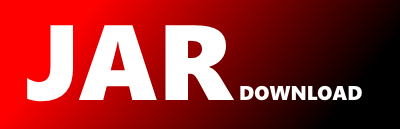
com.arangodb.internal.net.HostSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core module for ArangoDB Java Driver
package com.arangodb.internal.net;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
public class HostSet {
private static final Logger LOGGER = LoggerFactory.getLogger(HostSet.class);
private final ArrayList hosts = new ArrayList<>();
private volatile String jwt = null;
public HostSet() {
super();
}
public HostSet(List hosts) {
super();
for (Host host : hosts) {
addHost(host);
}
}
public List getHostsList() {
return Collections.unmodifiableList(hosts);
}
public void addHost(Host newHost) {
if (hosts.contains(newHost)) {
LOGGER.debug("Host {} already in Set", newHost);
for (Host host : hosts) {
if (host.equals(newHost)) {
host.setMarkforDeletion(false);
}
}
} else {
newHost.setJwt(jwt);
hosts.add(newHost);
LOGGER.debug("Added Host {} - now {} Hosts in List", newHost, hosts.size());
}
}
public void close() {
LOGGER.debug("Close all Hosts in Set");
for (Host host : hosts) {
try {
LOGGER.debug("Try to close Host {}", host);
host.close();
} catch (IOException e) {
LOGGER.warn("Error during closing the Host " + host, e);
}
}
}
public void markAllForDeletion() {
for (Host host : hosts) {
host.setMarkforDeletion(true);
}
}
public void clearAllMarkedForDeletion() {
LOGGER.debug("Clear all Hosts in Set with markForDeletion");
Iterator iterable = hosts.iterator();
while (iterable.hasNext()) {
Host host = iterable.next();
if (host.isMarkforDeletion()) {
try {
LOGGER.debug("Try to close Host {}", host);
host.close();
} catch (IOException e) {
LOGGER.warn("Error during closing the Host " + host, e);
} finally {
iterable.remove();
}
}
}
}
public void clear() {
LOGGER.debug("Clear all Hosts in Set");
close();
hosts.clear();
}
public void setJwt(String jwt) {
this.jwt = jwt;
for (Host h : hosts) {
h.setJwt(jwt);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy