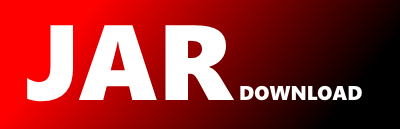
com.arangodb.internal.InternalArangoCollection Maven / Gradle / Ivy
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb.internal;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import com.arangodb.ArangoDBException;
import com.arangodb.entity.DocumentCreateEntity;
import com.arangodb.entity.DocumentDeleteEntity;
import com.arangodb.entity.DocumentField;
import com.arangodb.entity.DocumentUpdateEntity;
import com.arangodb.entity.ErrorEntity;
import com.arangodb.entity.IndexEntity;
import com.arangodb.entity.MultiDocumentEntity;
import com.arangodb.entity.Permissions;
import com.arangodb.internal.ArangoExecutor.ResponseDeserializer;
import com.arangodb.internal.velocystream.internal.VstConnection;
import com.arangodb.model.CollectionPropertiesOptions;
import com.arangodb.model.CollectionRenameOptions;
import com.arangodb.model.DocumentCreateOptions;
import com.arangodb.model.DocumentDeleteOptions;
import com.arangodb.model.DocumentExistsOptions;
import com.arangodb.model.DocumentImportOptions;
import com.arangodb.model.DocumentReadOptions;
import com.arangodb.model.DocumentReplaceOptions;
import com.arangodb.model.DocumentUpdateOptions;
import com.arangodb.model.FulltextIndexOptions;
import com.arangodb.model.GeoIndexOptions;
import com.arangodb.model.HashIndexOptions;
import com.arangodb.model.ImportType;
import com.arangodb.model.OptionsBuilder;
import com.arangodb.model.PersistentIndexOptions;
import com.arangodb.model.SkiplistIndexOptions;
import com.arangodb.model.UserAccessOptions;
import com.arangodb.util.ArangoSerializer;
import com.arangodb.velocypack.Type;
import com.arangodb.velocypack.VPackSlice;
import com.arangodb.velocypack.exception.VPackException;
import com.arangodb.velocystream.Request;
import com.arangodb.velocystream.RequestType;
import com.arangodb.velocystream.Response;
/**
* @author Mark Vollmary
*
*/
public class InternalArangoCollection, D extends InternalArangoDatabase, E extends ArangoExecutor, R, C extends VstConnection>
extends ArangoExecuteable {
private final D db;
private final String name;
public InternalArangoCollection(final D db, final String name) {
super(db.executor(), db.util());
this.db = db;
this.name = name;
}
public D db() {
return db;
}
public String name() {
return name;
}
protected Request insertDocumentRequest(final T value, final DocumentCreateOptions options) {
final Request request = new Request(db.name(), RequestType.POST,
executor.createPath(ArangoDBConstants.PATH_API_DOCUMENT, name));
final DocumentCreateOptions params = (options != null ? options : new DocumentCreateOptions());
request.putQueryParam(ArangoDBConstants.WAIT_FOR_SYNC, params.getWaitForSync());
request.putQueryParam(ArangoDBConstants.RETURN_NEW, params.getReturnNew());
request.setBody(util().serialize(value));
return request;
}
protected ResponseDeserializer> insertDocumentResponseDeserializer(final T value) {
return new ResponseDeserializer>() {
@SuppressWarnings("unchecked")
@Override
public DocumentCreateEntity deserialize(final Response response) throws VPackException {
final VPackSlice body = response.getBody();
final DocumentCreateEntity doc = util().deserialize(body, DocumentCreateEntity.class);
final VPackSlice newDoc = body.get(ArangoDBConstants.NEW);
if (newDoc.isObject()) {
doc.setNew((T) util().deserialize(newDoc, value.getClass()));
}
final Map values = new HashMap();
values.put(DocumentField.Type.ID, doc.getId());
values.put(DocumentField.Type.KEY, doc.getKey());
values.put(DocumentField.Type.REV, doc.getRev());
executor.documentCache().setValues(value, values);
return doc;
}
};
}
protected Request insertDocumentsRequest(final Collection values, final DocumentCreateOptions params) {
final Request request = new Request(db.name(), RequestType.POST,
executor.createPath(ArangoDBConstants.PATH_API_DOCUMENT, name));
request.putQueryParam(ArangoDBConstants.WAIT_FOR_SYNC, params.getWaitForSync());
request.putQueryParam(ArangoDBConstants.RETURN_NEW, params.getReturnNew());
request.setBody(
util().serialize(values, new ArangoSerializer.Options().serializeNullValues(false).stringAsJson(true)));
return request;
}
@SuppressWarnings("unchecked")
protected ResponseDeserializer>> insertDocumentsResponseDeserializer(
final Collection values,
final DocumentCreateOptions params) {
return new ResponseDeserializer>>() {
@Override
public MultiDocumentEntity> deserialize(final Response response)
throws VPackException {
Class type = null;
if (params.getReturnNew() != null && params.getReturnNew()) {
if (!values.isEmpty()) {
type = (Class) values.iterator().next().getClass();
}
}
final MultiDocumentEntity> multiDocument = new MultiDocumentEntity>();
final Collection> docs = new ArrayList>();
final Collection errors = new ArrayList();
final Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy