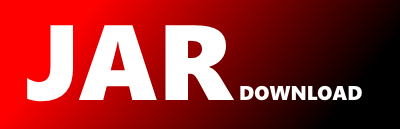
com.arcadedb.database.DetachedDocument Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
package com.arcadedb.database;
import com.arcadedb.serializer.json.JSONObject;
import java.util.*;
public class DetachedDocument extends ImmutableDocument {
private Map map;
protected DetachedDocument(final BaseDocument source) {
super(null, source.type, source.rid, null);
init(source);
}
private void init(final Document sourceDocument) {
this.map = new LinkedHashMap<>();
final Map sourceMap = sourceDocument.propertiesAsMap();
for (final Map.Entry entry : sourceMap.entrySet()) {
Object value = entry.getValue();
if (value instanceof List) {
for (int i = 0; i < ((List) value).size(); i++) {
final Object embValue = ((List) value).get(i);
if (embValue instanceof EmbeddedDocument)
((List) value).set(i, ((EmbeddedDocument) embValue).detach());
}
} else if (value instanceof Map) {
final Map map = (Map) value;
for (final String propName : map.keySet()) {
final Object embValue = map.get(propName);
if (embValue instanceof EmbeddedDocument)
map.put(propName, ((EmbeddedDocument) embValue).detach());
}
} else if (value instanceof EmbeddedDocument)
value = ((EmbeddedDocument) value).detach();
this.map.put(entry.getKey(), value);
}
}
@Override
public synchronized MutableDocument modify() {
throw new UnsupportedOperationException("Detached document cannot be modified. Get a new regular object from the database by its id to modify it");
}
@Override
public synchronized Map toMap(final boolean includeMetadata) {
final Map result = new HashMap<>(map);
if (includeMetadata) {
result.put("@cat", "d");
result.put("@type", type.getName());
if (getIdentity() != null)
result.put("@rid", getIdentity().toString());
}
return result;
}
@Override
public synchronized JSONObject toJSON(final boolean includeMetadata) {
final JSONObject result = new JSONSerializer(database).map2json(map);
if (includeMetadata) {
result.put("@cat", "d");
result.put("@type", type.getName());
if (getIdentity() != null)
result.put("@rid", getIdentity().toString());
}
return result;
}
@Override
public synchronized boolean has(final String propertyName) {
return map.containsKey(propertyName);
}
public synchronized Object get(final String propertyName) {
return map.get(propertyName);
}
@Override
public void setBuffer(final Binary buffer) {
throw new UnsupportedOperationException("setBuffer");
}
@Override
public synchronized String toString() {
final StringBuilder result = new StringBuilder(256);
if (rid != null)
result.append(rid);
if (type != null) {
result.append('@');
result.append(type.getName());
}
result.append('[');
if (map == null) {
result.append('?');
} else {
int i = 0;
for (final Map.Entry entry : map.entrySet()) {
if (i > 0)
result.append(',');
result.append(entry.getKey());
result.append('=');
result.append(entry.getValue());
i++;
}
}
result.append(']');
return result.toString();
}
@Override
public synchronized Set getPropertyNames() {
return map.keySet();
}
@Override
public void reload() {
map = null;
buffer = null;
super.reload();
init(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy