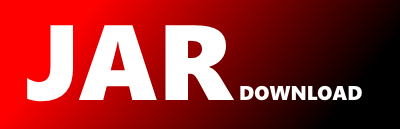
com.arangodb.ArangoGraph Maven / Gradle / Ivy
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb;
import java.util.Collection;
import com.arangodb.entity.EdgeDefinition;
import com.arangodb.entity.GraphEntity;
import com.arangodb.internal.ArangoExecutorSync;
import com.arangodb.internal.InternalArangoGraph;
import com.arangodb.internal.velocystream.internal.ConnectionSync;
import com.arangodb.velocystream.Response;
/**
* @author Mark Vollmary
*
*/
public class ArangoGraph
extends InternalArangoGraph {
protected ArangoGraph(final ArangoDatabase db, final String name) {
super(db, name);
}
/**
* Checks whether the graph exists
*
* @return true if the graph exists, otherwise false
*/
public boolean exists() throws ArangoDBException {
try {
getInfo();
return true;
} catch (final ArangoDBException e) {
return false;
}
}
/**
* Delete an existing graph
*
* @see API Documentation
* @throws ArangoDBException
*/
public void drop() throws ArangoDBException {
executor.execute(dropRequest(), Void.class);
}
/**
* Get a graph from the graph module
*
* @see API Documentation
* @return the definition content of this graph
* @throws ArangoDBException
*/
public GraphEntity getInfo() throws ArangoDBException {
return executor.execute(getInfoRequest(), getInfoResponseDeserializer());
}
/**
* Lists all vertex collections used in this graph
*
* @see API
* Documentation
* @return all vertex collections within this graph
* @throws ArangoDBException
*/
public Collection getVertexCollections() throws ArangoDBException {
return executor.execute(getVertexCollectionsRequest(), getVertexCollectionsResponseDeserializer());
}
/**
* Adds a vertex collection to the set of collections of the graph. If the collection does not exist, it will be
* created.
*
* @see API
* Documentation
* @param name
* The name of the collection
* @return information about the graph
* @throws ArangoDBException
*/
public GraphEntity addVertexCollection(final String name) throws ArangoDBException {
return executor.execute(addVertexCollectionRequest(name), addVertexCollectionResponseDeserializer());
}
/**
* Returns a handler of the vertex collection by the given name
*
* @param name
* Name of the vertex collection
* @return collection handler
*/
public ArangoVertexCollection vertexCollection(final String name) {
return new ArangoVertexCollection(this, name);
}
/**
* Returns a handler of the edge collection by the given name
*
* @param name
* Name of the edge collection
* @return collection handler
*/
public ArangoEdgeCollection edgeCollection(final String name) {
return new ArangoEdgeCollection(this, name);
}
/**
* Lists all edge collections used in this graph
*
* @see API
* Documentation
* @return all edge collections within this graph
* @throws ArangoDBException
*/
public Collection getEdgeDefinitions() throws ArangoDBException {
return executor.execute(getEdgeDefinitionsRequest(), getEdgeDefinitionsDeserializer());
}
/**
* Add a new edge definition to the graph
*
* @see API
* Documentation
* @param definition
* @return information about the graph
* @throws ArangoDBException
*/
public GraphEntity addEdgeDefinition(final EdgeDefinition definition) throws ArangoDBException {
return executor.execute(addEdgeDefinitionRequest(definition), addEdgeDefinitionResponseDeserializer());
}
/**
* Change one specific edge definition. This will modify all occurrences of this definition in all graphs known to
* your database
*
* @see API
* Documentation
* @param definition
* The edge definition
* @return information about the graph
* @throws ArangoDBException
*/
public GraphEntity replaceEdgeDefinition(final EdgeDefinition definition) throws ArangoDBException {
return executor.execute(replaceEdgeDefinitionRequest(definition), replaceEdgeDefinitionResponseDeserializer());
}
/**
* Remove one edge definition from the graph. This will only remove the edge collection, the vertex collections
* remain untouched and can still be used in your queries
*
* @see API
* Documentation
* @param definitionName
* The name of the edge collection used in the definition
* @return information about the graph
* @throws ArangoDBException
*/
public GraphEntity removeEdgeDefinition(final String definitionName) throws ArangoDBException {
return executor.execute(removeEdgeDefinitionRequest(definitionName),
removeEdgeDefinitionResponseDeserializer());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy