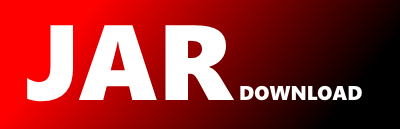
com.arcadedb.query.sql.parser.DropPropertyStatement Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. ODropPropertyStatement.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Database;
import com.arcadedb.exception.CommandExecutionException;
import com.arcadedb.index.Index;
import com.arcadedb.index.TypeIndex;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.InternalResultSet;
import com.arcadedb.query.sql.executor.ResultInternal;
import com.arcadedb.query.sql.executor.ResultSet;
import com.arcadedb.schema.DocumentType;
import java.util.*;
public class DropPropertyStatement extends DDLStatement {
protected Identifier typeName;
protected Identifier propertyName;
protected boolean ifExists = false;
protected boolean force = false;
public DropPropertyStatement(final int id) {
super(id);
}
@Override
public ResultSet executeDDL(final CommandContext context) {
final InternalResultSet rs = new InternalResultSet();
final Database database = context.getDatabase();
final DocumentType sourceClass = database.getSchema().getType(typeName.getStringValue());
if (sourceClass == null)
throw new CommandExecutionException("Source class '" + typeName + "' not found");
if (sourceClass.getProperty(propertyName.getStringValue()) == null) {
if (ifExists) {
return rs;
}
throw new CommandExecutionException("Property '" + propertyName + "' not found on class " + typeName);
}
final List indexes = sourceClass.getIndexesByProperties(propertyName.getStringValue());
if (!indexes.isEmpty()) {
if (force) {
for (final Index index : indexes) {
database.getSchema().dropIndex(index.getName());
final ResultInternal result = new ResultInternal();
result.setProperty("operation", "cascade drop index");
result.setProperty("indexName", index.getName());
rs.add(result);
}
} else {
final StringBuilder indexNames = new StringBuilder();
boolean first = true;
for (final TypeIndex index : indexes) {
if (!first) {
indexNames.append(", ");
} else {
first = false;
}
indexNames.append(index.getName());
}
throw new CommandExecutionException(
"Property used in indexes (" + indexNames + "). Please drop these indexes before removing property or use FORCE parameter.");
}
}
// REMOVE THE PROPERTY
sourceClass.dropProperty(propertyName.getStringValue());
final ResultInternal result = new ResultInternal();
result.setProperty("operation", "drop property");
result.setProperty("typeName", typeName.getStringValue());
result.setProperty("propertyName", propertyName.getStringValue());
rs.add(result);
return rs;
}
@Override
public void toString(final Map params, final StringBuilder builder) {
builder.append("DROP PROPERTY ");
typeName.toString(params, builder);
builder.append(".");
propertyName.toString(params, builder);
if (ifExists) {
builder.append(" IF EXISTS");
}
if (force) {
builder.append(" FORCE");
}
}
@Override
public DropPropertyStatement copy() {
final DropPropertyStatement result = new DropPropertyStatement(-1);
result.typeName = typeName == null ? null : typeName.copy();
result.propertyName = propertyName == null ? null : propertyName.copy();
result.force = force;
result.ifExists = ifExists;
return result;
}
@Override
public boolean equals( final Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
final DropPropertyStatement that = (DropPropertyStatement) o;
if (force != that.force)
return false;
if (ifExists != that.ifExists) {
return false;
}
if (!Objects.equals(typeName, that.typeName))
return false;
return Objects.equals(propertyName, that.propertyName);
}
@Override
public int hashCode() {
int result = typeName != null ? typeName.hashCode() : 0;
result = 31 * result + (propertyName != null ? propertyName.hashCode() : 0);
result = 31 * result + (force ? 1 : 0);
return result;
}
}
/* JavaCC - OriginalChecksum=6a9b4b1694dc36caf2b801218faebe42 (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy