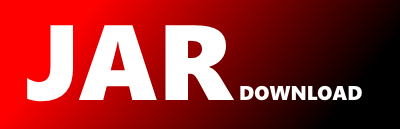
com.arcadedb.query.sql.parser.ArrayConcatExpression Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. OArrayConcatExpression.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Identifiable;
import com.arcadedb.exception.CommandExecutionException;
import com.arcadedb.query.sql.executor.AggregationContext;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.MultiValue;
import com.arcadedb.query.sql.executor.Result;
import java.util.*;
public class ArrayConcatExpression extends SimpleNode {
List childExpressions = new ArrayList<>();
public ArrayConcatExpression(final int id) {
super(id);
}
public List getChildExpressions() {
return childExpressions;
}
public void setChildExpressions(final List childExpressions) {
this.childExpressions = childExpressions;
}
public Object apply(final Object left, final Object right) {
if (left == null && right == null) {
return null;
}
if (right == null) {
if (MultiValue.isMultiValue(left)) {
return left;
} else {
return Collections.singletonList(left);
}
}
if (left == null) {
if (MultiValue.isMultiValue(right)) {
return right;
} else {
return Collections.singletonList(right);
}
}
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy