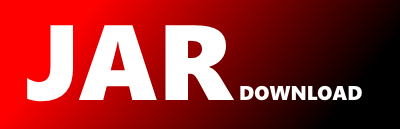
com.arcadedb.query.sql.parser.IfStatement Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. IfStatement.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Database;
import com.arcadedb.query.sql.executor.BasicCommandContext;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.EmptyStep;
import com.arcadedb.query.sql.executor.ExecutionStepInternal;
import com.arcadedb.query.sql.executor.IfExecutionPlan;
import com.arcadedb.query.sql.executor.IfStep;
import com.arcadedb.query.sql.executor.ResultSet;
import com.arcadedb.query.sql.executor.SelectExecutionPlan;
import com.arcadedb.query.sql.executor.UpdateExecutionPlan;
import java.util.*;
import java.util.stream.*;
public class IfStatement extends Statement {
protected BooleanExpression expression;
protected List statements = new ArrayList();
protected List elseStatements = new ArrayList();//TODO support ELSE in the SQL syntax
public IfStatement(final int id) {
super(id);
}
@Override
public boolean isIdempotent() {
for (final Statement stm : statements) {
if (!stm.isIdempotent()) {
return false;
}
}
for (final Statement stm : elseStatements) {
if (!stm.isIdempotent()) {
return false;
}
}
return true;
}
@Override
public ResultSet execute(final Database db, final Object[] args, final CommandContext parentcontext, final boolean usePlanCache) {
final BasicCommandContext context = new BasicCommandContext();
if (parentcontext != null) {
context.setParentWithoutOverridingChild(parentcontext);
}
context.setDatabase(db);
context.setInputParameters(args);
final IfExecutionPlan executionPlan;
if (usePlanCache) {
executionPlan = createExecutionPlan(context, false);
} else {
executionPlan = (IfExecutionPlan) createExecutionPlanNoCache(context, false);
}
ExecutionStepInternal last = executionPlan.executeUntilReturn();
if (last == null) {
last = new EmptyStep(context, false);
}
if (isIdempotent()) {
final SelectExecutionPlan finalPlan = new SelectExecutionPlan(context);
finalPlan.chain(last);
return new LocalResultSet(finalPlan);
} else {
final UpdateExecutionPlan finalPlan = new UpdateExecutionPlan(context);
finalPlan.chain(last);
finalPlan.executeInternal();
return new LocalResultSet(finalPlan);
}
}
@Override
public ResultSet execute(final Database db, final Map params, final CommandContext parentcontext, final boolean usePlanCache) {
final BasicCommandContext context = new BasicCommandContext();
if (parentcontext != null) {
context.setParentWithoutOverridingChild(parentcontext);
}
context.setDatabase(db);
context.setInputParameters(params);
final IfExecutionPlan executionPlan;
if (usePlanCache) {
executionPlan = createExecutionPlan(context, false);
} else {
executionPlan = (IfExecutionPlan) createExecutionPlanNoCache(context, false);
}
ExecutionStepInternal last = executionPlan.executeUntilReturn();
if (last == null) {
last = new EmptyStep(context, false);
}
if (isIdempotent()) {
final SelectExecutionPlan finalPlan = new SelectExecutionPlan(context);
finalPlan.chain(last);
return new LocalResultSet(finalPlan);
} else {
final UpdateExecutionPlan finalPlan = new UpdateExecutionPlan(context);
finalPlan.chain(last);
finalPlan.executeInternal();
return new LocalResultSet(finalPlan);
}
}
@Override
public IfExecutionPlan createExecutionPlan(final CommandContext context, final boolean enableProfiling) {
final IfExecutionPlan plan = new IfExecutionPlan(context);
final IfStep step = new IfStep(context, enableProfiling);
step.setCondition(this.expression);
plan.chain(step);
step.positiveStatements = statements;
step.negativeStatements = elseStatements;
return plan;
}
@Override
public void toString(final Map params, final StringBuilder builder) {
builder.append("IF(");
expression.toString(params, builder);
builder.append("){\n");
for (final Statement stm : statements) {
stm.toString(params, builder);
builder.append(";\n");
}
builder.append("}");
if (elseStatements.size() > 0) {
builder.append("\nELSE {\n");
for (final Statement stm : elseStatements) {
stm.toString(params, builder);
builder.append(";\n");
}
builder.append("}");
}
}
@Override
public IfStatement copy() {
final IfStatement result = new IfStatement(-1);
result.expression = expression == null ? null : expression.copy();
result.statements = statements == null ? null : statements.stream().map(Statement::copy).collect(Collectors.toList());
result.elseStatements = elseStatements == null ? null : elseStatements.stream().map(Statement::copy).collect(Collectors.toList());
return result;
}
@Override
protected Object[] getIdentityElements() {
return new Object[] { expression, statements, elseStatements };
}
public List getStatements() {
return statements;
}
public boolean containsReturn() {
for (final Statement stm : this.statements) {
if (stm instanceof ReturnStatement) {
return true;
}
if (stm instanceof ForEachBlock && ((ForEachBlock) stm).containsReturn()) {
return true;
}
if (stm instanceof IfStatement && ((IfStatement) stm).containsReturn()) {
return true;
}
}
if (elseStatements != null) {
for (final Statement stm : this.elseStatements) {
if (stm instanceof ReturnStatement) {
return true;
}
if (stm instanceof ForEachBlock && ((ForEachBlock) stm).containsReturn()) {
return true;
}
if (stm instanceof IfStatement && ((IfStatement) stm).containsReturn()) {
return true;
}
}
}
return false;
}
}
/* JavaCC - OriginalChecksum=a8cd4fb832a4f3b6e71bb1a12f8d8819 (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy