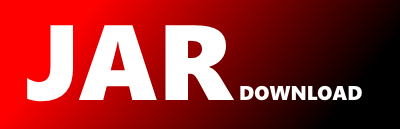
com.arcadedb.query.sql.parser.InsertBody Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. OInsertBody.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import java.util.*;
import java.util.stream.*;
public class InsertBody extends SimpleNode {
protected List identifierList;
protected List> valueExpressions;
protected List setExpressions;
protected Json contentJson;
protected JsonArray contentArray;
protected InputParameter contentInputParam;
public InsertBody(final int id) {
super(id);
}
public void toString(final Map params, final StringBuilder builder) {
if (identifierList != null) {
builder.append("(");
boolean first = true;
for (final Identifier item : identifierList) {
if (!first) {
builder.append(", ");
}
item.toString(params, builder);
first = false;
}
builder.append(") VALUES ");
if (valueExpressions != null) {
boolean firstList = true;
for (final List itemList : valueExpressions) {
if (firstList) {
builder.append("(");
} else {
builder.append("),(");
}
first = true;
for (final Expression item : itemList) {
if (!first) {
builder.append(", ");
}
item.toString(params, builder);
first = false;
}
firstList = false;
}
}
builder.append(")");
}
if (setExpressions != null) {
builder.append("SET ");
boolean first = true;
for (final InsertSetExpression item : setExpressions) {
if (!first) {
builder.append(", ");
}
item.toString(params, builder);
first = false;
}
}
if (contentJson != null) {
builder.append("CONTENT ");
contentJson.toString(params, builder);
} else if (contentArray != null) {
builder.append("CONTENT ");
contentArray.toString(params, builder);
} else if (contentInputParam != null) {
builder.append("CONTENT ");
contentInputParam.toString(params, builder);
}
}
public InsertBody copy() {
final InsertBody result = new InsertBody(-1);
result.identifierList = identifierList == null ? null : identifierList.stream().map(x -> x.copy()).collect(Collectors.toList());
result.valueExpressions = valueExpressions == null ?
null :
valueExpressions.stream().map(sub -> sub.stream().map(x -> x.copy()).collect(Collectors.toList()))
.collect(Collectors.toList());
result.setExpressions = setExpressions == null ? null : setExpressions.stream().map(x -> x.copy()).collect(Collectors.toList());
result.contentJson = contentJson == null ? null : contentJson.copy();
result.contentArray = contentArray == null ? null : contentArray.copy();
result.contentInputParam = contentInputParam == null ? null : contentInputParam.copy();
return result;
}
@Override
public boolean equals(final Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
final InsertBody that = (InsertBody) o;
if (!Objects.equals(identifierList, that.identifierList))
return false;
if (!Objects.equals(valueExpressions, that.valueExpressions))
return false;
if (!Objects.equals(setExpressions, that.setExpressions))
return false;
if (!Objects.equals(contentJson, that.contentJson))
return false;
if (!Objects.equals(contentArray, that.contentArray))
return false;
return Objects.equals(contentInputParam, that.contentInputParam);
}
@Override
public int hashCode() {
int result = identifierList != null ? identifierList.hashCode() : 0;
result = 31 * result + (valueExpressions != null ? valueExpressions.hashCode() : 0);
result = 31 * result + (setExpressions != null ? setExpressions.hashCode() : 0);
result = 31 * result + (contentJson != null ? contentJson.hashCode() : 0);
result = 31 * result + (contentArray != null ? contentArray.hashCode() : 0);
result = 31 * result + (contentInputParam != null ? contentInputParam.hashCode() : 0);
return result;
}
public List getIdentifierList() {
return identifierList;
}
public List> getValueExpressions() {
return valueExpressions;
}
public List getSetExpressions() {
return setExpressions;
}
public Json getJsonContent() {
return contentJson;
}
public JsonArray getJsonArrayContent() {
return contentArray;
}
public InputParameter getContentInputParam() {
return contentInputParam;
}
}
/* JavaCC - OriginalChecksum=7d2079a41a1fc63a812cb679e729b23a (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy