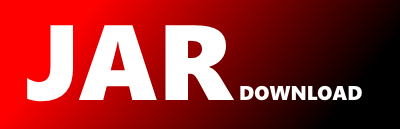
com.arangodb.entity.AqlExecutionExplainEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core module for ArangoDB Java Driver
/*
* DISCLAIMER
*
* Copyright 2016 ArangoDB GmbH, Cologne, Germany
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Copyright holder is ArangoDB GmbH, Cologne, Germany
*/
package com.arangodb.entity;
import com.arangodb.ArangoDatabase;
import com.arangodb.model.ExplainAqlQueryOptions;
import java.util.Collection;
import java.util.Map;
/**
* @author Mark Vollmary
* @deprecated for removal, use {@link ArangoDatabase#explainAqlQuery(String, Map, ExplainAqlQueryOptions)} instead
*/
@Deprecated
public final class AqlExecutionExplainEntity {
private ExecutionPlan plan;
private Collection plans;
private Collection warnings;
private ExecutionStats stats;
private Boolean cacheable;
public ExecutionPlan getPlan() {
return plan;
}
public Collection getPlans() {
return plans;
}
public Collection getWarnings() {
return warnings;
}
public ExecutionStats getStats() {
return stats;
}
public Boolean getCacheable() {
return cacheable;
}
public static final class ExecutionPlan {
private Collection nodes;
private Collection rules;
private Collection collections;
private Collection variables;
private Integer estimatedCost;
private Integer estimatedNrItems;
public Collection getNodes() {
return nodes;
}
public Collection getRules() {
return rules;
}
public Collection getCollections() {
return collections;
}
public Collection getVariables() {
return variables;
}
public Integer getEstimatedCost() {
return estimatedCost;
}
public Integer getEstimatedNrItems() {
return estimatedNrItems;
}
}
public static final class ExecutionNode {
private String type;
private Collection dependencies;
private Long id;
private Integer estimatedCost;
private Integer estimatedNrItems;
private Long depth;
private String database;
private String collection;
private ExecutionVariable inVariable;
private ExecutionVariable outVariable;
private ExecutionVariable conditionVariable;
private Boolean random;
private Long offset;
private Long limit;
private Boolean fullCount;
private ExecutionNode subquery;
private Boolean isConst;
private Boolean canThrow;
private String expressionType;
private Collection indexes;
private ExecutionExpression expression;
private ExecutionCollection condition;
private Boolean reverse;
public String getType() {
return type;
}
public Collection getDependencies() {
return dependencies;
}
public Long getId() {
return id;
}
public Integer getEstimatedCost() {
return estimatedCost;
}
public Integer getEstimatedNrItems() {
return estimatedNrItems;
}
public Long getDepth() {
return depth;
}
public String getDatabase() {
return database;
}
public String getCollection() {
return collection;
}
public ExecutionVariable getInVariable() {
return inVariable;
}
public ExecutionVariable getOutVariable() {
return outVariable;
}
public ExecutionVariable getConditionVariable() {
return conditionVariable;
}
public Boolean getRandom() {
return random;
}
public Long getOffset() {
return offset;
}
public Long getLimit() {
return limit;
}
public Boolean getFullCount() {
return fullCount;
}
public ExecutionNode getSubquery() {
return subquery;
}
public Boolean getIsConst() {
return isConst;
}
public Boolean getCanThrow() {
return canThrow;
}
public String getExpressionType() {
return expressionType;
}
public Collection getIndexes() {
return indexes;
}
public ExecutionExpression getExpression() {
return expression;
}
public ExecutionCollection getCondition() {
return condition;
}
public Boolean getReverse() {
return reverse;
}
}
public static final class ExecutionVariable {
private Long id;
private String name;
public Long getId() {
return id;
}
public String getName() {
return name;
}
}
public static final class ExecutionExpression {
private String type;
private String name;
private Long id;
private Object value;
private Boolean sorted;
private String quantifier;
private Collection levels;
private Collection subNodes;
public String getType() {
return type;
}
public String getName() {
return name;
}
public Long getId() {
return id;
}
public Object getValue() {
return value;
}
public Boolean getSorted() {
return sorted;
}
public String getQuantifier() {
return quantifier;
}
public Collection getLevels() {
return levels;
}
public Collection getSubNodes() {
return subNodes;
}
}
public static final class ExecutionCollection {
private String name;
private String type;
public String getName() {
return name;
}
public String getType() {
return type;
}
}
public static final class ExecutionStats {
private Integer rulesExecuted;
private Integer rulesSkipped;
private Integer plansCreated;
private Long peakMemoryUsage;
private Double executionTime;
public Integer getRulesExecuted() {
return rulesExecuted;
}
public Integer getRulesSkipped() {
return rulesSkipped;
}
public Integer getPlansCreated() {
return plansCreated;
}
public Long getPeakMemoryUsage() {
return peakMemoryUsage;
}
public Double getExecutionTime() {
return executionTime;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy