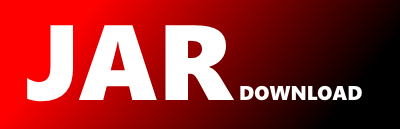
com.arcadedb.query.sql.parser.ForEachBlock Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. OForEachBlock.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Database;
import com.arcadedb.query.sql.executor.BasicCommandContext;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.ForEachExecutionPlan;
import com.arcadedb.query.sql.executor.ForEachStep;
import com.arcadedb.query.sql.executor.GlobalLetExpressionStep;
import com.arcadedb.query.sql.executor.ResultSet;
import com.arcadedb.query.sql.executor.UpdateExecutionPlan;
import java.util.*;
import java.util.concurrent.atomic.*;
import java.util.stream.*;
//import com.orientechnologies.orient.core.sql.executor.LetExpressionStep;
public class ForEachBlock extends Statement {
protected static final AtomicInteger FOREACH_VARIABLE_PROGR = new AtomicInteger();
protected Identifier loopVariable;
protected Expression loopValues;
protected List statements = new ArrayList<>();
public ForEachBlock(final int id) {
super(id);
}
@Override
public ResultSet execute(final Database db, final Object[] args, final CommandContext parentcontext, final boolean usePlanCache) {
final BasicCommandContext context = new BasicCommandContext();
if (parentcontext != null)
context.setParentWithoutOverridingChild(parentcontext);
context.setDatabase(db);
context.setInputParameters(args);
final UpdateExecutionPlan executionPlan = createExecutionPlan(context, false);
executionPlan.executeInternal();
return new LocalResultSet(executionPlan);
}
@Override
public ResultSet execute(final Database db, final Map params, final CommandContext parentcontext, final boolean usePlanCache) {
final BasicCommandContext context = new BasicCommandContext();
if (parentcontext != null)
context.setParentWithoutOverridingChild(parentcontext);
context.setDatabase(db);
context.setInputParameters(params);
final UpdateExecutionPlan executionPlan = createExecutionPlan(context, false);
executionPlan.executeInternal();
return new LocalResultSet(executionPlan);
}
public UpdateExecutionPlan createExecutionPlan(final CommandContext context, final boolean enableProfiling) {
ForEachExecutionPlan plan = new ForEachExecutionPlan(context);
int nextProg = FOREACH_VARIABLE_PROGR.incrementAndGet();
if (nextProg < 0)
FOREACH_VARIABLE_PROGR.set(0);
Identifier varName = new Identifier("$__ARCADEDB_FOREACH_VAR_" + nextProg);
plan.chain(new GlobalLetExpressionStep(varName, loopValues, context, enableProfiling));
plan.chain(new ForEachStep(loopVariable, new Expression(varName), statements, context, enableProfiling));
return plan;
}
@Override
public Statement copy() {
final ForEachBlock result = new ForEachBlock(-1);
result.loopVariable = loopVariable.copy();
result.loopValues = loopValues.copy();
result.statements = statements.stream().map(x -> x.copy()).collect(Collectors.toList());
return result;
}
@Override
public boolean equals(final Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
final ForEachBlock that = (ForEachBlock) o;
if (!Objects.equals(loopVariable, that.loopVariable))
return false;
if (!Objects.equals(loopValues, that.loopValues))
return false;
return Objects.equals(statements, that.statements);
}
@Override
public int hashCode() {
int result = loopVariable != null ? loopVariable.hashCode() : 0;
result = 31 * result + (loopValues != null ? loopValues.hashCode() : 0);
result = 31 * result + (statements != null ? statements.hashCode() : 0);
return result;
}
@Override
public void toString(final Map params, final StringBuilder builder) {
builder.append("FOREACH (");
loopVariable.toString(params, builder);
builder.append(" IN ");
loopValues.toString(params, builder);
builder.append(") {\n");
for (Statement stm : statements) {
stm.toString(params, builder);
builder.append("\n");
}
builder.append("}");
}
public boolean containsReturn() {
for (final Statement stm : this.statements) {
if (stm instanceof ReturnStatement) {
return true;
}
if (stm instanceof ForEachBlock && ((ForEachBlock) stm).containsReturn()) {
return true;
}
if (stm instanceof IfStatement && ((IfStatement) stm).containsReturn()) {
return true;
}
}
return false;
}
}
/* JavaCC - OriginalChecksum=071053b057a38c57f3c90d28399615d0 (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy