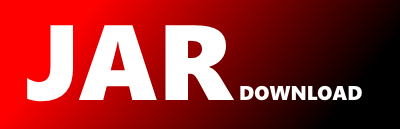
com.arcadedb.query.sql.parser.DeleteEdgeStatement Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. ODeleteEdgeStatement.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Database;
import com.arcadedb.exception.ArcadeDBException;
import com.arcadedb.query.sql.executor.BasicCommandContext;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.DeleteEdgeExecutionPlanner;
import com.arcadedb.query.sql.executor.DeleteExecutionPlan;
import com.arcadedb.query.sql.executor.ResultSet;
import java.util.*;
import java.util.stream.*;
public class DeleteEdgeStatement extends Statement {
protected Identifier typeName;
protected Identifier targetBucketName;
protected Rid rid;
protected List rids;
protected Expression leftExpression;
protected Expression rightExpression;
protected WhereClause whereClause;
public DeleteEdgeStatement(final int id) {
super(id);
}
@Override
public ResultSet execute(final Database db, final Map params, final CommandContext parentcontext, final boolean usePlanCache) {
final BasicCommandContext context = new BasicCommandContext();
if (parentcontext != null) {
context.setParentWithoutOverridingChild(parentcontext);
}
context.setDatabase(db);
context.setInputParameters(params);
final DeleteExecutionPlan executionPlan = createExecutionPlan(context, false);
executionPlan.executeInternal();
return new LocalResultSet(executionPlan);
}
@Override
public ResultSet execute(final Database db, final Object[] args, final CommandContext parentcontext, final boolean usePlanCache) {
final Map params = new HashMap<>();
if (args != null) {
for (int i = 0; i < args.length; i++) {
params.put(String.valueOf(i), args[i]);
}
}
return execute(db, params, parentcontext);
}
public DeleteExecutionPlan createExecutionPlan(final CommandContext context, final boolean enableProfiling) {
final DeleteEdgeExecutionPlanner planner = new DeleteEdgeExecutionPlanner(this);
return planner.createExecutionPlan(context, enableProfiling);
}
public void toString(final Map params, final StringBuilder builder) {
builder.append("DELETE EDGE");
if (typeName != null) {
builder.append(" ");
typeName.toString(params, builder);
if (targetBucketName != null) {
builder.append(" BUCKET ");
targetBucketName.toString(params, builder);
}
}
if (rid != null) {
builder.append(" ");
rid.toString(params, builder);
}
if (rids != null) {
builder.append(" [");
boolean first = true;
for (final Rid rid : rids) {
if (!first) {
builder.append(", ");
}
rid.toString(params, builder);
first = false;
}
builder.append("]");
}
if (leftExpression != null) {
builder.append(" FROM ");
leftExpression.toString(params, builder);
}
if (rightExpression != null) {
builder.append(" TO ");
rightExpression.toString(params, builder);
}
if (whereClause != null) {
builder.append(" WHERE ");
whereClause.toString(params, builder);
}
if (limit != null) {
limit.toString(params, builder);
}
}
@Override
public DeleteEdgeStatement copy() {
final DeleteEdgeStatement result;
try {
result = getClass().getConstructor(Integer.TYPE).newInstance(-1);
result.typeName = typeName == null ? null : typeName.copy();
result.targetBucketName = targetBucketName == null ? null : targetBucketName.copy();
result.rid = rid == null ? null : rid.copy();
result.rids = rids == null ? null : rids.stream().map(x -> x.copy()).collect(Collectors.toList());
result.leftExpression = leftExpression == null ? null : leftExpression.copy();
result.rightExpression = rightExpression == null ? null : rightExpression.copy();
result.whereClause = whereClause == null ? null : whereClause.copy();
result.limit = limit == null ? null : limit.copy();
return result;
} catch (final Exception e) {
throw new ArcadeDBException(e);
}
}
@Override
public boolean equals(final Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
final DeleteEdgeStatement that = (DeleteEdgeStatement) o;
if (!Objects.equals(typeName, that.typeName))
return false;
if (!Objects.equals(targetBucketName, that.targetBucketName))
return false;
if (!Objects.equals(rid, that.rid))
return false;
if (!Objects.equals(rids, that.rids))
return false;
if (!Objects.equals(leftExpression, that.leftExpression))
return false;
if (!Objects.equals(rightExpression, that.rightExpression))
return false;
if (!Objects.equals(whereClause, that.whereClause))
return false;
return Objects.equals(limit, that.limit);
}
@Override
public int hashCode() {
int result = typeName != null ? typeName.hashCode() : 0;
result = 31 * result + (targetBucketName != null ? targetBucketName.hashCode() : 0);
result = 31 * result + (rid != null ? rid.hashCode() : 0);
result = 31 * result + (rids != null ? rids.hashCode() : 0);
result = 31 * result + (leftExpression != null ? leftExpression.hashCode() : 0);
result = 31 * result + (rightExpression != null ? rightExpression.hashCode() : 0);
result = 31 * result + (whereClause != null ? whereClause.hashCode() : 0);
result = 31 * result + (limit != null ? limit.hashCode() : 0);
return result;
}
public Identifier getTypeName() {
return typeName;
}
public Identifier getTargetBucketName() {
return targetBucketName;
}
public Rid getRid() {
return rid;
}
public List getRids() {
return rids;
}
public WhereClause getWhereClause() {
return whereClause;
}
public Expression getLeftExpression() {
return leftExpression;
}
public Expression getRightExpression() {
return rightExpression;
}
}
/* JavaCC - OriginalChecksum=8f4c5bafa99572d7d87a5d0a2c7d55a7 (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy