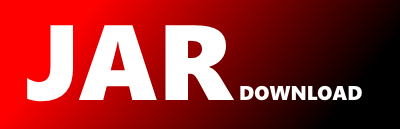
com.arangodb.ArangoCollectionAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core module for ArangoDB Java Driver
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb;
import com.arangodb.entity.*;
import com.arangodb.model.*;
import com.arangodb.util.RawData;
import javax.annotation.concurrent.ThreadSafe;
import java.util.Collection;
import java.util.concurrent.CompletableFuture;
/**
* Asynchronous version of {@link ArangoCollection}
*/
@ThreadSafe
public interface ArangoCollectionAsync extends ArangoSerdeAccessor {
/**
* @return database async API
*/
ArangoDatabaseAsync db();
/**
* @return collection name
*/
String name();
/**
* Asynchronous version of {@link ArangoCollection#insertDocument(Object)}
*/
CompletableFuture> insertDocument(Object value);
/**
* Asynchronous version of {@link ArangoCollection#insertDocument(Object, DocumentCreateOptions)}
*/
CompletableFuture> insertDocument(T value, DocumentCreateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#insertDocument(Object, DocumentCreateOptions, Class)}
*/
CompletableFuture> insertDocument(T value, DocumentCreateOptions options, Class type);
/**
* Asynchronous version of {@link ArangoCollection#insertDocuments(RawData)}
*/
CompletableFuture>> insertDocuments(RawData values);
/**
* Asynchronous version of {@link ArangoCollection#insertDocuments(RawData, DocumentCreateOptions)}
*/
CompletableFuture>> insertDocuments(
RawData values, DocumentCreateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#insertDocuments(Iterable)}
*/
CompletableFuture>> insertDocuments(Iterable> values);
/**
* Asynchronous version of {@link ArangoCollection#insertDocuments(Iterable, DocumentCreateOptions)}
*/
CompletableFuture>> insertDocuments(
Iterable> values, DocumentCreateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#insertDocuments(Iterable, DocumentCreateOptions, Class)}
*/
CompletableFuture>> insertDocuments(
Iterable extends T> values, DocumentCreateOptions options, Class type);
/**
* Asynchronous version of {@link ArangoCollection#importDocuments(Iterable)}
*/
CompletableFuture importDocuments(Iterable> values);
/**
* Asynchronous version of {@link ArangoCollection#importDocuments(Iterable, DocumentImportOptions)}
*/
CompletableFuture importDocuments(Iterable> values, DocumentImportOptions options);
/**
* Asynchronous version of {@link ArangoCollection#importDocuments(RawData)}
*/
CompletableFuture importDocuments(RawData values);
/**
* Asynchronous version of {@link ArangoCollection#importDocuments(RawData, DocumentImportOptions)}
*/
CompletableFuture importDocuments(RawData values, DocumentImportOptions options);
/**
* Asynchronous version of {@link ArangoCollection#getDocument(String, Class)}
*/
CompletableFuture getDocument(String key, Class type);
/**
* Asynchronous version of {@link ArangoCollection#getDocument(String, Class, DocumentReadOptions)}
*/
CompletableFuture getDocument(String key, Class type, DocumentReadOptions options);
/**
* Asynchronous version of {@link ArangoCollection#getDocuments(Iterable, Class)}
*/
CompletableFuture> getDocuments(Iterable keys, Class type);
/**
* Asynchronous version of {@link ArangoCollection#getDocuments(Iterable, Class, DocumentReadOptions)}
*/
CompletableFuture> getDocuments(Iterable keys, Class type, DocumentReadOptions options);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocument(String, Object)}
*/
CompletableFuture> replaceDocument(String key, Object value);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocument(String, Object, DocumentReplaceOptions)}
*/
CompletableFuture> replaceDocument(String key, T value, DocumentReplaceOptions options);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocument(String, Object, DocumentReplaceOptions, Class)}
*/
CompletableFuture> replaceDocument(String key, T value, DocumentReplaceOptions options, Class type);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocuments(RawData)}
*/
CompletableFuture>> replaceDocuments(RawData values);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocuments(RawData, DocumentReplaceOptions)}
*/
CompletableFuture>> replaceDocuments(
RawData values, DocumentReplaceOptions options);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocuments(Iterable)} )}
*/
CompletableFuture>> replaceDocuments(Iterable> values);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocuments(Iterable, DocumentReplaceOptions)}
*/
CompletableFuture>> replaceDocuments(
Iterable> values, DocumentReplaceOptions options);
/**
* Asynchronous version of {@link ArangoCollection#replaceDocuments(Iterable, DocumentReplaceOptions, Class)}
*/
CompletableFuture>> replaceDocuments(
Iterable extends T> values, DocumentReplaceOptions options, Class type);
/**
* Asynchronous version of {@link ArangoCollection#updateDocument(String, Object)}
*/
CompletableFuture> updateDocument(String key, Object value);
/**
* Asynchronous version of {@link ArangoCollection#updateDocument(String, Object, DocumentUpdateOptions)}
*/
CompletableFuture> updateDocument(String key, T value, DocumentUpdateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#updateDocument(String, Object, DocumentUpdateOptions, Class)}
*/
CompletableFuture> updateDocument(String key, Object value, DocumentUpdateOptions options,
Class returnType);
/**
* Asynchronous version of {@link ArangoCollection#updateDocuments(RawData)}
*/
CompletableFuture>> updateDocuments(RawData values);
/**
* Asynchronous version of {@link ArangoCollection#updateDocuments(RawData, DocumentUpdateOptions)}
*/
CompletableFuture>> updateDocuments(
RawData values, DocumentUpdateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#updateDocuments(Iterable)}
*/
CompletableFuture>> updateDocuments(Iterable> values);
/**
* Asynchronous version of {@link ArangoCollection#updateDocuments(Iterable, DocumentUpdateOptions)}
*/
CompletableFuture>> updateDocuments(
Iterable> values, DocumentUpdateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#updateDocuments(Iterable, DocumentUpdateOptions, Class)}
*/
CompletableFuture>> updateDocuments(
Iterable> values, DocumentUpdateOptions options, Class returnType);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocument(String)}
*/
CompletableFuture> deleteDocument(String key);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocument(String, DocumentDeleteOptions)}
*/
CompletableFuture> deleteDocument(String key, DocumentDeleteOptions options);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocument(String, DocumentDeleteOptions, Class)}
*/
CompletableFuture> deleteDocument(String key, DocumentDeleteOptions options, Class type);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocuments(RawData)}
*/
CompletableFuture>> deleteDocuments(RawData values);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocuments(RawData, DocumentDeleteOptions)}
*/
CompletableFuture>> deleteDocuments(
RawData values, DocumentDeleteOptions options);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocuments(Iterable)}
*/
CompletableFuture>> deleteDocuments(Iterable> values);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocuments(Iterable, DocumentDeleteOptions)}
*/
CompletableFuture>> deleteDocuments(
Iterable> values, DocumentDeleteOptions options);
/**
* Asynchronous version of {@link ArangoCollection#deleteDocuments(Iterable, DocumentDeleteOptions, Class)}
*/
CompletableFuture>> deleteDocuments(
Iterable> values, DocumentDeleteOptions options, Class type);
/**
* Asynchronous version of {@link ArangoCollection#documentExists(String)}
*/
CompletableFuture documentExists(String key);
/**
* Asynchronous version of {@link ArangoCollection#documentExists(String, DocumentExistsOptions)}
*/
CompletableFuture documentExists(String key, DocumentExistsOptions options);
/**
* Asynchronous version of {@link ArangoCollection#getIndex(String)}
*/
CompletableFuture getIndex(String id);
/**
* Asynchronous version of {@link ArangoCollection#getInvertedIndex(String)}
*/
CompletableFuture getInvertedIndex(String id);
/**
* Asynchronous version of {@link ArangoCollection#deleteIndex(String)}
*/
CompletableFuture deleteIndex(String id);
/**
* Asynchronous version of {@link ArangoCollection#ensurePersistentIndex(Iterable, PersistentIndexOptions)}
*/
CompletableFuture ensurePersistentIndex(Iterable fields, PersistentIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#ensureGeoIndex(Iterable, GeoIndexOptions)}
*/
CompletableFuture ensureGeoIndex(Iterable fields, GeoIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#ensureFulltextIndex(Iterable, FulltextIndexOptions)}
*/
@Deprecated
CompletableFuture ensureFulltextIndex(Iterable fields, FulltextIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#ensureTtlIndex(Iterable, TtlIndexOptions)}
*/
CompletableFuture ensureTtlIndex(Iterable fields, TtlIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#ensureZKDIndex(Iterable, ZKDIndexOptions)}
*
* @deprecated since ArangoDB 3.12, use {@link #ensureMDIndex(Iterable, MDIndexOptions)} or
* {@link #ensureMDPrefixedIndex(Iterable, MDPrefixedIndexOptions)} instead.
