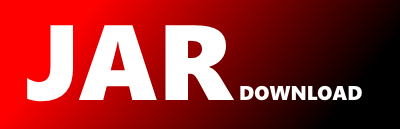
com.arassec.igor.module.file.action.CopyFileAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of module-file Show documentation
Show all versions of module-file Show documentation
Provides file handling for igor.
The newest version!
package com.arassec.igor.module.file.action;
import com.arassec.igor.core.model.annotation.IgorComponent;
import com.arassec.igor.core.model.annotation.IgorParam;
import com.arassec.igor.core.model.job.execution.JobExecution;
import com.arassec.igor.core.model.job.execution.WorkInProgressMonitor;
import com.arassec.igor.core.util.IgorException;
import com.arassec.igor.module.file.connector.FallbackFileConnector;
import com.arassec.igor.module.file.connector.FileConnector;
import com.arassec.igor.module.file.connector.FileStreamData;
import lombok.Getter;
import lombok.Setter;
import lombok.extern.slf4j.Slf4j;
import org.springframework.util.StringUtils;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Copies a file from one connector to another.
*/
@Slf4j
@Setter
@Getter
@IgorComponent
public class CopyFileAction extends BaseFileAction {
/**
* Key for the copy file action's data.
*/
public static final String KEY_COPY_FILE_ACTION = "copiedFile";
/**
* Key to the source file's name.
*/
public static final String KEY_SOURCE_FILENAME = "sourceFilename";
/**
* Key to the source file's directory.
*/
public static final String KEY_SOURCE_DIRECTORY = "sourceDirectory";
/**
* Key to the target file's name.
*/
public static final String KEY_TARGET_FILENAME = "targetFilename";
/**
* Key to the target directory.
*/
public static final String KEY_TARGET_DIRECTORY = "targetDirectory";
/**
* File-suffix appended to files during transfer.
*/
private static final String IN_TRANSFER_SUFFIX = ".igor";
/**
* The connector providing the file to copy.
*/
@NotNull
@IgorParam
private FileConnector source;
/**
* Source directory to copy the file from.
*/
@NotBlank
@IgorParam(defaultValue = DIRECTORY_TEMPLATE)
private String sourceDirectory;
/**
* Source file to copy.
*/
@NotBlank
@IgorParam(defaultValue = FILENAME_TEMPLATE)
private String sourceFilename;
/**
* The destination for the copied file.
*/
@NotNull
@IgorParam
private FileConnector target;
/**
* The target directory to copy/move the file to.
*/
@NotBlank
@IgorParam
private String targetDirectory;
/**
* The target file name.
*/
@NotBlank
@IgorParam
private String targetFilename;
/**
* Enables a ".igor" file suffix during file transfer. The suffix will be removed after the file has been copied completely.
*/
@IgorParam(advanced = true)
private boolean appendTransferSuffix = true;
/**
* If set to {@code true}, igor appends a filetype suffix if avaliable (e.g. '.html' or '.jpeg').
*/
@IgorParam(advanced = true)
private boolean appendFiletypeSuffix = false;
/**
* Creates a new component instance.
*/
public CopyFileAction() {
super("copy-file-action");
source = new FallbackFileConnector();
target = new FallbackFileConnector();
}
/**
* Copies the supplied source file to the destination connector. During transfer the file is optionally saved with the suffix
* ".igor", which will be removed after successful transfer.
*
* @param data The data to process.
* @param jobExecution The job execution log.
*
* @return The manipulated data.
*/
@Override
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy