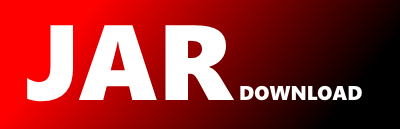
com.arangodb.ArangoDatabase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core module for ArangoDB Java Driver
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb;
import com.arangodb.entity.*;
import com.arangodb.entity.arangosearch.analyzer.SearchAnalyzer;
import com.arangodb.model.*;
import com.arangodb.model.arangosearch.AnalyzerDeleteOptions;
import com.arangodb.model.arangosearch.ArangoSearchCreateOptions;
import com.arangodb.model.arangosearch.SearchAliasCreateOptions;
import javax.annotation.concurrent.ThreadSafe;
import java.util.Collection;
import java.util.Map;
/**
* Interface for operations on ArangoDB database level.
*
* @author Mark Vollmary
* @author Michele Rastelli
* @see Databases API Documentation
* @see Query API Documentation
*/
@ThreadSafe
public interface ArangoDatabase extends ArangoSerdeAccessor {
/**
* Return the main entry point for the ArangoDB driver
*
* @return main entry point
*/
ArangoDB arango();
/**
* Returns the name of the database
*
* @return database name
*/
String name();
/**
* Returns the server name and version number.
*
* @return the server version, number
* @see API
* Documentation
*/
ArangoDBVersion getVersion();
/**
* Returns the name of the used storage engine.
*
* @return the storage engine name
* @see API
* Documentation
*/
ArangoDBEngine getEngine();
/**
* Checks whether the database exists
*
* @return true if the database exists, otherwise false
* @see API
* Documentation
*/
boolean exists();
/**
* Retrieves a list of all databases the current user can access
*
* @return a list of all databases the current user can access
* @see API
* Documentation
*/
Collection getAccessibleDatabases();
/**
* Returns a {@code ArangoCollection} instance for the given collection name.
*
* @param name Name of the collection
* @return collection handler
*/
ArangoCollection collection(String name);
/**
* Creates a collection for the given collection's name, then returns collection information from the server.
*
* @param name The name of the collection
* @return information about the collection
* @see API
* Documentation
*/
CollectionEntity createCollection(String name);
/**
* Creates a collection with the given {@code options} for this collection's name, then returns collection
* information from the server.
*
* @param name The name of the collection
* @param options Additional options, can be null
* @return information about the collection
* @see API
* Documentation
*/
CollectionEntity createCollection(String name, CollectionCreateOptions options);
/**
* Fetches all collections from the database and returns an list of collection descriptions.
*
* @return list of information about all collections
* @see API
* Documentation
*/
Collection getCollections();
/**
* Fetches all collections from the database and returns an list of collection descriptions.
*
* @param options Additional options, can be null
* @return list of information about all collections
* @see API
* Documentation
*/
Collection getCollections(CollectionsReadOptions options);
/**
* Returns an index
*
* @param id The index-handle
* @return information about the index
* @see API Documentation
*/
IndexEntity getIndex(String id);
/**
* Deletes an index
*
* @param id The index-handle
* @return the id of the index
* @see API Documentation
*/
String deleteIndex(String id);
/**
* Creates the database
*
* @return true if the database was created successfully.
* @see API
* Documentation
*/
Boolean create();
/**
* Deletes the database from the server.
*
* @return true if the database was dropped successfully
* @see API
* Documentation
*/
Boolean drop();
/**
* Grants or revoke access to the database for user {@code user}. You need permission to the _system database in
* order to execute this call.
*
* @param user The name of the user
* @param permissions The permissions the user grant
* @see
* API Documentation
*/
void grantAccess(String user, Permissions permissions);
/**
* Grants access to the database for user {@code user}. You need permission to the _system database in order to
* execute this call.
*
* @param user The name of the user
* @see
* API Documentation
*/
void grantAccess(String user);
/**
* Revokes access to the database dbname for user {@code user}. You need permission to the _system database in order
* to execute this call.
*
* @param user The name of the user
* @see
* API Documentation
*/
void revokeAccess(String user);
/**
* Clear the database access level, revert back to the default access level.
*
* @param user The name of the user
* @see
* API Documentation
* @since ArangoDB 3.2.0
*/
void resetAccess(String user);
/**
* Sets the default access level for collections within this database for the user {@code user}. You need permission
* to the _system database in order to execute this call.