*/
@Deprecated
CompletableFuture ensureZKDIndex(Iterable fields, ZKDIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#ensureMDIndex(Iterable, MDIndexOptions)}
*/
CompletableFuture ensureMDIndex(Iterable fields, MDIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#ensureMDPrefixedIndex(Iterable, MDPrefixedIndexOptions)}
*/
CompletableFuture ensureMDPrefixedIndex(Iterable fields, MDPrefixedIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#ensureInvertedIndex(InvertedIndexOptions)}
*/
CompletableFuture ensureInvertedIndex(InvertedIndexOptions options);
/**
* Asynchronous version of {@link ArangoCollection#getIndexes()}
*/
CompletableFuture> getIndexes();
/**
* Asynchronous version of {@link ArangoCollection#getInvertedIndexes()}
*/
CompletableFuture> getInvertedIndexes();
/**
* Asynchronous version of {@link ArangoCollection#exists()}
*/
CompletableFuture exists();
/**
* Asynchronous version of {@link ArangoCollection#truncate()}
*/
CompletableFuture truncate();
/**
* Asynchronous version of {@link ArangoCollection#truncate(CollectionTruncateOptions)}
*/
CompletableFuture truncate(CollectionTruncateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#count()}
*/
CompletableFuture count();
/**
* Asynchronous version of {@link ArangoCollection#count(CollectionCountOptions)}
*/
CompletableFuture count(CollectionCountOptions options);
/**
* Asynchronous version of {@link ArangoCollection#create()}
*/
CompletableFuture create();
/**
* Asynchronous version of {@link ArangoCollection#create(CollectionCreateOptions)}
*/
CompletableFuture create(CollectionCreateOptions options);
/**
* Asynchronous version of {@link ArangoCollection#drop()}
*/
CompletableFuture drop();
/**
* Asynchronous version of {@link ArangoCollection#drop(boolean)}
*/
CompletableFuture drop(boolean isSystem);
/**
* Asynchronous version of {@link ArangoCollection#getInfo()}
*/
CompletableFuture getInfo();
/**
* Asynchronous version of {@link ArangoCollection#getProperties()}
*/
CompletableFuture getProperties();
/**
* Asynchronous version of {@link ArangoCollection#changeProperties(CollectionPropertiesOptions)}
*/
CompletableFuture changeProperties(CollectionPropertiesOptions options);
/**
* Asynchronous version of {@link ArangoCollection#rename(String)}
*/
CompletableFuture rename(String newName);
/**
* Asynchronous version of {@link ArangoCollection#getResponsibleShard(Object)}
*/
CompletableFuture getResponsibleShard(final Object value);
/**
* Asynchronous version of {@link ArangoCollection#getRevision()}
*/
CompletableFuture getRevision();
/**
* Asynchronous version of {@link ArangoCollection#grantAccess(String, Permissions)}
*/
CompletableFuture grantAccess(String user, Permissions permissions);
/**
* Asynchronous version of {@link ArangoCollection#revokeAccess(String)}
*/
CompletableFuture revokeAccess(String user);
/**
* Asynchronous version of {@link ArangoCollection#resetAccess(String)}
*/
CompletableFuture resetAccess(String user);
/**
* Asynchronous version of {@link ArangoCollection#getPermissions(String)}
*/
CompletableFuture getPermissions(String user);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy