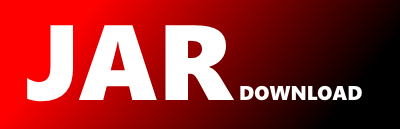
com.arcadedb.query.sql.parser.ParenthesisExpression Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. OParenthesisExpression.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import com.arcadedb.database.Identifiable;
import com.arcadedb.exception.CommandExecutionException;
import com.arcadedb.query.sql.executor.BasicCommandContext;
import com.arcadedb.query.sql.executor.CommandContext;
import com.arcadedb.query.sql.executor.InsertExecutionPlan;
import com.arcadedb.query.sql.executor.InternalExecutionPlan;
import com.arcadedb.query.sql.executor.Result;
import com.arcadedb.query.sql.executor.ResultInternal;
import java.util.*;
public class ParenthesisExpression extends MathExpression {
protected Expression expression;
protected Statement statement;
public ParenthesisExpression(final int id) {
super(id);
}
public ParenthesisExpression(final Expression exp) {
super(-1);
this.expression = exp;
}
@Override
public Object execute(final Identifiable iCurrentRecord, final CommandContext context) {
if (expression != null) {
return expression.execute(iCurrentRecord, context);
}
if (statement != null) {
throw new UnsupportedOperationException("Execution of select in parentheses is not supported");
}
return super.execute(iCurrentRecord, context);
}
@Override
public Object execute(final Result iCurrentRecord, final CommandContext context) {
if (expression != null) {
return expression.execute(iCurrentRecord, context);
}
if (statement != null) {
final BasicCommandContext subCtx = new BasicCommandContext();
subCtx.setDatabase(context.getDatabase());
subCtx.setParent(context);
final InternalExecutionPlan execPlan = statement.createExecutionPlan(subCtx, false);
if (execPlan instanceof InsertExecutionPlan) {
((InsertExecutionPlan) execPlan).executeInternal();
}
final LocalResultSet rs = new LocalResultSet(execPlan);
final List result = new ArrayList<>();
while (rs.hasNext()) {
result.add(rs.next());
}
// List result = rs.stream().collect(Collectors.toList());//TODO streamed...
rs.close();
return result;
}
return super.execute(iCurrentRecord, context);
}
public void toString(final Map params, final StringBuilder builder) {
builder.append("(");
if (expression != null) {
expression.toString(params, builder);
} else if (statement != null) {
statement.toString(params, builder);
}
builder.append(")");
}
public boolean isExpand() {
if (expression != null) {
return expression.isExpand();
}
return false;
}
public boolean isAggregate(CommandContext context) {
if (expression != null) {
return expression.isAggregate(context);
}
return false;
}
public boolean isCount() {
if (expression != null)
return expression.isCount();
return false;
}
@Override
public boolean isEarlyCalculated(final CommandContext context) {
// TODO implement query execution and early calculation;
return expression != null && expression.isEarlyCalculated(context);
}
public SimpleNode splitForAggregation(final AggregateProjectionSplit aggregateProj, final CommandContext context) {
if (isAggregate(context)) {
final ParenthesisExpression result = new ParenthesisExpression(-1);
result.expression = expression.splitForAggregation(aggregateProj, context);
return result;
} else {
return this;
}
}
@Override
public ParenthesisExpression copy() {
final ParenthesisExpression result = new ParenthesisExpression(-1);
result.expression = expression == null ? null : expression.copy();
result.statement = statement == null ? null : statement.copy();
result.cachedStringForm = cachedStringForm;
return result;
}
public void setStatement(final Statement statement) {
this.statement = statement;
}
public void extractSubQueries(final SubQueryCollector collector) {
if (expression != null) {
expression.extractSubQueries(collector);
} else if (statement != null) {
final Identifier alias = collector.addStatement(statement);
statement = null;
expression = new Expression(alias);
}
}
public void extractSubQueries(final Identifier letAlias, final SubQueryCollector collector) {
if (expression != null) {
expression.extractSubQueries(collector);
} else if (statement != null) {
final Identifier alias = collector.addStatement(letAlias, statement);
statement = null;
expression = new Expression(alias);
}
}
public boolean refersToParent() {
if (expression != null && expression.refersToParent()) {
return true;
}
return statement != null && statement.refersToParent();
}
@Override
public boolean equals(final Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
if (!super.equals(o))
return false;
final ParenthesisExpression that = (ParenthesisExpression) o;
if (!Objects.equals(expression, that.expression))
return false;
return Objects.equals(statement, that.statement);
}
@Override
public int hashCode() {
int result = super.hashCode();
result = 31 * result + (expression != null ? expression.hashCode() : 0);
result = 31 * result + (statement != null ? statement.hashCode() : 0);
return result;
}
public List getMatchPatternInvolvedAliases() {
return expression.getMatchPatternInvolvedAliases();//TODO also check the statement...?
}
@Override
public void applyRemove(final ResultInternal result, final CommandContext context) {
if (expression != null) {
expression.applyRemove(result, context);
} else {
throw new CommandExecutionException("Cannot apply REMOVE " + this);
}
}
@Override
protected SimpleNode[] getCacheableElements() {
return new SimpleNode[] { expression, statement };
}
}
/* JavaCC - OriginalChecksum=4656e5faf4f54dc3fc45a06d8e375c35 (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy