*/
public Collection getGraphs() throws ArangoDBException {
return executor.execute(getGraphsRequest(), getGraphsResponseDeserializer());
}
/**
* Execute a server-side transaction
*
* @see API
* Documentation
* @param action
* the actual transaction operations to be executed, in the form of stringified JavaScript code
* @param type
* The type of the result (POJO class, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return the result of the transaction if it succeeded
* @throws ArangoDBException
*/
public T transaction(final String action, final Class type, final TransactionOptions options)
throws ArangoDBException {
return executor.execute(transactionRequest(action, options), transactionResponseDeserializer(type));
}
/**
* Retrieves information about the current database
*
* @see API
* Documentation
* @return information about the current database
* @throws ArangoDBException
*/
public DatabaseEntity getInfo() throws ArangoDBException {
return executor.execute(getInfoRequest(), getInfoResponseDeserializer());
}
/**
* Execute a server-side traversal
*
* @see API
* Documentation
* @param vertexClass
* The type of the vertex documents (POJO class, VPackSlice or String for Json)
* @param edgeClass
* The type of the edge documents (POJO class, VPackSlice or String for Json)
* @param options
* Additional options
* @return Result of the executed traversal
* @throws ArangoDBException
*/
public TraversalEntity executeTraversal(
final Class vertexClass,
final Class edgeClass,
final TraversalOptions options) throws ArangoDBException {
final Request request = executeTraversalRequest(options);
return executor.execute(request, executeTraversalResponseDeserializer(vertexClass, edgeClass));
}
/**
* Reads a single document
*
* @see API
* Documentation
* @param id
* The id of the document
* @param type
* The type of the document (POJO class, VPackSlice or String for Json)
* @return the document identified by the id
* @throws ArangoDBException
*/
public T getDocument(final String id, final Class type) throws ArangoDBException {
executor.validateDocumentId(id);
final String[] split = id.split("/");
return collection(split[0]).getDocument(split[1], type);
}
/**
* Reads a single document
*
* @see API
* Documentation
* @param id
* The id of the document
* @param type
* The type of the document (POJO class, VPackSlice or String for Json)
* @param options
* Additional options, can be null
* @return the document identified by the id
* @throws ArangoDBException
*/
public T getDocument(final String id, final Class type, final DocumentReadOptions options)
throws ArangoDBException {
executor.validateDocumentId(id);
final String[] split = id.split("/");
return collection(split[0]).getDocument(split[1], type, options);
}
/**
* Reload the routing table.
*
* @see API
* Documentation
* @throws ArangoDBException
*/
public void reloadRouting() throws ArangoDBException {
executor.execute(reloadRoutingRequest(), Void.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy