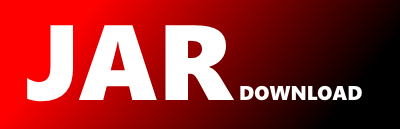
com.arangodb.internal.InternalArangoDatabase Maven / Gradle / Ivy
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb.internal;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import com.arangodb.entity.CollectionEntity;
import com.arangodb.entity.DatabaseEntity;
import com.arangodb.entity.EdgeDefinition;
import com.arangodb.entity.GraphEntity;
import com.arangodb.entity.PathEntity;
import com.arangodb.entity.QueryCachePropertiesEntity;
import com.arangodb.entity.QueryTrackingPropertiesEntity;
import com.arangodb.entity.TraversalEntity;
import com.arangodb.internal.ArangoExecutor.ResponseDeserializer;
import com.arangodb.internal.velocystream.Connection;
import com.arangodb.model.AqlFunctionCreateOptions;
import com.arangodb.model.AqlFunctionDeleteOptions;
import com.arangodb.model.AqlFunctionGetOptions;
import com.arangodb.model.AqlQueryExplainOptions;
import com.arangodb.model.AqlQueryOptions;
import com.arangodb.model.AqlQueryParseOptions;
import com.arangodb.model.CollectionCreateOptions;
import com.arangodb.model.CollectionsReadOptions;
import com.arangodb.model.GraphCreateOptions;
import com.arangodb.model.OptionsBuilder;
import com.arangodb.model.TransactionOptions;
import com.arangodb.model.TraversalOptions;
import com.arangodb.model.UserAccessOptions;
import com.arangodb.velocypack.Type;
import com.arangodb.velocypack.VPackSlice;
import com.arangodb.velocypack.exception.VPackException;
import com.arangodb.velocystream.Request;
import com.arangodb.velocystream.RequestType;
import com.arangodb.velocystream.Response;
/**
* @author Mark - mark at arangodb.com
*
*/
public class InternalArangoDatabase, R, C extends Connection>
extends ArangoExecuteable {
private final String name;
public InternalArangoDatabase(final E executor, final String name) {
super(executor);
this.name = name;
}
public String name() {
return name;
}
protected Request createCollectionRequest(final String name, final CollectionCreateOptions options) {
return new Request(name(), RequestType.POST, ArangoDBConstants.PATH_API_COLLECTION).setBody(
executor.serialize(OptionsBuilder.build(options != null ? options : new CollectionCreateOptions(), name)));
}
protected Request getCollectionsRequest(final CollectionsReadOptions options) {
final Request request;
request = new Request(name(), RequestType.GET, ArangoDBConstants.PATH_API_COLLECTION);
final CollectionsReadOptions params = (options != null ? options : new CollectionsReadOptions());
request.putQueryParam(ArangoDBConstants.EXCLUDE_SYSTEM, params.getExcludeSystem());
return request;
}
protected ResponseDeserializer> getCollectionsResponseDeserializer() {
return new ResponseDeserializer>() {
@Override
public Collection deserialize(final Response response) throws VPackException {
final VPackSlice result = response.getBody().get(ArangoDBConstants.RESULT);
return executor.deserialize(result, new Type>() {
}.getType());
}
};
}
protected Request getIndexRequest(final String id) {
return new Request(name, RequestType.GET, executor.createPath(ArangoDBConstants.PATH_API_INDEX, id));
}
protected Request deleteIndexRequest(final String id) {
return new Request(name, RequestType.DELETE, executor.createPath(ArangoDBConstants.PATH_API_INDEX, id));
}
protected ResponseDeserializer deleteIndexResponseDeserializer() {
return new ResponseDeserializer() {
@Override
public String deserialize(final Response response) throws VPackException {
return response.getBody().get(ArangoDBConstants.ID).getAsString();
}
};
}
protected Request dropRequest() {
return new Request(ArangoDBConstants.SYSTEM, RequestType.DELETE,
executor.createPath(ArangoDBConstants.PATH_API_DATABASE, name));
}
protected ResponseDeserializer createDropResponseDeserializer() {
return new ResponseDeserializer() {
@Override
public Boolean deserialize(final Response response) throws VPackException {
return response.getBody().get(ArangoDBConstants.RESULT).getAsBoolean();
}
};
}
protected Request grantAccessRequest(final String user) {
return new Request(ArangoDBConstants.SYSTEM, RequestType.PUT,
executor.createPath(ArangoDBConstants.PATH_API_USER, user, ArangoDBConstants.DATABASE, name)).setBody(
executor.serialize(OptionsBuilder.build(new UserAccessOptions(), ArangoDBConstants.RW)));
}
protected Request revokeAccessRequest(final String user) {
return new Request(ArangoDBConstants.SYSTEM, RequestType.PUT,
executor.createPath(ArangoDBConstants.PATH_API_USER, user, ArangoDBConstants.DATABASE, name)).setBody(
executor.serialize(OptionsBuilder.build(new UserAccessOptions(), ArangoDBConstants.NONE)));
}
protected Request queryRequest(
final String query,
final Map bindVars,
final AqlQueryOptions options) {
return new Request(name, RequestType.POST, ArangoDBConstants.PATH_API_CURSOR).setBody(executor
.serialize(OptionsBuilder.build(options != null ? options : new AqlQueryOptions(), query, bindVars)));
}
protected Request queryNextRequest(final String id) {
return new Request(name, RequestType.PUT, executor.createPath(ArangoDBConstants.PATH_API_CURSOR, id));
}
protected Request queryCloseRequest(final String id) {
return new Request(name, RequestType.DELETE, executor.createPath(ArangoDBConstants.PATH_API_CURSOR, id));
}
protected Request explainQueryRequest(
final String query,
final Map bindVars,
final AqlQueryExplainOptions options) {
return new Request(name, RequestType.POST, ArangoDBConstants.PATH_API_EXPLAIN).setBody(executor.serialize(
OptionsBuilder.build(options != null ? options : new AqlQueryExplainOptions(), query, bindVars)));
}
protected Request parseQueryRequest(final String query) {
return new Request(name, RequestType.POST, ArangoDBConstants.PATH_API_QUERY)
.setBody(executor.serialize(OptionsBuilder.build(new AqlQueryParseOptions(), query)));
}
protected Request clearQueryCacheRequest() {
return new Request(name, RequestType.DELETE, ArangoDBConstants.PATH_API_QUERY_CACHE);
}
protected Request getQueryCachePropertiesRequest() {
return new Request(name, RequestType.GET, ArangoDBConstants.PATH_API_QUERY_CACHE_PROPERTIES);
}
protected Request setQueryCachePropertiesRequest(final QueryCachePropertiesEntity properties) {
return new Request(name, RequestType.PUT, ArangoDBConstants.PATH_API_QUERY_CACHE_PROPERTIES)
.setBody(executor.serialize(properties));
}
protected Request getQueryTrackingPropertiesRequest() {
return new Request(name, RequestType.GET, ArangoDBConstants.PATH_API_QUERY_PROPERTIES);
}
protected Request setQueryTrackingPropertiesRequest(final QueryTrackingPropertiesEntity properties) {
return new Request(name, RequestType.PUT, ArangoDBConstants.PATH_API_QUERY_PROPERTIES)
.setBody(executor.serialize(properties));
}
protected Request getCurrentlyRunningQueriesRequest() {
return new Request(name, RequestType.GET, ArangoDBConstants.PATH_API_QUERY_CURRENT);
}
protected Request getSlowQueriesRequest() {
return new Request(name, RequestType.GET, ArangoDBConstants.PATH_API_QUERY_SLOW);
}
protected Request clearSlowQueriesRequest() {
return new Request(name, RequestType.DELETE, ArangoDBConstants.PATH_API_QUERY_SLOW);
}
protected Request killQueryRequest(final String id) {
return new Request(name, RequestType.DELETE, executor.createPath(ArangoDBConstants.PATH_API_QUERY, id));
}
protected Request createAqlFunctionRequest(
final String name,
final String code,
final AqlFunctionCreateOptions options) {
return new Request(name(), RequestType.POST, ArangoDBConstants.PATH_API_AQLFUNCTION).setBody(executor.serialize(
OptionsBuilder.build(options != null ? options : new AqlFunctionCreateOptions(), name, code)));
}
protected Request deleteAqlFunctionRequest(final String name, final AqlFunctionDeleteOptions options) {
final Request request = new Request(name(), RequestType.DELETE,
executor.createPath(ArangoDBConstants.PATH_API_AQLFUNCTION, name));
final AqlFunctionDeleteOptions params = options != null ? options : new AqlFunctionDeleteOptions();
request.putQueryParam(ArangoDBConstants.GROUP, params.getGroup());
return request;
}
protected Request getAqlFunctionsRequest(final AqlFunctionGetOptions options) {
final Request request = new Request(name(), RequestType.GET, ArangoDBConstants.PATH_API_AQLFUNCTION);
final AqlFunctionGetOptions params = options != null ? options : new AqlFunctionGetOptions();
request.putQueryParam(ArangoDBConstants.NAMESPACE, params.getNamespace());
return request;
}
protected Request createGraphRequest(
final String name,
final Collection edgeDefinitions,
final GraphCreateOptions options) {