*
* @param user The name of the user
* @param permissions The permissions the user grant
* @see
* API Documentation
* @since ArangoDB 3.2.0
*/
void grantDefaultCollectionAccess(String user, Permissions permissions);
/**
* Get specific database access level
*
* @param user The name of the user
* @return permissions of the user
* @see API
* Documentation
* @since ArangoDB 3.2.0
*/
Permissions getPermissions(String user);
/**
* Performs a database query using the given {@code query} and {@code bindVars}, then returns a new
* {@code ArangoCursor} instance for the result list.
*
* @param query An AQL query string
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @param bindVars key/value pairs defining the variables to bind the query to
* @param options Additional options that will be passed to the query API, can be null
* @return cursor of the results
* @see API
* Documentation
*/
ArangoCursor query(String query, Class type, Map bindVars, AqlQueryOptions options);
/**
* Performs a database query using the given {@code query}, then returns a new {@code ArangoCursor} instance for the
* result list.
*
* @param query An AQL query string
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options that will be passed to the query API, can be null
* @return cursor of the results
* @see API
* Documentation
*/
ArangoCursor query(String query, Class type, AqlQueryOptions options);
/**
* Performs a database query using the given {@code query} and {@code bindVars}, then returns a new
* {@code ArangoCursor} instance for the result list.
*
* @param query An AQL query string
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @param bindVars key/value pairs defining the variables to bind the query to
* @return cursor of the results
* @see API
* Documentation
*/
ArangoCursor query(String query, Class type, Map bindVars);
/**
* Performs a database query using the given {@code query}, then returns a new {@code ArangoCursor} instance for the
* result list.
*
* @param query An AQL query string
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @return cursor of the results
* @see API
* Documentation
*/
ArangoCursor query(String query, Class type);
/**
* Return an cursor from the given cursor-ID if still existing
*
* @param cursorId The ID of the cursor
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @return cursor of the results
* @see API
* Documentation
*/
ArangoCursor cursor(String cursorId, Class type);
/**
* Return an cursor from the given cursor-ID if still existing
*
* @param cursorId The ID of the cursor
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @param options options
* @return cursor of the results
* @see API
* Documentation
*/
ArangoCursor cursor(String cursorId, Class type, AqlQueryOptions options);
/**
* Return an cursor from the given cursor-ID if still existing
*
* @param cursorId The ID of the cursor
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @param nextBatchId The ID of the next cursor batch (set only if cursor allows retries, see
* {@link AqlQueryOptions#allowRetry(Boolean)}
* @return cursor of the results
* @see API Documentation
* @since ArangoDB 3.11
*/
ArangoCursor cursor(String cursorId, Class type, String nextBatchId);
/**
* Return an cursor from the given cursor-ID if still existing
*
* @param cursorId The ID of the cursor
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @param nextBatchId The ID of the next cursor batch (set only if cursor allows retries, see
* {@link AqlQueryOptions#allowRetry(Boolean)}
* @param options options
* @return cursor of the results
* @see API Documentation
* @since ArangoDB 3.11
*/
ArangoCursor cursor(String cursorId, Class type, String nextBatchId, AqlQueryOptions options);
/**
* Explain an AQL query and return information about it
*
* @param query the query which you want explained
* @param bindVars key/value pairs representing the bind parameters
* @param options Additional options, can be null
* @return information about the query
* @see API
* Documentation
*
* @deprecated for removal, use {@link ArangoDatabase#explainAqlQuery(String, Map, AqlQueryExplainOptions)} instead
*/
@Deprecated
AqlExecutionExplainEntity explainQuery(String query, Map bindVars, AqlQueryExplainOptions options);
/**
* Explain an AQL query and return information about it
*
* @param query the query which you want explained
* @param bindVars key/value pairs representing the bind parameters
* @param options Additional options, can be null
* @return information about the query
* @see API
* Documentation
*
* @deprecated for removal, use {@link ArangoDatabase#explainAqlQuery(String, Map, ExplainAqlQueryOptions)} instead
*/
@Deprecated
AqlQueryExplainEntity explainAqlQuery(String query, Map bindVars, AqlQueryExplainOptions options);
/**
* Explain an AQL query and return information about it
*
* @param query the query which you want explained
* @param bindVars key/value pairs representing the bind parameters
* @param options Additional options, can be null
* @return information about the query
* @see API
* Documentation
*/
AqlQueryExplainEntity explainAqlQuery(String query, Map bindVars, ExplainAqlQueryOptions options);
/**
* Parse an AQL query and return information about it This method is for query validation only. To actually query
* the database, see {@link ArangoDatabase#query(String, Class, Map, AqlQueryOptions)}
*
* @param query the query which you want parse
* @return imformation about the query
* @see API
* Documentation
*/
AqlParseEntity parseQuery(String query);
/**
* Clears the AQL query cache
*
* @see API
* Documentation
*/
void clearQueryCache();
/**
* Returns the global configuration for the AQL query cache
*
* @return configuration for the AQL query cache
* @see API
* Documentation
*/
QueryCachePropertiesEntity getQueryCacheProperties();
/**
* Changes the configuration for the AQL query cache. Note: changing the properties may invalidate all results in
* the cache.
*
* @param properties properties to be set
* @return current set of properties
* @see API
* Documentation
*/
QueryCachePropertiesEntity setQueryCacheProperties(QueryCachePropertiesEntity properties);
/**
* Returns the configuration for the AQL query tracking
*
* @return configuration for the AQL query tracking
* @see API
* Documentation
*/
QueryTrackingPropertiesEntity getQueryTrackingProperties();
/**
* Changes the configuration for the AQL query tracking
*
* @param properties properties to be set
* @return current set of properties
* @see API
* Documentation
*/
QueryTrackingPropertiesEntity setQueryTrackingProperties(QueryTrackingPropertiesEntity properties);
/**
* Returns a list of currently running AQL queries
*
* @return a list of currently running AQL queries
* @see API
* Documentation
*/
Collection getCurrentlyRunningQueries();
/**
* Returns a list of slow running AQL queries
*
* @return a list of slow running AQL queries
* @see API
* Documentation
*/
Collection getSlowQueries();
/**
* Clears the list of slow AQL queries
*
* @see API
* Documentation
*/
void clearSlowQueries();
/**
* Kills a running query. The query will be terminated at the next cancelation point.
*
* @param id The id of the query
* @see API
* Documentation
*/
void killQuery(String id);
/**
* Create a new AQL user function
*
* @param name A valid AQL function name, e.g.: `"myfuncs::accounting::calculate_vat"`
* @param code A String evaluating to a JavaScript function
* @param options Additional options, can be null
* @see API
* Documentation
*/
void createAqlFunction(String name, String code, AqlFunctionCreateOptions options);
/**
* Deletes the AQL user function with the given name from the database.
*
* @param name The name of the user function to delete
* @param options Additional options, can be null
* @return number of deleted functions (since ArangoDB 3.4.0)
* @see API
* Documentation
*/
Integer deleteAqlFunction(String name, AqlFunctionDeleteOptions options);
/**
* Gets all reqistered AQL user functions
*
* @param options Additional options, can be null
* @return all reqistered AQL user functions
* @see API
* Documentation
*/
Collection getAqlFunctions(AqlFunctionGetOptions options);
/**
* Returns a {@code ArangoGraph} instance for the given graph name.
*
* @param name Name of the graph
* @return graph handler
*/
ArangoGraph graph(String name);
/**
* Create a new graph in the graph module. The creation of a graph requires the name of the graph and a definition
* of its edges.
*
* @param name Name of the graph
* @param edgeDefinitions An array of definitions for the edge
* @return information about the graph
* @see API
* Documentation
*/
GraphEntity createGraph(String name, Iterable edgeDefinitions);
/**
* Create a new graph in the graph module. The creation of a graph requires the name of the graph and a definition
* of its edges.
*
* @param name Name of the graph
* @param edgeDefinitions An array of definitions for the edge
* @param options Additional options, can be null
* @return information about the graph
* @see API
* Documentation
*/
GraphEntity createGraph(String name, Iterable edgeDefinitions, GraphCreateOptions options);
/**
* Lists all graphs known to the graph module
*
* @return graphs stored in this database
* @see API
* Documentation
*/
Collection getGraphs();
/**
* Performs a server-side transaction and returns its return value.
*
* @param action A String evaluating to a JavaScript function to be executed on the server.
* @param type The type of the result (POJO or {@link com.arangodb.util.RawData})
* @param options Additional options, can be null
* @return the result of the transaction if it succeeded
* @see API
* Documentation
*/
T transaction(String action, Class type, TransactionOptions options);
/**
* Begins a Stream Transaction.
*
* @param options Additional options, can be null
* @return information about the transaction
* @see API
* Documentation
* @since ArangoDB 3.5.0
*/
StreamTransactionEntity beginStreamTransaction(StreamTransactionOptions options);
/**
* Aborts a Stream Transaction.
*
* @return information about the transaction
* @see API
* Documentation
*/
StreamTransactionEntity abortStreamTransaction(String id);
/**
* Gets information about a Stream Transaction.
*
* @return information about the transaction
* @see
* API Documentation
* @since ArangoDB 3.5.0
*/
StreamTransactionEntity getStreamTransaction(String id);
/**
* Gets all the currently running Stream Transactions.
*
* @return all the currently running Stream Transactions
* @see
* API Documentation
* @since ArangoDB 3.5.0
*/
Collection getStreamTransactions();
/**
* Commits a Stream Transaction.
*
* @return information about the transaction
* @see
* API Documentation
* @since ArangoDB 3.5.0
*/
StreamTransactionEntity commitStreamTransaction(String id);
/**
* Retrieves information about the current database
*
* @return information about the current database
* @see API
* Documentation
*/
DatabaseEntity getInfo();
/**
* Reload the routing table.
*
* @see API
* Documentation
*/
void reloadRouting();
/**
* Fetches all views from the database and returns a list of view descriptions.
*
* @return list of information about all views
* @see API Documentation
* @since ArangoDB 3.4.0
*/
Collection getViews();
/**
* Returns a {@code ArangoView} instance for the given view name.
*
* @param name Name of the view
* @return view handler
* @since ArangoDB 3.4.0
*/
ArangoView view(String name);
/**
* Returns a {@link ArangoSearch} instance for the given view name.
*
* @param name Name of the view
* @return ArangoSearch view handler
* @since ArangoDB 3.4.0
*/
ArangoSearch arangoSearch(String name);
/**
* Returns a {@link SearchAlias} instance for the given view name.
*
* @param name Name of the view
* @return SearchAlias view handler
* @since ArangoDB 3.10
*/
SearchAlias searchAlias(String name);
/**
* Creates a view of the given {@code type}, then returns view information from the server.
*
* @param name The name of the view
* @param type The type of the view
* @return information about the view
* @since ArangoDB 3.4.0
*/
ViewEntity createView(String name, ViewType type);
/**
* Creates a ArangoSearch view with the given {@code options}, then returns view information from the server.
*
* @param name The name of the view
* @param options Additional options, can be null
* @return information about the view
* @see API
* Documentation
* @since ArangoDB 3.4.0
*/
ViewEntity createArangoSearch(String name, ArangoSearchCreateOptions options);
/**
* Creates a SearchAlias view with the given {@code options}, then returns view information from the server.
*
* @param name The name of the view
* @param options Additional options, can be null
* @return information about the view
* @see API
* Documentation
* @since ArangoDB 3.10
*/
ViewEntity createSearchAlias(String name, SearchAliasCreateOptions options);
/**
* Creates an Analyzer
*
* @param analyzer SearchAnalyzer
* @return the created Analyzer
* @see API Documentation
* @since ArangoDB 3.5.0
*/
SearchAnalyzer createSearchAnalyzer(SearchAnalyzer analyzer);
/**
* Gets information about an Analyzer
*
* @param name of the Analyzer without database prefix
* @return information about an Analyzer
* @see API Documentation
* @since ArangoDB 3.5.0
*/
SearchAnalyzer getSearchAnalyzer(String name);
/**
* Retrieves all analyzers definitions.
*
* @return collection of all analyzers definitions
* @see API Documentation
* @since ArangoDB 3.5.0
*/
Collection getSearchAnalyzers();
/**
* Deletes an Analyzer
*
* @param name of the Analyzer without database prefix
* @see API Documentation
* @since ArangoDB 3.5.0
*/
void deleteSearchAnalyzer(String name);
/**
* Deletes an Analyzer
*
* @param name of the Analyzer without database prefix
* @param options AnalyzerDeleteOptions
* @see API Documentation
* @since ArangoDB 3.5.0
*/
void deleteSearchAnalyzer(String name, AnalyzerDeleteOptions options);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy