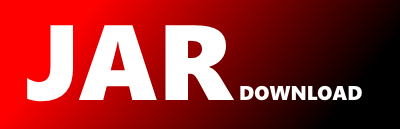
com.arcadedb.query.sql.parser.SimpleNode Maven / Gradle / Ivy
/*
* Copyright © 2021-present Arcade Data Ltd ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-FileCopyrightText: 2021-present Arcade Data Ltd ([email protected])
* SPDX-License-Identifier: Apache-2.0
*/
/* Generated By:JJTree: Do not edit this line. SimpleNode.java Version 4.3 */
/* JavaCCOptions:MULTI=true,NODE_USES_PARSER=false,VISITOR=true,TRACK_TOKENS=true,NODE_PREFIX=O,NODE_EXTENDS=,NODE_FACTORY=,SUPPORT_USERTYPE_VISIBILITY_PUBLIC=true */
package com.arcadedb.query.sql.parser;
import java.util.*;
public abstract class SimpleNode implements Node {
protected Node[] children;
protected Object value;
protected String cachedStringForm;
public SimpleNode() {
}
public SimpleNode(final int i) {
}
public void jjtOpen() {
// NO ACTIONS
}
public void jjtClose() {
// NO ACTIONS
}
public void jjtSetParent(final Node n) {
// parent = n;
}
public Node jjtGetParent() {
return null;//parent;
}
public void jjtAddChild(final Node n, final int i) {
if (children == null) {
children = new Node[i + 1];
} else if (i >= children.length) {
final Node[] c = new Node[i + 1];
System.arraycopy(children, 0, c, 0, children.length);
children = c;
}
children[i] = n;
}
public Node jjtGetChild(final int i) {
return children[i];
}
public int jjtGetNumChildren() {
return (children == null) ? 0 : children.length;
}
public void jjtSetValue(final Object value) {
this.value = value;
}
public Object jjtGetValue() {
return value;
}
public Token jjtGetFirstToken() {
//return firstToken;
return null;
}
public void jjtSetFirstToken(final Token token) {
//this.firstToken = token;
}
public Token jjtGetLastToken() {
//return lastToken;
return null;
}
public void jjtSetLastToken(final Token token) {
//this.lastToken = token;
}
/*
* You can override these two methods in subTypes of SimpleNode to customize the way the node appears when the tree is dumped.
* If your output uses more than one line you should override toString(String), otherwise overriding toString() is probably all
* you need to do.
*/
public String toString() {
if (cachedStringForm == null) {
final StringBuilder result = new StringBuilder();
toString(null, result);
cachedStringForm = result.toString();
}
return cachedStringForm;
}
public String toString(final String prefix) {
return prefix + this;
}
/*
* Override this method if you want to customize how the node dumps out its children.
*/
@Override
public boolean equals(final Object other) {
if (this == other)
return true;
if (other == null || getClass() != other.getClass())
return false;
final Object[] ownElements = getIdentityElements();
if (ownElements.length == 0)
// NOT IMPLEMENTED, USE THE DEFAULT IMPLEMENTATION
return super.equals(other);
final Object[] otherElements = ((SimpleNode) other).getIdentityElements();
for (int i = 0; i < ownElements.length; i++)
if (!Objects.equals(ownElements[i], otherElements[i]))
return false;
return true;
}
@Override
public int hashCode() {
final Object[] elements = getIdentityElements();
if (elements.length == 0)
// NOT IMPLEMENTED, USE THE DEFAULT IMPLEMENTATION
return super.hashCode();
return Objects.hashCode(elements);
}
protected Object[] getIdentityElements() {
return new Object[0];
}
protected SimpleNode[] getCacheableElements() {
return new SimpleNode[0];
}
public boolean isCacheable() {
for (SimpleNode e : getCacheableElements())
if (e != null && !e.isCacheable())
return false;
return true;
}
public boolean refersToParent() {
for (SimpleNode e : getCacheableElements())
if (e != null && e.refersToParent())
return true;
return false;
}
public void toString(final Map params, final StringBuilder builder) {
throw new UnsupportedOperationException("Not implemented in " + getClass().getSimpleName());
}
public Object getValue() {
return value;
}
public SimpleNode copy() {
throw new UnsupportedOperationException();
}
}
/* JavaCC - OriginalChecksum=d5ed710e8a3f29d574adbb1d37e08f3b (do not edit this line) */
© 2015 - 2024 Weber Informatics LLC | Privacy Policy