return new Request(name(), RequestType.POST, ArangoDBConstants.PATH_API_GHARIAL).setBody(executor.serialize(
OptionsBuilder.build(options != null ? options : new GraphCreateOptions(), name, edgeDefinitions)));
}
protected ResponseDeserializer createGraphResponseDeserializer() {
return new ResponseDeserializer() {
@Override
public GraphEntity deserialize(final Response response) throws VPackException {
return executor.deserialize(response.getBody().get(ArangoDBConstants.GRAPH), GraphEntity.class);
}
};
}
protected Request getGraphsRequest() {
return new Request(name, RequestType.GET, ArangoDBConstants.PATH_API_GHARIAL);
}
protected ResponseDeserializer> getGraphsResponseDeserializer() {
return new ResponseDeserializer>() {
@Override
public Collection deserialize(final Response response) throws VPackException {
return executor.deserialize(response.getBody().get(ArangoDBConstants.GRAPHS),
new Type>() {
}.getType());
}
};
}
protected Request transactionRequest(final String action, final TransactionOptions options) {
return new Request(name, RequestType.POST, ArangoDBConstants.PATH_API_TRANSACTION).setBody(
executor.serialize(OptionsBuilder.build(options != null ? options : new TransactionOptions(), action)));
}
protected ResponseDeserializer transactionResponseDeserializer(final Class type) {
return new ResponseDeserializer() {
@Override
public T deserialize(final Response response) throws VPackException {
final VPackSlice body = response.getBody();
if (body != null) {
final VPackSlice result = body.get(ArangoDBConstants.RESULT);
if (!result.isNone()) {
return executor.deserialize(result, type);
}
}
return null;
}
};
}
protected Request getInfoRequest() {
return new Request(name, RequestType.GET,
executor.createPath(ArangoDBConstants.PATH_API_DATABASE, ArangoDBConstants.CURRENT));
}
protected ResponseDeserializer getInfoResponseDeserializer() {
return new ResponseDeserializer() {
@Override
public DatabaseEntity deserialize(final Response response) throws VPackException {
return executor.deserialize(response.getBody().get(ArangoDBConstants.RESULT), DatabaseEntity.class);
}
};
}
protected Request executeTraversalRequest(final TraversalOptions options) {
return new Request(name, RequestType.POST, ArangoDBConstants.PATH_API_TRAVERSAL)
.setBody(executor.serialize(options != null ? options : new TransactionOptions()));
}
@SuppressWarnings("hiding")
protected ResponseDeserializer> executeTraversalResponseDeserializer(
final Class vertexClass,
final Class edgeClass) {
return new ResponseDeserializer>() {
@Override
public TraversalEntity deserialize(final Response response) throws VPackException {
final TraversalEntity result = new TraversalEntity();
final VPackSlice visited = response.getBody().get(ArangoDBConstants.RESULT)
.get(ArangoDBConstants.VISITED);
result.setVertices(deserializeVertices(vertexClass, visited));
final Collection> paths = new ArrayList>();
for (final Iterator iterator = visited.get("paths").arrayIterator(); iterator.hasNext();) {
final PathEntity path = new PathEntity();
final VPackSlice next = iterator.next();
path.setEdges(deserializeEdges(edgeClass, next));
path.setVertices(deserializeVertices(vertexClass, next));
paths.add(path);
}
result.setPaths(paths);
return result;
}
};
}
@SuppressWarnings("unchecked")
protected Collection deserializeVertices(final Class vertexClass, final VPackSlice vpack)
throws VPackException {
final Collection vertices = new ArrayList();
for (final Iterator iterator = vpack.get(ArangoDBConstants.VERTICES).arrayIterator(); iterator
.hasNext();) {
vertices.add((V) executor.deserialize(iterator.next(), vertexClass));
}
return vertices;
}
@SuppressWarnings({ "hiding", "unchecked" })
protected Collection deserializeEdges(final Class edgeClass, final VPackSlice next)
throws VPackException {
final Collection edges = new ArrayList();
for (final Iterator iteratorEdge = next.get(ArangoDBConstants.EDGES).arrayIterator(); iteratorEdge
.hasNext();) {
edges.add((E) executor.deserialize(iteratorEdge.next(), edgeClass));
}
return edges;
}
protected Request reloadRoutingRequest() {
return new Request(name, RequestType.POST, ArangoDBConstants.PATH_API_ADMIN_ROUTING_RELOAD);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy