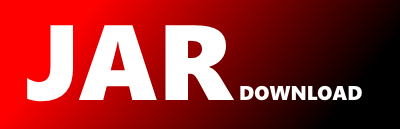
com.arangodb.Request Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core module for ArangoDB Java Driver
package com.arangodb;
import java.util.HashMap;
import java.util.Map;
public final class Request {
private final String db;
private final Method method;
private final String path;
private final Map queryParams;
private final Map headers;
private final T body;
public enum Method {
DELETE,
GET,
POST,
PUT,
HEAD,
PATCH,
OPTIONS
}
public static Builder builder() {
return new Builder<>();
}
private Request(String db, Method method, String path, Map queryParams, Map headers, T body) {
this.db = db;
this.method = method;
this.path = path;
this.queryParams = queryParams;
this.headers = headers;
this.body = body;
}
public String getDb() {
return db;
}
public Method getMethod() {
return method;
}
public String getPath() {
return path;
}
public Map getQueryParams() {
return queryParams;
}
public Map getHeaders() {
return headers;
}
public T getBody() {
return body;
}
public static final class Builder {
private String db;
private Request.Method method;
private String path;
private final Map queryParams;
private final Map headers;
private T body;
public Builder() {
queryParams = new HashMap<>();
headers = new HashMap<>();
}
public Builder db(String db) {
this.db = db;
return this;
}
public Builder method(Request.Method method) {
this.method = method;
return this;
}
public Builder path(String path) {
this.path = path;
return this;
}
public Builder queryParam(final String key, final String value) {
if (value != null) {
queryParams.put(key, value);
}
return this;
}
public Builder queryParams(Map queryParams) {
if (queryParams != null) {
for (Map.Entry it : queryParams.entrySet()) {
queryParam(it.getKey(), it.getValue());
}
}
return this;
}
public Builder header(final String key, final String value) {
if (value != null) {
headers.put(key, value);
}
return this;
}
public Builder headers(Map headers) {
if (headers != null) {
for (Map.Entry it : headers.entrySet()) {
header(it.getKey(), it.getValue());
}
}
return this;
}
public Builder body(T body) {
this.body = body;
return this;
}
public Request build() {
return new Request<>(db, method, path, queryParams, headers, body);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy